erlang相关书籍网盘分享下载:https://blog.csdn.net/weixin_40352578/article/details/82944159
1.将列表中的integer,float,atom转成字符串并合并成一个字符串:[1,a,4.9,"sdfds"] 结果:"1a4.9sdfds"(禁用++ -- app
end concat实现)
-export([to_list/1]).
to_binary(Term) when is_atom(Term)->
atom_to_binary(Term,utf8);
to_binary(Term) when is_integer(Term)->
integer_to_binary(Term);
to_binary(Term) when is_list(Term)->
list_to_binary(Term);
to_binary(Term) when is_float(Term)->
to_binary(float_to_list(Term,[{decimals, 1}])).
get_binary(List)->
get_binary(List,<<>>).
get_binary([],Ret)->Ret;
get_binary([H|T],Ret)->
Bin=to_binary(H),%将列表元素转换为二进制
get_binary(T,<<Ret/binary,Bin/binary>>).%拼接二进制数据
to_list(List)->
binary_to_list(get_binary(List)).%将列表转换为二进制数值,再转换为字符串
2.得到列表或元组中的指定位的元素 {a,g,c,e} 第1位a [a,g,c,e] 第1位a(禁用erlang lists API实现)
%% API
-export([get_index_element/2]).
for([H|T],Index,Cursor,Length) when Index<Length,Cursor<Length ->
if
Index=:=Cursor->
H;
true->
for(T,Index,Cursor+1,Length)
end;
for(_,_,_,_) ->
void.
list_length([],Length)->
Length;
list_length([_X|T],Length)->
list_length(T,Length+1).
get_index_element(L,I) when is_list(L)->
%%%Length=erlang:length(L),
%%%获取列表长度
Length = list_length(L,1),
%%%获取指定位置元素
for(L,I,1,Length);
get_index_element(L,Index) when is_tuple(L)->
get_index_element(tuple_to_list(L),Index).
3.根据偶数奇数过滤列表或元组中的元素(禁用API实现)
%% API
-export([get_element/2]).
%%%其中odd代表奇数,even代表偶数
get_element(L,T) when is_list(L)->
case T of
odd ->
[X||X <- L,(X rem 2)=:=1];
even ->
[X||X <- L,(X rem 2)=:=0]
end;
get_element(X,T) when is_tuple(X)->
list_to_tuple(get_element(tuple_to_list(X),T)).
4.便用匿名函数对列表奇数或偶数过淲
%% API
-export([get_element/2]).
get_element(L,T)->
Fun= fun(Odd)->
case Odd of
odd->
[X||X <- L,(X rem 2)=:=1];
even ->
[X||X <- L,(X rem 2)=:=0]
end
end,
Fun(T).
5.计算数字列表[1,2,3,4,5,6,7,8,9]索引N到M的和
%% API
-export([sum/3]).
index_sum([],_,_,_,S)->
S;
index_sum([H|T],Min,Max,Index,Sum) when is_integer(H),is_integer(Min),is_integer(Max)->
if
Index=<Max andalso Index>=Min ->
index_sum(T,Min,Max,Index+1,Sum+H);
Index>Max->
index_sum([],Min,Max,Index+1,Sum);
Index<Min->
index_sum(T,Min,Max,Index+1,Sum);
true->
false
end;
index_sum(_,_,_,_,_)->
'error params!'.
sum(L,Min,Max)->
if
Min>Max->
"error params";
Min>length(L)->
"params too large";
true->
index_sum(L,Min,Max,1,0)
end.
6.查询List1是否List2的前缀(禁用string API实现)
%% API
-export([check_pre/2]).
check_pre([],_) ->
true;
%%%使用变量的不可改变的性质
check_pre([H|L1],[H|L2]) ->
check_pre(L1,L2);
check_pre(_,_) ->
false.
