一、题目
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself).”
二、题目大意
找出两个节点的最低公共祖先节点,允许这两个节点自身为公共祖先节点。
三、解题思路
对于这个问题只有两种情况:
- 当这两个节点处于不同的左右子树中时,那么最低公共祖先节点就是这两棵左右子树的根节点;
- 当这两个节点处于同一子树中,那么最低公共祖先节点就是这两个节点中最低的那个节点。
四、代码实现
const lowestCommonAncestor = (root, p, q) => {
if (!root || root == p || root == q) {
return root
}
const left = lowestCommonAncestor(root.left, p, q)
const right = lowestCommonAncestor(root.right, p, q)
if (left && right) {
return root
}
return left ? left : right
}
如果本文对您有帮助,欢迎关注微信公众号,为您推送更多内容,ε=ε=ε=┏(゜ロ゜;)┛。
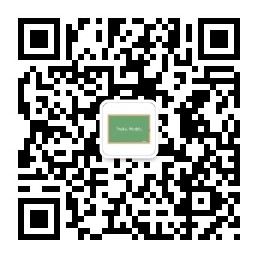