webapck.base.config.js
/**
* Created by msw on 2018/4/19.
*/
const path = require('path');
const webpack = require('webpack');
const HtmlWebpackPlugin = require('html-webpack-plugin');
const ExtractTextPlugin = require('extract-text-webpack-plugin');
const fs = require('fs');
const babelrc = JSON.parse(fs.readFileSync('./.babelrc'));
require('babel-register')(babelrc);
const config = require('./config/config');
//判断当前运行环境是开发模式还是生产模式
const nodeEnv = process.env.NODE_ENV || 'development'; //获取package.json文件中script设置的NODE_ENV环境
var isPro = nodeEnv === 'production ';
console.log('当前运行环境:', isPro ? 'production' : 'development');
var rootPath = "./src";
var resolve = function (dir) {
return path.join(__dirname, dir);
};
//设置插件
var plugins = [
//使用插件进行抽取样式
new ExtractTextPlugin('css/[name].css')
];
var array = config.htmlPlugin(rootPath);
array.forEach(function(v){
var str = v.replace(rootPath+'/',"");
console.log(str);
plugins.push(
new HtmlWebpackPlugin({
filename: v.replace(rootPath+"/",""),
template: v,
chunks:[str.replace(".html","")],
hash: true, // hash选项的作用是 给生成的 js 文件一个独特的 hash 值,该 hash 值是该次 webpack 编译的 hash 值。默认值为 false
showErrors: true, // showErrors 的作用是,如果 webpack 编译出现错误,webpack会将错误信息包裹在一个 pre 标签内,属性的默认值为 true
inject: true, // 注入选项 有四个值 ture: 默认值,script标签位于html文件的 body 底部, body: 同 true, head: script标签位于html文件的 head 底部,false:不注入script标签
minify:{
removeComments:true //是否压缩时 去除注释
},
cache: true // 默认值是 true。表示只有在内容变化时才生成一个新的文件
})
)
})
//根据环境设置使用的插件
if (isPro) {
//内置插件,这里面的标识就相当于全局变量,直接使用配置的标识。
new webpack.DefinePlugin({
'process.env':{
'NODE_ENV': JSON.stringify(nodeEnv)
}
}),
//去压缩你的JavaScript代码
plugins.push(
new webpack.optimize.UglifyJsPlugin({
compress: {
warnings: false
}
})
)
} else {
//内置插件,这里面的标识就相当于全局变量,直接使用配置的标识。
new webpack.DefinePlugin({
'process.env':{
'NODE_ENV': JSON.stringify(nodeEnv)
}
}),
plugins.push(
//模块热替换
new webpack.HotModuleReplacementPlugin()
)
}
baseConfigs = {
devtool: !isPro && 'cheap-module-eval-source-map', //webpack sourcemap模式map
entry: config.entry(rootPath),
//entry: {
//app: ['./src/main']
//},
output: {
filename: '[name].js',
path: path.resolve(__dirname, 'static'),
////当生成的资源文件和站点不在同一地方时需要配置改地址 e.g.:站点在src,资源生成到/src/static/dist,那么publicPath="/static/dist"
//publicPath: isPro ? './' : './',
chunkFilename: '[name].[hash].js'
},
plugins: plugins,
node: {
fs: 'empty'
},
resolve: {
extensions: ['.js', '.vue', '.less', '.css'], //自动解析确定的扩展。默认值为:
modules: [ //告诉 webpack 解析模块时应该搜索的目录。此时的配置是node_modules目录优先于./src目录
path.resolve(__dirname, 'node_modules'),
path.join(__dirname, './src')
],
alias: {
'vue': 'vue/dist/vue.js' //代理引入名称,在require("components")相当于require("src/components")
}
},
module: {
rules: [{
test: /\.js$/, //正则表达式,用于匹配到的文件
use: ['babel-loader'], //字符串或者数组,处理匹配到的文件,数组模式
exclude: /node_modules/, //exclude 表示哪些目录中的 .js 文件不要进行 babel-loader
include: resolve('/') //include 表示哪些目录中的 .js 文件需要进行 babel-loader
},{
test: /\.vue$/,
use: ['vue-loader'],
exclude: /node_modules/,
include: resolve('src')
}, {
test: /\.(png|jpe?g|gif|svg)(\?.*)?$/,
use: ['url-loader?limit=8192&name=images/[hash:16].[ext]']
}, {
test: /\.(less|css)$/,
use: ExtractTextPlugin.extract({
use: ['css-loader', 'less-loader'],
fallback: 'style-loader'
})
}]
},
devServer: {
contentBase: resolve('/'), //告诉服务器从哪里提供内容,默认情况下,将使用当前工作目录作为提供内容的目录,但是你可以修改为其他目录
historyApiFallback: true, //当使用HTML5 History API,任意的 404 响应可以提供为 index.html 页面。通过传入以下启用
compress: true, //一切服务都启用gzip 压缩
port: 8088, //指定要监听请求的端口号:
//host: "127.0.0.1", //指定使用一个 host
hot: true, //启用 webpack 的模块热替换特性:
inline: true, //在 dev-server 的两种不同模式之间切换。默认情况下,应用程序启用内联模式(inline mode)。这意味着一段处理实时重载的脚本被插入到你的包(bundle)中,并且构建消息将会出现在浏览器控制台。
stats: {
// 增加资源信息
assets: true,
// 增加子级的信息
children: false,
// 增加内置的模块信息
modules: false,
// 增加包信息(设置为 `false` 能允许较少的冗长输出)
chunks: false,
// 增加 publicPath 的信息
publicPath: false,
// 增加时间信息
timings: true,
// 增加提示
warnings: true,
// `webpack --colors` 等同于
colors: {
green: '\u001b[32m',
}
},
}
};
exports.baseConfig = baseConfigs;
webpack.config.js
入口文件
/**
* Created by msw on 2018/4/19.
