yagmail模块
python标准库中发送电子邮件的模块比较复杂,因此,有许多开原的库提供了更加易用的接口来发送电子邮件,其中yagmail是一个使用比较广泛的开原项目,yagmail底层依然使用了smtplib和email模块,但是yagmail提供了更好的接口,并具有更好的易读性
yagmail是开原项目,因此,在使用前需要安装
pip install yagmai
用法:

#连接邮箱服务器 yag = yagmail.SMTP(user='[email protected]', password='xxxx', host='smtp.163.com') #发送邮件 yag.send(to='[email protected]', cc='[email protected]',subject='这是测试邮件', contents='这是测试邮件的内容') #断开连接 yag.close()
pymysql模块

#pymysql操作数据库 import pymysql # 打开数据库连接 db = pymysql.connect(host="192.168.254.24", user="root", password="root", db="mysql", port=3306) # 使用cursor()方法获取操作游标 cur = db.cursor() # 1.查询操作 # 编写sql 查询语句 user 对应我的表名 sql = "select host,user,password from user" try: cur.execute(sql) # 执行sql语句 results = cur.fetchall() # 获取查询的所有记录 for i in results:#遍历结果 print(i) except Exception as e: raise e finally: db.close() # 关闭连接
paramiko模块

#通过paramiko模块连接主机运行bash命令 import paramiko hostname = '192.168.254.24' port = 22 username = 'root' password = 'root' ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(hostname=hostname,port=port,username=username,password=password) stdin, stdout, stderr = ssh.exec_command("ls -ltr") print(stdout.read().decode('utf-8')) #通过paramiko模块连接主机上传 import paramiko hostname = '192.168.254.24' port = 22 username = 'root' password = 'root' t=paramiko.Transport((hostname,port)) t.connect(username=username,password=password) sftp = paramiko.SFTPClient.from_transport(t) sftp.put(r'C:\Users\fengzi\Desktop\Linux.xmind', '/root/aaa.xmind') sftp.close() #通过paramiko模块连接主机下载 import paramiko hostname = '192.168.254.24' port = 22 username = 'root' password = 'root' t=paramiko.Transport((hostname,port)) t.connect(username=username,password=password) sftp = paramiko.SFTPClient.from_transport(t) sftp.get('/root/test3.yml', r'C:\Users\fengzi\Desktop\test3.yml') sftp.close()
pyinotify模块
pip install pyinotify
pyinotify提供的事件:
标志 | 事件含义 |
IN_ACCESS | 被监控项目或者被监控目录中的文件被访问,比如一个文件被读取 |
IN_MODIFY | 被监控项目或者被监控目录中的文件被修改 |
IN_ATTRIB | 被监控项目或者被监控目录中的文件的元数据被修改 |
IN_CLOSE_WRITE | 一个打开切等待写入的文件或者目录被关闭 |
IN_CLOSE_NOWRITE | 一个以只读方式打开的文件或者目录被关闭 |
IN_OPEN | 文件或者目录被打开 |
IN_MOVED_FROM | 被监控项目或者目录中的文件被移除监控区域 |
IN_MOVED_TO | 文件或目录被移入监控区域 |
IN_CREATE | 在所监控的目录中创建子目录或文件 |
IN_DELETE | 在所监控的目录中删除目录或文件 |
IN_CLOSE* | 文件被关闭,等同于IN_CLOSE_WRITE* |
IN_MOVE | 文件被移动,等同于IN_CLOSE_NOWRITE |
在具体实现时,时间仅仅是一个标志位,因此,我们可以使用“与”操作来合并多个时间,下面来看一个实例

import pyinotify #创建一个监控实例 wm = pyinotify.WatchManager() #定义要监控的内容 mask = pyinotify.IN_DELETE | pyinotify.IN_CREATE #这里pyinotify.ALL_EVENTS表示监控所有事件 #在实例中添加动作 wm.add_watch('/tmp', mask) #加载监控实例对象 notifier = pyinotify.Notifier(wm) #循环处理时间 notifier.loop()
configparse模块