7.逆转列表或元组(禁用lists API实现)
%% API
-export([reverse_operation/1]).
reverse_operation([],Result)->
Result;
reverse_operation([X|T],Result)->
reverse_operation(T,[X|Result]).
reverse_operation(L) when is_list(L)->
reverse_operation(L,[]);
reverse_operation(T) when is_tuple(T)->
list_to_tuple(reverse_operation(tuple_to_list(T))).
8.对列表进行排序
%% API
-export([list_sort/1]).
list_sort([])->
[];
list_sort([Pivot|T])->
list_sort([X||X<-T,X<Pivot])
++[Pivot]++
list_sort([X||X<-T,X>=Pivot]).
9.对数字列表进行求和再除以指定的参数,得到商余
%% API
-export([sum_to_div/2]).
list_sum([],Result)->
Result;
list_sum([X|T],Result) when is_integer(X)->
list_sum(T,Result+X);
list_sum(_,_)->
exit('error params') .
list_div(S,X)->
{S div X,S rem X}.
sum_to_div(L,X) when is_list(L) andalso is_integer(X)->
if
X>0 ->
list_div(list_sum(L,0),X);
true ->
"error params"
end;
sum_to_div(_,_)->
exit('error params') .
10.获得当前的堆栈
%% API
-export([get_stack/0]).
get_stack()->
try
throw('test stack!')
catch
_:_ ->io:format("stack info:~p~n",erlang:get_stacktrace())
end.
11.获得列表或元组中的最大最小值(禁用API实现)
%% API
-export([get_max/1,get_min/1]).
max_value([],Result)->
Result;
max_value([X|T],Result)->
if
X>=Result->
max_value(T,X);
true->
max_value(T,Result)
end.
get_max(T) when is_tuple(T)->
max_value(tuple_to_list(T),0);
get_max(L) when is_list(L)->
max_value(L,0).
12.查找元素在元组或列表中的位置
%% API
-export([get_element_index/2]).
element_value([],_,_,Result)->
Result;
element_value([X|T],Index,Value,Result)->
if
X=:=Value->
element_value(T,Index+1,Value,Result++[Index]);
true->
element_value(T,Index+1,Value,Result)
end.
get_element_index(T,Value) when is_tuple(T)->
get_element_index(tuple_to_list(T),Value);
get_element_index(L,Value) when is_list(L)->
element_value(L,1,Value,[]).
13.判断A列表([3,5,7,3])是否在B列表([8,3,5,3,5,7,3,9,3,5,6,3])中出现,出现则输出在B列表第几位开始
%% API
-export([get_sub_index/2]).
check_pre([],_) ->
true;
check_pre([H|L1],[H|L2]) ->
check_pre(L1,L2);
check_pre(_,_) ->
false.
for([],_,N,_)->
N;
for([A|T1],[B|T2],N,Term) when N=<Term->
if
A=:=B ->
%%%检测列表A是不是列表B的子列表
case check_pre(T1,T2) of
true->N;
_->for([A|T1],T2,N+1,Term)
end;
true ->
for([A|T1],T2,N+1,Term)
end;
for(_,_,_,_)->
"A is not B's substring".
get_sub_index(A,B) when is_list(A) andalso is_list(B)->
for(A,B,1,length(B));
get_sub_index(_,_)->
"error params".
14.{8,5,2,9,6,4,3,7,1} 将此元组中的奇数进行求和后除3的商(得值A),并将偶数求和后乘3(得值B),然后求A+B结果
%% API
-export([get_calculate/1]).
get_element(L,T) when is_list(L)->
if
T=:=odd->
[X||X <- L,(X rem 2)=:=1];
T=:=even->
[X||X <- L,(X rem 2)=:=0];
true->"error params"
end.
get_calculate(X) when is_tuple(X)->
A=lists:sum(get_element(tuple_to_list(X),odd))/3,
B=lists:sum(get_element(tuple_to_list(X),even))*3,
trunc(A+B) .
15.在shell中将unicode码显示为中文
%% API
-export([test/0]).
test()->
io:format("~ts~n",["中国"]).
