本文将介绍系统控件ExpandableListView的简要使用,主要学习了系统提供的二级菜单列表的实现方式。学习了指示展示效果。
效果1:
如下,修改了指示器的排列位置,列表左侧,现在修改为列表右侧。主要是设置android:layoutDirection 设置排列方式即能达到特定的效果。
<ExpandableListView
android:layoutDirection="rtl"
android:id="@+id/expandListView"
android:layout_width="match_parent"
android:layout_height="match_parent">
</ExpandableListView>
// item_parent
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layoutDirection="ltr"
android:layout_height="45dp">
....
</RelativeLayout>
// item_child
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="horizontal"
android:layoutDirection="ltr"
android:layout_height="60dp">
</LinearLayout>
效果2:
其实我只是想修改下指示的下标罢了,可是图标会拉伸很丑啊。暂时不知道为啥自己设置的groupInficator会是变形的,知道的大神请告知。尝试过设置.9图并没有什么用处。
<ExpandableListView
android:layoutDirection="rtl"
android:id="@+id/expandListView"
android:groupIndicator="@drawable/expand_group_indicator"
android:layout_width="match_parent"
android:layout_height="match_parent">
</ExpandableListView>
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_expanded="true" android:drawable="@drawable/shape_right"/>
<item android:drawable="@drawable/shape_bottom"/>
</selector>
效果3: 完全自定义的指示图标
好了下面开始贴代码咯,啧啧
核心adapter
public class EpLvOneAdapter extends BaseExpandableListAdapter{
private List<EpLvOneBean> mEpLvOneBeanList;
private Context mContext;
private SparseArray<ImageView> mIndicators = new SparseArray<>();
public EpLvOneAdapter(Context context,List<EpLvOneBean> epLvOneBeanList) {
mEpLvOneBeanList = epLvOneBeanList;
mContext = context;
}
@Override
public int getGroupCount() {
if (mEpLvOneBeanList != null){
return mEpLvOneBeanList.size();
}
return 0;
}
@Override
public int getChildrenCount(int groupPosition) {
if (mEpLvOneBeanList != null){
EpLvOneBean epLvOneBean = mEpLvOneBeanList.get(groupPosition);
if (null != epLvOneBean && null != epLvOneBean.getEpOneChildBeans()){
return epLvOneBean.getEpOneChildBeans().size();
}
}
return 0;
}
@Override
public Object getGroup(int groupPosition) {
return mEpLvOneBeanList.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
if ( null != mEpLvOneBeanList.get(groupPosition).getEpOneChildBeans()){
return mEpLvOneBeanList.get(groupPosition).getEpOneChildBeans().get(childPosition);
}
return null;
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
//每个item的id是否是固定?一般为true
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
GroupViewHolder groupViewHolder;
if (null == convertView){
groupViewHolder = new GroupViewHolder();
convertView = LayoutInflater.from(mContext).inflate(R.layout.item_one_group,parent,false);
groupViewHolder.tvGroupName = convertView.findViewById(R.id.tvGroupName);
groupViewHolder.tvGroupNum = convertView.findViewById(R.id.tvGroupNum);
groupViewHolder.ivIndicator = convertView.findViewById(R.id.ivIndicator);
convertView.setTag(groupViewHolder);
}else {
groupViewHolder = (GroupViewHolder) convertView.getTag();
}
EpLvOneBean epLvOneBean = mEpLvOneBeanList.get(groupPosition);
groupViewHolder.tvGroupName.setText(epLvOneBean.getName());
if (null != epLvOneBean.getEpOneChildBeans()) {
groupViewHolder.tvGroupNum.setText(String.valueOf(epLvOneBean.getEpOneChildBeans().size()));
}else {
groupViewHolder.tvGroupNum.setText("0");
}
if (mIndicators.get(groupPosition) == null) {
mIndicators.put(groupPosition, groupViewHolder.ivIndicator);
}
setIndicatorState(groupPosition, isExpanded);
return convertView;
}
public void setIndicatorState(int groupPosition, boolean isExpanded) {
if (isExpanded) {
mIndicators.get(groupPosition).setImageResource(R.drawable.shape_bottom);
} else {
mIndicators.get(groupPosition).setImageResource(R.drawable.shape_right);
}