一、ConfigParser简介 ConfigParser 是用来读取配置文件的包。配置文件的格式如下:中括号“[ ]”内包含的为section。section 下面为类似于key-value 的配置内容。 [db] db_host = 127.0.0.1 db_port = 69 db_user = root db_pass = root host_port = 69 [concurrent] thread = 10 processor = 20 括号“[ ]”内包含的为section。紧接着section 为类似于key-value 的options 的配置内容。 二、ConfigParser 初始化对象 使用ConfigParser 首选需要初始化实例,并读取配置文件: import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") 三、ConfigParser 常用方法 1、获取所用的section节点 # 获取所用的section节点 import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") print(config.sections()) #运行结果 # ['db', 'concurrent'] 2、获取指定section 的options。即将配置文件某个section 内key 读取到列表中: import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") r = config.options("db") print(r) #运行结果 # ['db_host', 'db_port', 'db_user', 'db_pass', 'host_port'] 3、获取指点section下指点option的值 import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") r = config.get("db", "db_host") # r1 = config.getint("db", "k1") #将获取到值转换为int型 # r2 = config.getboolean("db", "k2" ) #将获取到值转换为bool型 # r3 = config.getfloat("db", "k3" ) #将获取到值转换为浮点型 print(r) #运行结果 # 127.0.0.1 4、获取指点section的所用配置信息 import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") r = config.items("db") print(r) #运行结果 #[('db_host', '127.0.0.1'), ('db_port', '69'), ('db_user', 'root'), ('db_pass', 'root'), ('host_port', '69')] 5、修改某个option的值,如果不存在则会出创建 # 修改某个option的值,如果不存在该option 则会创建 import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") config.set("db", "db_port", "69") #修改db_port的值为69 config.write(open("ini", "w")) 运行结果 6、检查section或option是否存在,bool值 import configparser config = configparser.ConfigParser() config.has_section("section") #是否存在该section config.has_option("section", "option") #是否存在该option 7、添加section 和 option import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") if not config.has_section("default"): # 检查是否存在section config.add_section("default") if not config.has_option("default", "db_host"): # 检查是否存在该option config.set("default", "db_host", "1.1.1.1") config.write(open("ini", "w")) 运行结果 8、删除section 和 option import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") config.remove_section("default") #整个section下的所有内容都将删除 config.write(open("ini", "w")) 运行结果 9、写入文件 以下的几行代码只是将文件内容读取到内存中,进过一系列操作之后必须写回文件,才能生效。 import configparser config = configparser.ConfigParser() config.read("ini", encoding="utf-8") 写回文件的方式如下:(使用configparser的write方法) config.write(open("ini", "w"))
pexpect模块

import pexpect ip="127.0.0.1" name="root" pwd="root" #发送命令执行交互 child=pexpect.spawn('ssh %s@%s' % ("root",ip) ) child.expect ('password:') child.sendline(pwd) child.expect('$') child.sendline('df -h') #发送命令 child.sendline("exit") child.interact() #关闭pexpect child.close()
socket模块
半双工

#linux服务器(半双工) import socket import subprocess import threading server = socket.socket() server.bind(('', 8888)) server.listen(5) print('等待电话.....') conn, addr = server.accept() print('电话来了......') while True: data = conn.recv(10240) cmd = subprocess.Popen(data.decode('utf-8'), shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE) stdout = cmd.stdout.read() stderr = cmd.stdout.read() conn.send(stdout + stderr) #客户端 import socket import threading client = socket.socket() client.connect(('192.168.254.24', 8888)) while True: info = input('===>:') if not info:continue client.send(info.encode('utf-8')) data = client.recv(10240) print(data.decode('utf-8'))
全双工

#全双工电话 #服务器端 import socket import subprocess import threading server = socket.socket() server.bind(('', 8888)) server.listen(5) print('等待电话.....') conn, addr = server.accept() print('电话来了......') def recv(): while True: data = conn.recv(10240) print(data.decode('utf-8')) def send(): while True: data = input('===>:') conn.send(data.encode('utf-8')) t1 = threading.Thread(target=recv) t2 = threading.Thread(target=send) t1.start() t2.start() #客户端 import socket import threading client = socket.socket() client.connect(('localhost', 8888)) def send(): while True: info = input('===>:') client.send(info.encode('utf-8')) def recv(): while True: data = client.recv(1024) print(data.decode('utf-8')) t1 = threading.Thread(target=send) t2 = threading.Thread(target=recv) t1.start() t2.start()
socket监控服务端口
扫描二维码关注公众号,回复:
5626594 查看本文章
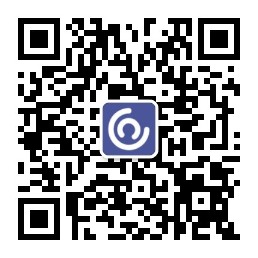