16.传入列表L1=[K|_]、L2=[V|_]、L3=[{K,V}|_],L1和L2一一对应,L1为键列表,L2为值列表,L3存在重复键,把L3对应键的值加到L2
%% API
-export([get_duplicate_data/3]).
%%%nth(N, List) -> Elem
%%%获取列表元素的下标
find_list_index([],_,_)->
false;
find_list_index([H|L],Element,Index)->
if
H=:=Element ->
Index;
true ->
find_list_index(L,Element,Index+1)
end.
find_list_index(L,Element)->
find_list_index(L,Element,1).
%%%替换列表中指定索引的元素
replace_list_element([],_,_,_,Result)->
Result;
replace_list_element([H|L],Index,Cursor,Element, Result)->
if
Index=:=Cursor->
replace_list_element(L,Index,Cursor+1,Element,[Element|Result]);
true ->
replace_list_element(L,Index,Cursor+1,Element,[H|Result])
end.
replace_list_element(L,Index,Element)->
replace_list_element(L,Index,1,Element,[]).
get_duplicate_data(_,L2,[])->
L2;
get_duplicate_data(L1,L2,[H3|L3])->
%%%获取L3中元素key在L1中的位置
case find_list_index(L1,element(1,H3) ) of
Index->
%%%将L3中对应的值累加到L2中
Sum = element(2,H3) +lists:nth(Index,L2),
get_duplicate_data(L1,replace_list_element(L2,Index,Sum),L3);
_->get_duplicate_data(L1,L2,L3)
end.
17.删除或查询元组中第N个位置的值等于Key的tuple(禁用lists API实现)
%% API
-export([remove_duple/3]).
delete_duple([],_,_,_,Result)->
Result;
%%%N:第N个变量,Key:游标
delete_duple([X|T],N,Key,Index,Result)->
if
%%%找出第N个变量元素为等于key的tuple
Key=:=element(1,X) andalso N=:=Index->
delete_duple(T,N,Key,Index+1,Result);
true ->
if
%%%判断变量元素是否为key
Key=:=element(1,X) ->
delete_duple(T,N,Key,Index+1,Result++[X]);
true ->
delete_duple(T,N,Key,Index,Result++[X])
end
end.
remove_duple(L,N,Key) when is_tuple(L)->
list_to_tuple(delete_duple(tuple_to_list(L) ,N,Key,1,[])).
18.对一个字符串按指定符字劈分(禁用string API实现)
%% API
-export([string_split/2]).
list_reverse([],Acc)->Acc;
list_reverse([H|T],Acc)->
list_reverse(T,[H|Acc]).
split_operation([],_,[],Result)->Result;
split_operation([],_,Tmp,Result)->[Tmp|Result];
split_operation([X|T],[C|[]],Tmp,Result)->
%%io:format("~p:~p:~p:~p~n",[X,T,C,Result]),
case X of
C-> %%匹配字符成功,开始切割字符串
case Tmp of
[]->split_operation(T,[C],[],Result);
_->split_operation(T,[C],[],[Tmp|Result])
end;
_->split_operation(T,[C],[X|Tmp],Result)
end.
string_split(L,C)->
split_operation(list_reverse(L,[]),C,[],[]).
19.合并多个列表或元组
%% API
-export([merge_operation/1]).
%%%也可以使用list的api concat append
list_merge([],Result)->Result;
list_merge([H|T],Result) when is_tuple(H)->
list_merge(T,Result++tuple_to_list(H));
list_merge([H|T],Result) when is_list(H)->
list_merge(T,Result++H).
merge_operation(L) when is_list(L)->
list_merge(L,[]);
merge_operation(_L)->
'error params'.
