静态类成员函数
可以将成员函数声明为静态的(函数声明必须包含关键字static, 但是如果函数定义时独立的, 则其中不能包含 关键字static).
静态类成员函数有如下特点:
1.不能通过对象调用静态成员函数.
2.如果静态成员函数是在共有部分声明的, 则可以使用类名和作用域解析运算符来调用它.
3.静态成员函数不与特定的对象关联, 因此只能使用静态数据成员.
例如给String类添加一个名为HomeMany()的静态成员函数, 方法是在类声明中添加如下原型/定义:
static int HomMany(){return num_strings;}
调用它的方式如下:
int count = String::HomMany();
看一个例子, 重写了之前自己定义的String类
// string1.h
#ifndef STRING1_H_
#define STRING1_H_
#include <iostream>
using std::ostream;
using std::istream;
class String
{
private:
char * str;
int len;
static int num_strings;
// cin输入限制
static const int CINLIM = 80;
public:
// 构造函数
String(const char * s);
// 默认构造函数
String();
// 复制构造函数
String(const String &);
// 析构函数
~String();
// 定义内带实现, 就是内联函数
int length() const {return len;}
// 重载操作运算符
String & operator=(const String &);
String & operator=(const char *);
char & operator[](int i);
const char & operator[](int i) const;
// 友元函数, 重载操作运算符
friend bool operator<(const String &st, const String &st2);
friend bool operator>(const String &st, const String &st2);
friend bool operator==(const String &st, const String &st2);
friend ostream & operator<<(ostream & os, const String &st);
friend istream & operator>>(istream & is, String &st);
// 静态方法
static int HowMany();
};
#endif
第二个文件:
// 第二个文件:
// string1.cpp
#include <cstring>
#include "string1.h"
using std::cin;
using std::cout;
// 初始化静态成员
int String::num_strings = 0;
// 静态方法:
int String::HowMany()
{
return num_strings;
}
// 类方法
// 构造函数
String::String(const char * s)
{
len = std::strlen(s);
str = new char[len + 1];
std::strcpy(str, s);
num_strings++;
}
// 默认构造函数
String::String()
{
len = 4;
str = new char[1];
str[0] = '\0';
num_strings++;
}
// 构造函数
String::String(const String & st)
{
num_strings++;
len = st.len;
str = new char[len + 1];
std::strcpy(str, st.str);
}
// 析构函数
String::~String()
{
--num_strings;
delete[] str;
}
// 重载操作运算符
// 将一个String对象赋值给一个String
String & String::operator=(const String & st)
{
if(this == &st)
return *this;
delete[] str;
len = st.len;
str = new char[len + 1];
std::strcpy(str, st.str);
return *this;
}
// 将一个char型指针, 赋值给一个String对象
String & String::operator=(const char * s)
{
delete[] str;
len = std::strlen(s);
str = new char[len + 1];
std::strcpy(str, s);
return *this;
}
char & String::operator[](int i)
{
return str[i];
}
const char & String::operator[](int i) const
{
return str[i];
}
// 友元函数
bool operator<(const String &st1, const String &st2)
{
return (std::strcmp(st1.str, st2.str) < 0);
}
bool operator>(const String &st1, const String &st2)
{
return st2 < st1;
}
bool operator==(const String &st1, const String &st2)
{
return (std::strcmp(st1.str, st2.str) == 0);
}
ostream & operator<<(ostream & os, const String & st)
{
os << st.str;
return os;
}
istream & operator>>(istream & is, String & st)
{
char temp[String::CINLIM];
is.get(temp, String::CINLIM);
if(is)
st = temp;
while(is && is.get() != '\n')
continue;
return is;
}
第三个文件:
// 第三个文件
// usestring1.cpp
// compile with string1.cpp
#include <iostream>
#include "string1.h"
const int ArSize = 10;
const int MaxLen = 81;
int main()
{
using std::cout;
using std::cin;
using std::endl;
String name;
cout << "Hi, what's your name?" << endl;
cin >> name;
cout << name << ", please enter up-to " << ArSize << " short sayings <empty line to quit>" << endl;
String sayings[ArSize];
char temp[MaxLen];
int i;
for(i = 0; i < ArSize; i++)
{
cout << i + 1 << ": ";
cin.get(temp, MaxLen);
while(cin && cin.get() != '\n')
continue;
if(!cin || temp[0] == '\0')
break;
else
sayings[i] = temp;
}
int total = i;
if(total > 0)
{
cout << "Here are your sayings: " << endl;
for(i = 0; i < total; i++)
cout << sayings[i][0] << ": " << sayings[i] << endl;
int shortest = 0;
int first = 0;
for(i = 1; i < total; i++)
{
if(sayings[i].length() < sayings[shortest].length())
shortest = i;
if(sayings[i] < sayings[first])
first = i;
}
cout << "Shortest saying: " << endl;
cout << sayings[shortest] << endl;
cout << "First alaphabetically: " << endl;
cout << sayings[first] << endl;
cout << "This program used " << String::HowMany() << " String objects. Bye." << endl;
} else {
cout << "No input! Bye." << endl;
}
return 0;
}
程序运行结果为:
扫描二维码关注公众号,回复:
4524969 查看本文章
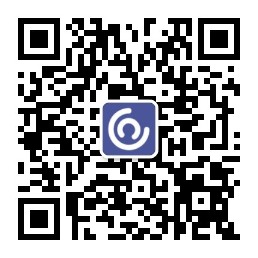