一、前端检查 案例
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title> 密码强度检测 </title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <style type="text/css"> input{float: left; font-size: 14px; width: 250px; height: 25px; border: 1px solid #ccc; padding-left: 10px;} #tips{float: left; font-size: 12px; width: 400px; height: 25px; margin: 4px 0 0 20px;} #tips span{float: left; width: 40px; height: 20px; color: #fff; overflow:hidden; margin-right: 10px; background: #D7D9DD; line-height:20px; text-align: center; } #tips .s1{background: #F45A68;}/*红色*/ #tips .s2{background: #fc0;}/*橙色*/ #tips .s3{background: #cc0;}/*黄色*/ #tips .s4{background: #14B12F;}/*绿色*/ </style> </head> <body> <input type="text" id="password" value="" maxlength="16" /> <div id="tips"> <span>弱</span> <span>中</span> <span>强</span> <span>很强</span> </div> </body> <script type="text/javascript"> var password = document.getElementById("password"); //获取文本框的对象 //var value = password.value; //获取用户在文本框里面填写的值 //获取所有的span标签 返回值是一个数组 var spanDoms = document.getElementsByTagName("span"); password.onkeyup = function(){ //获取用户输入的密码,然后判断其强度 返回0 或者 1 2 3 4 var index = checkPassWord(this.value); for(var i = 0; i <spanDoms.length; i++){ spanDoms[i].className = "";//清空span标签所有的class样式 if(index){//0 代表false 其余代表true spanDoms[index-1].className = "s" + index ; } } } //校验密码强度 function checkPassWord(value){ // 0: 表示第一个级别 1:表示第二个级别 2:表示第三个级别 // 3: 表示第四个级别 4:表示第五个级别 var modes = 0; if(value.length < 6){//最初级别 return modes; } if(/\d/.test(value)){//如果用户输入的密码 包含了数字 modes++; } if(/[a-z]/.test(value)){//如果用户输入的密码 包含了小写的a到z modes++; } if(/[A-Z]/.test(value)){//如果用户输入的密码 包含了大写的A到Z modes++; } if(/\W/.test(value)){//如果是非数字 字母 下划线 modes++; } switch(modes){ case 1 : return 1; break; case 2 : return 2; break; case 3 : return 3; break; case 4 : return 4; break; } } </script> </html>
二、后端检查案例
https://blog.csdn.net/u010156024/article/details/45673581
三、vue专项检查案例
http://www.datouwang.com/jiaoben/973.html
主要代码:
/* DaTouWang URL: www.datouwang.com */ var app = new Vue({ el: "#app", data: { password: null, password_length: 0, typed: false, contains_lovercase: false, contains_number: false, contains_uppercase: false, valid_password_length: false, valid_password: false }, methods: { p_len: function() { this.password_length = this.password.length; if (this.password_length > 7) { this.valid_password_length = true; } else { this.valid_password_length = false; } if (this.password_length > 0) { this.typed = true; } else { this.typed = false; } this.contains_lovercase = /[a-z]/.test(this.password); this.contains_number = /\d/.test(this.password); this.contains_uppercase = /[A-Z]/.test(this.password); // Check if the password is valid if (this.contains_lovercase == true && this.contains_number == true) { this.valid_password = false; if ( this.contains_uppercase == true && this.valid_password_length == true ) this.valid_password = true; } else { this.valid_password = false; } } } });
四、vue实际应用:
扫描二维码关注公众号,回复:
4633540 查看本文章
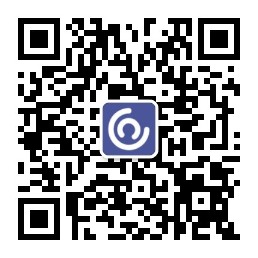
<style lang="css" scoped> #tips{float: left; font-size: 12px; width: 400px; height: 25px; margin: 4px 0 0 20px;} #tips span{float: left; width: 40px; height: 20px; color: #fff; overflow:hidden; margin-right: 10px; background: #D7D9DD; line-height:20px; text-align: center; } #tips .s1{background: #F45A68;}/*红色*/ #tips .s2{background: #fc0;}/*橙色*/ #tips .s3{background: #cc0;}/*黄色*/ #tips .s4{background: #14B12F;}/*绿色*/ </style> <template> <div> <!-- <sticky class-name="sub-navbar white"> <el-button style="margin:5px 10px;float:left" type="text" icon="el-icon-arrow-left" @click="handleReturn" >返回</el-button> </sticky> --> <div style="padding:20px 20px 20px 20px;width:500px;"> <div> <el-form ref="dataForm" :rules="rules" :model="temp" label-position="right" label-width="120px" size="small" > <el-form-item label="用户名:" prop="UserName"> <span>{{ temp.UserName }}</span> </el-form-item> <el-form-item label="职位/职称:" prop="Position"> <el-input v-model="temp.Position" :maxlength="20" /> </el-form-item> <el-form-item label="固定电话:" prop="TelPhone"> <el-input v-model="temp.TelPhone" :maxlength="20" /> </el-form-item> <el-form-item label="手机:" prop="Phone"> <el-input v-model="temp.Phone" :maxlength="20"/> </el-form-item> <el-form-item label="邮件:" prop="Email"> <el-input v-model="temp.Email" :maxlength="50"/> </el-form-item> <el-form-item> <el-button type="primary" size="small" @click="dialogFormVisible = true">{{ someWord.changePassword }}</el-button> <el-button type="primary" @click="submitForm()">保存</el-button> </el-form-item> </el-form> </div> </div> <el-dialog :title="someWord.changePassword" :visible.sync="dialogFormVisible" :close-on-click-modal="false" width="400px"> <el-form