一、环境配置
当前我的开发环境是Miniconda3+PyCharm。开发环境其实无所谓,自己使用Python3+Nodepad都可以。安装Flask库:
pip install Flask
二、第一个应用程序
将以下内容保存为helloworld.py:
# 导入Flask类 from flask import Flask # 实例化,可视为固定格式 app = Flask(__name__) # route()方法用于设定路由;类似spring路由配置 @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': # app.run(host, port, debug, options) # 默认值:host=127.0.0.1, port=5000, debug=false app.run()
直接运行该文件,然后访问:http://127.0.0.1:5000/helloworld。结果如下图:
三、get和post实现
3.1 创建用到的模板文件
Flask默认到templates目录下查找模板文件,在上边htlloworld.py同级目录下创建templates文件夹。
在templates文件夹下创建get.html,写入以下内容:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>get请求示例</title> </head> <body> <form action="/deal_request" method="get"> <input type="text" name="q" /> <input type="submit" value="搜索" /> </form> </body> </html>
再在templates文件夹下创建post.html,写入以下内容:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>post请求示例</title> </head> <body> <form action="/deal_request" method="post"> <input type="text" name="q" /> <input type="submit" value="搜索" /> </form> </body> </html>
最后在templates文件夹下创建result.html,写入以下内容:
<!-- Flask 使用Jinja2模板引擎,Jinja2模板引擎源于Django板模所以很多语法和Django是类似的 --> <h1>{{ result }}</h1>
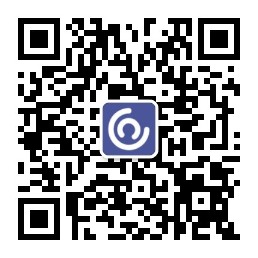
3.2 编写相关的处理方法
在helloworld.py中添加get_html()、post_html()和deal_request()三个方法,更多说明见注释。当前helloworld.py内容如下:
# 导入Flask类 from flask import Flask from flask import render_template from flask import request # 实例化,可视为固定格式 app = Flask(__name__) # route()方法用于设定路由;类似spring路由配置 #等价于在方法后写:app.add_url_rule('/', 'helloworld', hello_world) @app.route('/helloworld') def hello_world(): return 'Hello, World!' # 配置路由,当请求get.html时交由get_html()处理 @app.route('/get.html') def get_html(): # 使用render_template()方法重定向到templates文件夹下查找get.html文件 return render_template('get.html') # 配置路由,当请求post.html时交由post_html()处理 @app.route('/post.html') def post_html(): # 使用render_template()方法重定向到templates文件夹下查找post.html文件 return render_template('post.html') # 配置路由,当请求deal_request时交由deal_request()处理 # 默认处理get请求,我们通过methods参数指明也处理post请求 # 当然还可以直接指定methods = ['POST']只处理post请求, 这样下面就不需要if了 @app.route('/deal_request', methods = ['GET', 'POST']) def deal_request(): if request.method == "GET": # get通过request.args.get("param_name","")形式获取参数值 get_q = request.args.get("q","") return render_template("result.html", result=get_q) elif request.method == "POST": # post通过request.form["param_name"]形式获取参数值 post_q = request.form["q"] return render_template("result.html", result=post_q) if __name__ == '__main__': # app.run(host, port, debug, options) # 默认值:host=127.0.0.1, port=5000, debug=false app.run()
3.3 查看运行效果
重新运行helloworld.py。
当前目录结构如下(.idea目录不用管):
get.html如下:
get查询结果如下:
post.html如下:
post查询结果如下:
四、restful
4.1 安装flask-restful
restful我这里通过Flask-RESTful实现。
pip install flask-restful
4.2 实现rest代码
修改helloworld.py,添加三处,具体见注释