#监控服务端口,不通就发邮件报警 def sendmail(message, ip): import smtplib from email.mime.text import MIMEText from email.header import Header sender = '[email protected]' receiver = '[email protected]' subject = '%s报警' % ip username = '[email protected]' password = 'xxxx' msg = MIMEText(message, 'plain', 'utf-8') msg['Subject'] = Header(subject, 'utf-8') msg['From'] = 'Tim<[email protected]>' msg['To'] = "[email protected]" smtp = smtplib.SMTP() smtp.connect('smtp.163.com') smtp.login(username, password) smtp.sendmail(sender, receiver, msg.as_string()) smtp.quit() import re import socket socket.setdefaulttimeout(1) server = socket.socket() host_list = ['192.168.4.145:5555'] for info in host_list: ip = re.compile('(.*?):(.*)').search(info).group(1) port = re.compile('(.*?):(.*)').search(info).group(2) print(ip, port) res = server.connect_ex((ip, int(port))) if res != 0: sendmail('%s不通' % port, ip)
socket数据粘包
#nagle算法:会把数据量较小并且时间间隔较短的数据打包成一个发送给服务器

服务端: import socket import subprocess server = socket.socket() server.bind(('127.0.0.1', 8888)) server.listen(5) print('等待电话.....') conn, addr = server.accept() print('电话来了......') while True: data = conn.recv(1024) cmd = subprocess.Popen(data.decode('utf-8'), shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE) stdout = cmd.stdout.read() stderr = cmd.stdout.read() total_size = len(stdout + stderr) conn.send(str(total_size).encode('utf-8')) conn.send(stdout + stderr) 客户端: import socket client = socket.socket() client.connect(('127.0.0.1', 8888)) while True: info = input('===>:') if not info:continue client.send(info.encode('utf-8')) total_size = client.recv(1024) recv_size = 0 recv_data = b'' while recv_size < int(total_size.decode('utf-8')): data = client.recv(1024) recv_size += len(data) recv_data += data print(recv_data.decode('gbk')) print('总大小:%s\n接收大小:%s' % (total_size.decode('utf-8'), recv_size))
re模块:调用正则(.*?与.*很重要,熟记熟练)

import re import socket socket.setdefaulttimeout(1) server = socket.socket() host_list = ['192.168.4.145:5555','127.0.0.1:8888','2.2.2.2:80','3.3.3.3:3333'] for info in host_list: ip = re.compile('(.*?):(.*)').search(info).group(1) #.*?代表非贪婪匹配,即只匹配到第一个:即止 port = re.compile('(.*?):(.*)').search(info).group(2) #.*代表贪婪匹配 res = server.connect_ex((ip, int(port))) if res != 0: print('%s不通' % port, ip)
\w | 匹配字母数字 |
\W | 匹配非字母数字 |
\s | 匹配任意空白字符,等价于 [\t\n\r\f]. |
\S | 匹配任意非空字符 |
\d | 匹配任意数字,等价于 [0-9]. |
\D | 匹配任意非数字 |
\A | 匹配字符串开始 |
\Z | 匹配字符串结束,如果是存在换行,只匹配到换行前的结束字符串。c |
\z | 匹配字符串结束 |
\G | 匹配最后匹配完成的位置。 |
\b | 匹配一个单词边界,也就是指单词和空格间的位置。例如, 'er\b' 可以匹配"never" 中的 'er',但不能匹配 "verb" 中的 'er'。 |
\B | 匹配非单词边界。'er\B' 能匹配 "verb" 中的 'er',但不能匹配 "never" 中的 'er'。 |
\n, \t, 等. | 匹配一个换行符。匹配一个制表符。等 |
\1...\9 | 匹配第n个分组的子表达式。 |
\10 | 匹配第n个分组的子表达式,如果它经匹配。否则指的是八进制字符码的表达式。 |