ref="formPassword" :rules="formPasswordRules" :model="formPassword" label-position="right" label-width="100px" size="small" > <el-form-item label="原密码:" prop="oldPassword"> <el-input v-model="formPassword.oldPassword" type="password" maxlength="16"/> </el-form-item> <el-form-item label="新密码:" prop="newPassword"> <el-input v-model="formPassword.newPassword" type="password" maxlength="16"/> </el-form-item> <el-form-item label="确认密码:" prop="newPassword2"> <el-input v-model="formPassword.newPassword2" type="password" maxlength="16"/> </el-form-item> <el-form-item label="密码强度:" prop=""> <div id="tips" ><span>低</span><span>中</span> <span>高</span><span>很高</span> </div> </el-form-item> </el-form> <div slot="footer" class="dialog-footer"> <el-button @click="formPasswordCancel()">取 消</el-button> <el-button type="primary" @click="formPasswordUpdate()">确 定</el-button> </div> </el-dialog> </div> </template> <script> import { update, getInfo, changePassword } from '@/api/account' import waves from '@/directive/waves' // 水波纹指令 import Sticky from '@/components/Sticky' import { checkPassWordStrength } from '@/utils' export default { name: 'SubjectReportDetail', directives: { waves }, components: { Sticky }, props: { id: { type: Number, default: 0 } }, data() { return { spanDoms: null, passWordStrength: '', dialogFormVisible: false, loading: false, problemStatusOptions: [], finalResultOptions: [], subjectList: [], subjectNameOptions: [], chargeUserOptions: [], someWord: { changePassword: '修改密码' }, formPassword: { oldPassword: '', newPassword: '', newPassword2: '' }, temp: { UserID: undefined, CompanyID: undefined, Position: null, UserName: null, UserStatus: undefined, Password: null, RealName: null, Email: null, Phone: null, TelPhone: null, Address: null, Photo: null, InvestigatorID: undefined }, rules: { Email: [ { required: true, message: '不能为空' }, { type: 'email', message: '邮箱格式不对' } ], Phone: [ { required: true, message: '不能为空' }, { pattern: /^1[3|4|5|7|8][0-9]{9}$/, message: '请输入11位手机号码' } ] }, formPasswordRules: { oldPassword: [ { required: true, message: '不能为空!', trigger: 'blur' }, { min: 6, max: 16, message: '请输入6到16字' } ], newPassword: [ { required: true, message: '不能为空!', trigger: 'blur' }, { min: 6, max: 16, message: '请输入6到16字' } ], newPassword2: [ { required: true, message: '不能为空!', trigger: 'blur' }, { min: 6, max: 16, message: '请输入6到16字' } ] } } }, computed: { // Props,methods,data和computed的初始化都是在beforeCreated和created之间完成的。 }, watch: { 'formPassword.newPassword'(newValue, oldValue) { this.passWordStrength = checkPassWordStrength(newValue) }, 'passWordStrength'(newValue, oldValue) { // console.log(newValue, oldValue) const index = newValue if (index > 0) { const spanDoms = document.getElementById('tips').getElementsByTagName('span') for (let i = 0; i < spanDoms.length; i++) { spanDoms[i].className = ''// 清空span标签所有的class样式 if (index > 0) { // 0 代表false 其余代表true spanDoms[index - 1].className = 's' + index } } // console.log('spanDoms', spanDoms) } } }, created() { this.initInfo() }, mounted() { this.$nextTick(function() { }) }, methods: { formPasswordCancel() { this.dialogFormVisible = false this.$nextTick(() => { this.$refs['formPassword'].clearValidate() }) }, submitForm() { this.$refs['dataForm'].validate((valid) => { if (valid) { update(this.temp).then((response) => { if (response.data) { const mydata = response.data this.temp = mydata this.$notify({ title: '成功', message: '提交成功', type: 'success', duration: 2000 }) } }, error => { this.responseError(error) }) } }) }, formPasswordUpdate() { this.$refs['formPassword'].validate((valid) => { if (valid) { if (this.passWordStrength < 2) { this.$notify({ title: '警告', message: '密码强度低,请加入大小写字母和特殊符号!', type: 'warning', duration: 3000 }) return } if (this.formPassword.newPassword === this.formPassword.newPassword2) { changePassword(this.formPassword.oldPassword, this.formPassword.newPassword).then((response) => { if (response.success) { this.$notify({ title: '成功', message: '修改成功', type: 'success', duration: 2000 }) this.dialogFormVisible = false this.formPassword = { oldPassword: '', newPassword: '', newPassword2: '' } } }, error => { this.responseError(error) }) } else { this.$notify({ title: '警告', message: '新密码与确认密码必须相同!', type: 'warning', duration: 5000 }) } } }) }, resetTemp() { this.temp = { UserID: undefined, CompanyID: undefined, Position: null, UserName: null, UserStatus: undefined, Password: null, RealName: null, Email: null, Phone: null, TelPhone: null, Address: null, Photo: null, InvestigatorID: undefined } }, initInfo() { getInfo().then(response => { if (response.data) { this.temp = response.data.user } }, error => { this.responseError(error) }) }, responseError(error) { console.log(error) this.loading = false } } } </script>