20.{5,1,8,7,3,9,2,6,4} 将偶数乘以它在此元组中的偶数位数, 比如,8所在此元组中的偶数位数为1,2所在此元组中的偶数位数为2
%% API
-export([even_times/1]).
convert_to_times([],_,R)->R;
%%%Index代表偶数索引
convert_to_times([H|T],Index,Result) when is_list([H|T]), is_integer(H)->
if
H rem 2=:=0->
convert_to_times(T,Index+1,[H*Index|Result]);
true ->
convert_to_times(T,Index,[H|Result])
end.
even_times(L) when is_tuple(L)->
%%%将元组转换为列表进行操作
list_to_tuple(lists:reverse(convert_to_times(tuple_to_list(L),1,[])) );
even_times(L) when is_list(L)->
lists:reverse(convert_to_times(L,1,[]));
even_times(_)->
'error params'.
21.排序[{"a",5},{"b",1},{"c",8},{"d",7},{"e",3},{"f",9},{"g",2},{"h",6},{"i",4}], 以元组的值进行降序 优先用API
%% API
-export([list_sort/1]).
%%%使用匿名函数实现
%list_sort(L)->
% Fun = fun(A,B)->
% element(2,A)>element(2,B)
% end,
% lists:sort(Fun,L).
%%%API
list_sort(T)->
lists:reverse(lists:keysort(2,T)) .
22.{8,5,2,9,6,4,3,7,1} 将此元组中的奇数进行求和
%% API
-export([sum_odd/1]).
acc_odd([],Result)->Result;
acc_odd([H|T],Result) when is_integer(H)->
if
H rem 2=:=1 ->
acc_odd(T,Result+H);
true ->
acc_odd(T,Result)
end.
sum_odd(L) when is_tuple(L)->
acc_odd(tuple_to_list(L),0).
23.%%%传入任意I1、I2、D、Tuple四个参数,检查元组Tuple在索引I1、I2位置的值V1、V2,如果V1等于V2则删除V1,把D插入V2前面,返回新元组,
%%%如果V1不等于V2则把V2替换为V1,返回新元组
%%%注意不能报异常,不能用try,不满足条件的,返回字符串提示
%% API
-export([get_new_tuple/4]).
%%%替换列表中元素
list_exchange(L,I1,I2)->
setelement(I2,L,element(I1,L)).
%%%删除指定索引元素,返回新列表
list_delete([],_,_,Result)->Result;
list_delete([X|T],I1,I,Result)->
if
I1=:=I ->
list_delete(T,I1,I+1,Result);
true ->
list_delete(T,I1,I+1,[X|Result])
end.
%%%指定位置插入元素,返回新列表
list_insert([],_,_,_,Result)->
Result;
list_insert([X|T],I2,I,D,Result)->
if
I2=:=I ->
list_insert(T,I2,I+1,D,[X,D|Result]);
true ->
list_insert(T,I2,I+1,D,[X|Result])
end.
do_check(T,I1,I2)->
{ok,element(I1,T),element(I2,T)}.
get_new_tuple(I1,I2,D,T) when is_tuple(T)->
case catch do_check(T,I1,I2) of
{ok,V1,V2}->
if
%%%如果V1等于V2则删除V1,把D插入V2前面,返回新元组
V1=:=V2 ->
L=list_delete(tuple_to_list(T),I1,1,[]),
io:format("L:~p~p~n",[lists:reverse(L),D]),
lists:reverse(list_insert(lists:reverse(L),I2-1,1,D,[])) ;
true ->
%%%如果V1不等于V2则把V2替换为V1,返回新元组
list_exchange(T,I1,I2)
end;
{_,_}->
{"error params","cross the border!"}
end;
get_new_tuple(_,_,_,_)->
{"error params","please input tuple!"}.
24.删除列表或元组中全部符合指定键的元素
%% API
-export([remove_key_value/2]).
remove_key([],_,Result)->
lists:reverse(Result) ;
remove_key([H|T],Key,Result)->
if
Key=:=element(1,H) ->
remove_key(T,Key,Result);
true ->
remove_key(T,Key,[H|Result])
end.
remove_key_value(L,Key) when is_tuple(L)->
list_to_tuple(remove_key_value(tuple_to_list(L),Key));
remove_key_value(L,Key) when is_list(L)->
remove_key(L,Key,[]).