*/
const baseConfig = require('./webpack.base.config');
module.exports = baseConfig.baseConfig;
package.json
{
"name": "vue2-web",
"version": "1.0.0",
"description": "vue2、webpack2框架",
"main": "index.js",
"scripts": {
"start": "set NODE_ENV=development && webpack-dev-server",
"build": "set NODE_ENV=production && webpack -p --progress"
},
"author": "hyy",
"license": "ISC",
"devDependencies": {
"babel-core": "^6.24.1",
"babel-helper-vue-jsx-merge-props": "^2.0.2",
"babel-loader": "^6.4.1",
"babel-plugin-syntax-jsx": "^6.18.0",
"babel-plugin-transform-runtime": "^6.23.0",
"babel-plugin-transform-vue-jsx": "^3.4.2",
"babel-polyfill": "^6.23.0",
"babel-preset-es2015": "^6.24.1",
"babel-preset-flow-vue": "^1.0.0",
"babel-preset-stage-0": "^6.24.1",
"babel-preset-stage-2": "^6.24.1",
"css-loader": "^0.28.0",
"extract-text-webpack-plugin": "^2.1.0",
"file-loader": "^0.11.1",
"html-webpack-plugin": "^2.28.0",
"jquery": "^3.3.1",
"less": "^2.7.2",
"less-loader": "^4.0.3",
"style-loader": "^0.16.1",
"url-loader": "^0.5.8",
"vue-loader": "^11.3.4",
"vue-template-compiler": "^2.2.6",
"webpack": "^2.5.1",
"webpack-dev-server": "^2.5.2"
},
"dependencies": {
"swiper": "^3.4.2",
"vue": "^2.2.6",
"vue-resource": "^1.3.1",
"vue-router": "^2.4.0"
}
}
.babelrc
{
"presets": ["es2015", "flow-vue", "stage-0", "stage-2"],
"plugins": ["transform-vue-jsx"],
"comments": false,
"env": {
"production": {
"plugins": [
["transform-runtime", { "polyfill": false, "regenerator": false }]
]
}
}
}
config.js
在这一个配置文件中主要是通过遍历获取文件夹下的所有文件及路径
/**
* Create By MOSHUNWEI At 2018-07-05
* 入口文件,通过遍历获取所有入口js,入后输出到相应的目录
*/
const fs = require('fs');
//遍历获取所有js节点
var getEntries = function(url,map,rootPath) {
var files = [];
//判断给定的路径是否存在
if( fs.existsSync(url) ) {
//返回文件和子目录的数组
files = fs.readdirSync(url);
//console.log(files);
files.forEach(function(file,index){
var curPath = url + "/" + file;
// var curPath = path.join(url,file);
//fs.statSync同步读取文件夹文件,如果是文件夹,在重复触发函数
if(fs.statSync(curPath).isDirectory()) { // recurse
getEntries(curPath,map,rootPath);
// 是文件delete file
} else {
if(curPath.match(".*\.js$")==null?false:true){
var val = curPath.replace("\.js","").replace(rootPath+"/",'');
map[val]=val;
};
}
});
}else{
console.log("给定的路径不存在,请给出正确的路径");
}
};
function entries(path){
var map = {};
getEntries(path,map,path);
return map;
};
var getHtml = function(url,plugins) {
var files = [];
//判断给定的路径是否存在
if( fs.existsSync(url) ) {
//返回文件和子目录的数组
files = fs.readdirSync(url);
files.forEach(function(file,index){
var curPath = url + "/" + file;
// var curPath = path.join(url,file);
//fs.statSync同步读取文件夹文件,如果是文件夹,在重复触发函数
if(fs.statSync(curPath).isDirectory()) { // recurse
getHtml(curPath,plugins);
// 是文件delete file
} else {
if(curPath.match(".*\.html$")==null?false:true){
plugins.push(curPath);
};
}
});
}else{
console.log("给定的路径不存在,请给出正确的路径");
}
};
function htmlPlugins(path){
var plugins = [];
getHtml(path,plugins);
return plugins;
};
module.exports = {
entry:entries,
htmlPlugin:htmlPlugins
}
整个webpack项目的结构
就是通过webapck打包后把src目录下的js,vue,css,html都全部一起打包到static目录之下。
扫描二维码关注公众号,回复:
4000023 查看本文章
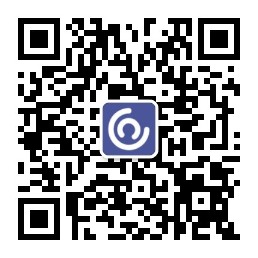
运行后的截图