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
EpOneChildBean epOneChildBean = mEpLvOneBeanList.get(groupPosition).getEpOneChildBeans().get(childPosition);
ChildViewHolder childViewHolder;
if (null == convertView){
childViewHolder = new ChildViewHolder();
convertView = LayoutInflater.from(mContext).inflate(R.layout.item_one_child,parent,false);
childViewHolder.tvChildName = convertView.findViewById(R.id.tvChildName);
childViewHolder.tvChildState = convertView.findViewById(R.id.tvChildState);
convertView.setTag(childViewHolder);
}else {
childViewHolder = (ChildViewHolder) convertView.getTag();
}
childViewHolder.tvChildName.setText(epOneChildBean.getChildName());
childViewHolder.tvChildState.setText(epOneChildBean.getChildState());
return convertView;
}
//二级列表中的item是否能够被选中?可以改为true
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
static class GroupViewHolder{
TextView tvGroupName;
TextView tvGroupNum;
ImageView ivIndicator;
}
static class ChildViewHolder{
TextView tvChildName;
TextView tvChildState;
}
}
item_one_group.xml 设置下拉图标的布局
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layoutDirection="ltr"
android:layout_height="45dp">
<TextView
android:id="@+id/tvGroupName"
android:text="特别关心"
android:textColor="#000"
android:layout_centerVertical="true"
android:textSize="16sp"
android:layout_marginLeft="6dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/tvGroupNum"
android:text="6"
android:layout_alignParentRight="true"
android:layout_centerVertical="true"
android:layout_marginRight="45dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<ImageView
android:layout_alignParentRight="true"
android:id="@+id/ivIndicator"
android:visibility="visible"
android:layout_marginRight="15dp"
android:layout_centerVertical="true"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:src="@drawable/shape_right"/>
</RelativeLayout>
group点击事件
扫描二维码关注公众号,回复:
4998597 查看本文章
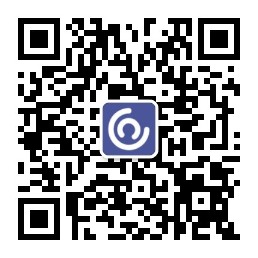
expandListView.setOnGroupClickListener(new ExpandableListView.OnGroupClickListener() {
@Override
public boolean onGroupClick(ExpandableListView parent, View v, int groupPosition, long id) {
boolean groupExpanded = parent.isGroupExpanded(groupPosition);
if (groupExpanded) {
parent.collapseGroup(groupPosition);
} else {
parent.expandGroup(groupPosition, true);
}
mEpLvOneAdapter.setIndicatorState(groupPosition, groupExpanded);
return true;
}
});
列表数据类型
public class EpLvOneBean {
private String name;
private List<EpOneChildBean> mEpOneChildBeans;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<EpOneChildBean> getEpOneChildBeans() {
return mEpOneChildBeans;
}
public void setEpOneChildBeans(List<EpOneChildBean> epOneChildBeans) {
mEpOneChildBeans = epOneChildBeans;
}
}
public class EpOneChildBean {
private String childName;
private String childState;
public String getChildName() {
return childName;
}
public void setChildName(String childName) {
this.childName = childName;
}
public String getChildState() {
return childState;
}
public void setChildState(String childState) {
this.childState = childState;
}
public EpOneChildBean(String childName, String childState) {
this.childName = childName;
this.childState = childState;
}
}
4. 技巧---默认展开且不可收缩
// 设置不可点击
expandListView.setOnGroupClickListener(new ExpandableListView.OnGroupClickListener() {
@Override
public boolean onGroupClick(ExpandableListView parent, View v, int groupPosition, long id) {
return true;
}
});
// 设置默认展开
int count = expandListView.getCount();
for (int i=0;i<count;i++){
expandListView.expandGroup(i);
}
参考资料:安卓的ExpandableListView的使用和优化
Android ExpandableListView使用小结
不错的recycle展开资料