25.替换列表或元组中第一个符合指定键的元素
%% API
-export([list_replace/3]).
%%%可以使用lists:keyreplace()实现
replace_operation([],_,_,_,Result)->
lists:reverse(Result);
%%%Index是游标
replace_operation([H|T],Index,Key,Value,Result)->
if
Key=:=element(1,H)andalso Index=:=1 ->
replace_operation(T,Index+1,Key,Value,[{Key,Value}|Result]);
true ->
replace_operation(T,Index,Key,Value,[H|Result])
end.
list_replace(L,Key,Value) when is_tuple(L)->
list_replace(tuple_to_list(L),Key,Value);
list_replace(L,Key,Value) when is_list(L)->
replace_operation(L,1,Key,Value,[]).
26.将指定的元素插入到列表中指定的位置,列表中后面的元素依次向后挪动
%% API
-export([insert_value/3]).
insert_operation([],_,_,_,Result)->
lists:reverse(Result) ;
%%%I代表插入位置,I1是游标,Value是插入值
insert_operation([H|T],I,Value,I1,Result)->
if
I=:=I1 ->
insert_operation(T,I,Value,I1+1,[H,Value|Result]);
true ->
insert_operation(T,I,Value,I1+1,[H|Result])
end.
insert_value(L,Index,Value) when is_list(L)->
insert_operation(L,Index,Value,1,[]).
27.对[6,4,5,7,1,8,2,3,9]进行排序(降序--冒泡排序)或API排序
%% API
-export([list_sort/1]).
%%API排序
list_sort(L)->
lists:sort(fun(_,_)->false end,L).
%%冒泡排序
28.字符串"goods,143:6|money:15|rmb:100|money:100|goods,142:5"解析成[{money,115},{rmb,100},{goods,[{143,6},{142,5}]}]可以代码写定KEY进行匹配归类处理
%% API
-export([string_split_func/1]).
%%%将字符串按照符号分割为列表
-import(test18,[string_split/2]).
-define(NTh(X,Y),lists:nth(X,Y)).
filter_money(S)->
{money,list_to_integer(?NTh(2,string_split(S,":"))) }.
filter_rmb(S)->
{rmb,list_to_integer(?NTh(2,string_split(S,":"))) }.
filter_goods(S)->
{goods,{list_to_integer(?NTh(1,string_split(?NTh(2,string_split(S,",")),":"))) ,list_to_integer(?NTh(2,string_split(?NTh(2,string_split(S,",")),":")))}}.
filter_string([],Result)->
Result;
filter_string([H|T],Result)->
case catch {string:str(H,"money"),string:str(H,"rmb"),string:str(H,"goods")} of
{_,0,0}->
filter_string(T,[filter_money(H)|Result]);
{0,_,0} ->
filter_string(T,[filter_rmb(H)|Result]);
{0,0,_}->
filter_string(T,[filter_goods(H)|Result]);
_->"illegal params"
end.
acc_rmb([],Result)->
Result;
acc_rmb([X|T],Result)->
if
element(1,X)=:=rmb ->
case Result of
[]->acc_rmb(T,[X]);
_->acc_rmb(T,[{rmb,element(2,X)+element(2,Result)}])
end;
true ->
acc_rmb(T,Result)
end.
acc_money([],Result)->
Result;
acc_money([X|T],Result)->
if
element(1,X)=:=money ->
case Result of
[]->acc_money(T,[X]);
_->acc_money(T,[{money,element(2,X)+element(2,?NTh(1,Result))}])
end;
true ->
acc_money(T,Result)
end.
acc_goods([],Result)->
Result;
acc_goods([X|T],Result)->
if
element(1,X)=:=goods ->
case Result of
[]->acc_goods(T,[X]);
_->io:format("goods~p~p~p~n",[x,Result,element(2,?NTh(1,Result))]),
acc_goods(T,[{goods,[element(2,X),element(2,?NTh(1,Result))]}])
end;
true ->
acc_goods(T,Result)
end.
get_final_string(L)->
acc_rmb(L,[])++acc_money(L,[])++acc_goods(L,[]).
string_split_func(L)->
%%%将字符串按照"|"分割成字符串元素
R=string_split(L,"|"),
io:format("R:~p~n",[R]),
%%%将字符串元素整理为元组
S=filter_string(R,[]),
io:format("S:~p~n",[S]),
%%%对列表进行归类获取组中结果
get_final_string(S).