# 导入Flask类 from flask import Flask from flask import render_template from flask import request # 添加位置一。从Flask-RESTful中导入以下三项 from flask_restful import Api from flask_restful import Resource from flask_restful import reqparse # 实例化,可视为固定格式 app = Flask(__name__) # 添加位置二。 # 实例化一个api用于配置rest路由 # 实例化一个参数解析类,用于rest获取get和post提交的参数 api = Api(app) parser = reqparse.RequestParser() # 注册q参数parser才能解析get和post中的q参数。这种注册才能解析的要求是否有点孤儿 parser.add_argument('q', type=str, help='Rate to charge for this resource') # route()方法用于设定路由;类似spring路由配置 #等价于在方法后写:app.add_url_rule('/', 'helloworld', hello_world) @app.route('/helloworld') def hello_world(): return 'Hello, World!' # 配置路由,当请求get.html时交由get_html()处理 @app.route('/get.html') def get_html(): # 使用render_template()方法重定向到templates文件夹下查找get.html文件 return render_template('get.html') # 配置路由,当请求post.html时交由post_html()处理 @app.route('/post.html') def post_html(): # 使用render_template()方法重定向到templates文件夹下查找post.html文件 return render_template('post.html') # 配置路由,当请求deal_request时交由deal_request()处理 # 默认处理get请求,我们通过methods参数指明也处理post请求 # 当然还可以直接指定methods = ['POST']只处理post请求, 这样下面就不需要if了 @app.route('/deal_request', methods = ['GET', 'POST']) def deal_request(): if request.method == "GET": # get通过request.args.get("param_name","")形式获取参数值 get_q = request.args.get("q","") return render_template("result.html", result=get_q) elif request.method == "POST": # post通过request.form["param_name"]形式获取参数值 post_q = request.form["q"] return render_template("result.html", result=post_q) # 添加位置三。 # 添加rest类(是类而不是和Flask一样直接是方法) class Rest(Resource): # get提交时的处理方法 def get(self): result = {} args = parser.parse_args() result["method"] = "get" result["q"] = args["q"] return result # post提交时的处理方法 def post(self): result = {} args = parser.parse_args() result["method"] = "post" result["q"] = args["q"] return result # 配置路由 api.add_resource(Rest, '/rest') if __name__ == '__main__': # app.run(host, port, debug, options) # 默认值:host=127.0.0.1, port=5000, debug=false app.run()
4.3 查看运行结果
即然是restful我们也就不写html了,直接用curl模拟提交。重新运行helloworld.py,请求如下图(之前非rest的get和post当然也还能正常访问):
五、Flask与Django比较
我们经常会听说这样的一个观点:Django是Python最流行的Web框架但配置比较复杂,Flask是一个轻量级的框架配置比较简单如果项目比较小推荐使用Flask。
5.1 Django配置复杂
如果对Django不是很了解,可以参看“Python3+PyCharm+Django+Django REST framework开发教程”和“Python3+Django get/post请求实现教程 ”。
仅从文章长度看就比这篇长很多,所以Django比Flask复杂(得多)是肯定的。更具体比较如下:
比较项 | Django | Flask | 复杂度比较 | 说明 |
项目创建 | Django需要用命令创建项目 | Flask直接编写文件就可运行 | Django复杂 | Django需要用命令创建项目是因为要创建出整个项目框架 |
路由 | Django使用专门的urls.py文件 | Flask直接使用@app.route() | Django笨重 | Django类似Strut2的配置Flask类似Spring的配置,Flask感觉更好 |
get和post | request.GET['name']和request.POST["name"] | request.args.get("name","")和request.form["q"] | 差不多 | Flask格式上不统一 |
restful | 使用django-resful框架 | 使用flask-restful框架 | 差不多 | Flask使用方法flask-rest使用类格式不统一;flask-restful需要注册参数有点孤儿 |
数据库操作 | django集成了对数据库的操作 | Flask没集成对数据库的操作要另行直连或使用sqlalchemy | 差不多 | django复杂很大程度来源于对数据库的集成。 |
5.2 Flask适合使用场景
Django复杂来源于其“可能用到的功能都先集成”,Flask的轻量来源其“暂时不用的功能都先不做处理”。随着项目规模的扩大最终Django有的东西Flask也都需要有,而且由于没做统一规划Flask将处于劣势位置。
个人认为Flask适用以下两个场景:
一是开发者没有Spring等企业级框架开发经验,不然Django很多项为什么要配置为什么这样配置不那样配置比较难理解。
二是项目大概是十来个页面、就只有两三张数据表甚至不用数据库的规模,在这种程度下减少的配置工作还算能抵得过增加的开发工作量。
如果之前用过Spring之类的框架写过代码的话个人还是推荐不管项目大小都直接用Django,主要是Flask不统一的格式让人很难受。
参考: