一. 简介
Feign是简化Java HTTP客户端开发的工具(java-to-httpclient-binder),它的灵感来自于Retrofit、JAXRS-2.0和
WebSocket。Feign的初衷是降低统一绑定Denominator到HTTP API的复杂度,不区分是否为restful
二. 配置(谁需要调用微服务就在谁身上配置)
1. 在发起调用的微服务的pom文件中添加依赖
<!--feign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
2. 在该微服务的启动类中添加注解(开启发现的客户端)
@EnableDiscoveryClient
@EnableFeignClients
3. 在微服务下创建一个client包, 在包下创建接口
@RequestMapping和方法名,参数等都可以和想要调用的接口一致,只是要改为接口的形式。
import com.ihrm.common.entity.Result;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* 声明接口,通过feign调用其它微服务
*/
@FeignClient("ihrm-company")
public interface DepartmentFeignClient {
/**
* 调用微服务的接口
*/
@RequestMapping(value = "/company/department/{id}", method = RequestMethod.GET)
public Result findById(@PathVariable("id") String id);
}
到此,Feign组件配置完成。
三. 测试Feign组件
1. 编写测试接口
@Autowired
private DepartmentFeignClient departmentFeignClient;
/**
* 测试Feign组件
* 调用系统微服务的/test接口,传递部门id,通过feign调用部门微服务获取部门信息
*/
@RequestMapping(value = "test/{id}", method = RequestMethod.GET)
public Result testFeign(@PathVariable(value = "id") String id) {
return departmentFeignClient.findById(id);
}
2. 启动微服务
3. 使用postman调用失败(显示未登录),主要是权限框架拦截了请求
4. 配置Feign拦截器(要导入feign依赖)
扫描二维码关注公众号,回复:
5499718 查看本文章
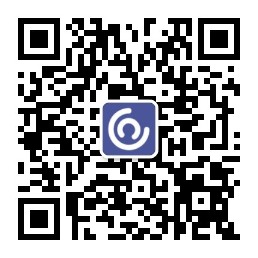
import feign.RequestInterceptor;
import feign.RequestTemplate;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
import java.util.Enumeration;
@Configuration
public class FeigmConfiguration {
//配置feign拦截器,解决请求头问题
@Bean
public RequestInterceptor requestInterceptor() {
return new RequestInterceptor() {
//获取所有浏览器发送的请求属性, 请求头赋值到feign
@Override
public void apply(RequestTemplate requestTemplate) {
//1. 获取请求属性
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
//2. 判断
if (attributes != null) {
//3. 获取request
HttpServletRequest request = attributes.getRequest();
//4. 获取浏览器的所有请求头
Enumeration<String> headerNames = request.getHeaderNames();
if (headerNames != null) {
//5. 遍历头信息
while (headerNames.hasMoreElements()) {
//6. 获取当前请求头属性名
String name = headerNames.nextElement();
//7. 根据属性名获取其对应值
String value = request.getHeader(name);
//8. 放入feign中
requestTemplate.header(name, value);
}
}
}
}
};
}
}
5. 重启微服务
6. 使用postman调用,调用成功