29.移除元组或列表中指定位置的元素
%% API
-export([remove_element/2]).
remove_operation([],_,_,Result)->
lists:reverse(Result) ;
%%%I为删除元素位置,I1为游标
remove_operation([H|T],I,I1,Result)->
if
I=:=I1 ->
remove_operation(T,I,I1+1,Result);
true ->
remove_operation(T,I,I1+1,[H|Result])
end.
remove_element(L,I) when is_tuple(L)->
list_to_tuple(remove_element(tuple_to_list(L),I));
remove_element(L,I) when is_list(L)->
remove_operation(L,I,1,[]);
remove_element(_,_)->
'error params'.
30.指定列表第几位之后的数据进行反转。如:指定[2,3,5,6,7,2]第3位后进行反转(谢绝一切API(lists:concat,lists:reverse等)、++、--方法)
%% API
-export([list_reverse_tail/2]).
%%列表反转
reverse_operation([],Result)->
Result;
reverse_operation([H|T],Result)->
reverse_operation(T,[H|Result]).
list_reverse(L)->
reverse_operation(L,[]).
%列表拼接
concat_operation([],L)->
L;
concat_operation([L1|T],L2)->
concat_operation(T,[L1|L2]).
list_concat(L1,L2)->
concat_operation(list_reverse(L1),L2).
reverse_tail([],_,_,Prefix,Tail)->
{list_reverse(Prefix),Tail};
reverse_tail([H|T],I,N,Prefix,Tail)->
if
I>N ->
reverse_tail(T,I+1,N,Prefix,[H|Tail]);
true ->
reverse_tail(T,I+1,N,[H|Prefix],Tail)
end.
list_reverse_tail(L,N)->
%%%分别获取前缀和后缀 可以使用,前缀sublist 后缀nthtail
case catch reverse_tail(L,1,N,[],[]) of
{Prefix,Tail}->list_concat(Prefix,Tail);
_->"unknown error"
end.
31.使用“匿名函数”检查列表的每个元素是否符合传入的最大最小值(都是闭区间)
%% API
-export([check_element/3]).
check_element([],_,_)->
"all element ok!";
check_element([H|T],Max,Min)->
if
H>=Min andalso H=<Max ->
check_element(T,Max,Min);
true ->
"illegal element exits "
end.
32.获得列表或元组的最大最小值
%% API
-export([list_value/1]).
list_max([],Max)->
Max;
list_max([H|T],Max)->
if
H>Max ->
list_max(T,H);
true ->
list_max(T,Max)
end.
list_min([],Min)->
Min;
list_min([H|T],Min)->
if
H<Min ->
list_min(T,H);
true ->
list_min(T,Min)
end.
list_value(L) when is_tuple(L)->
list_value(tuple_to_list(L));
list_value(L) when is_list(L)->
{list_max(L,hd(L)),list_min(L,hd(L))}.
33.生成指定数量元组,每个元素为随机integer元素, 元素不能有相同的
%% API
-export([generate_tuple/1]).
generate_tuple(N)->
list_to_tuple([X||X<-lists:seq(1,N)]).
希望大家提建议,感谢!!!
扫描二维码关注公众号,回复:
3682039 查看本文章
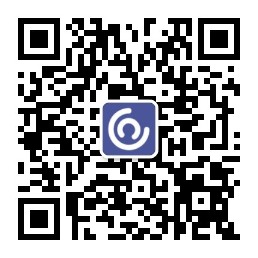