车辆管理的界面
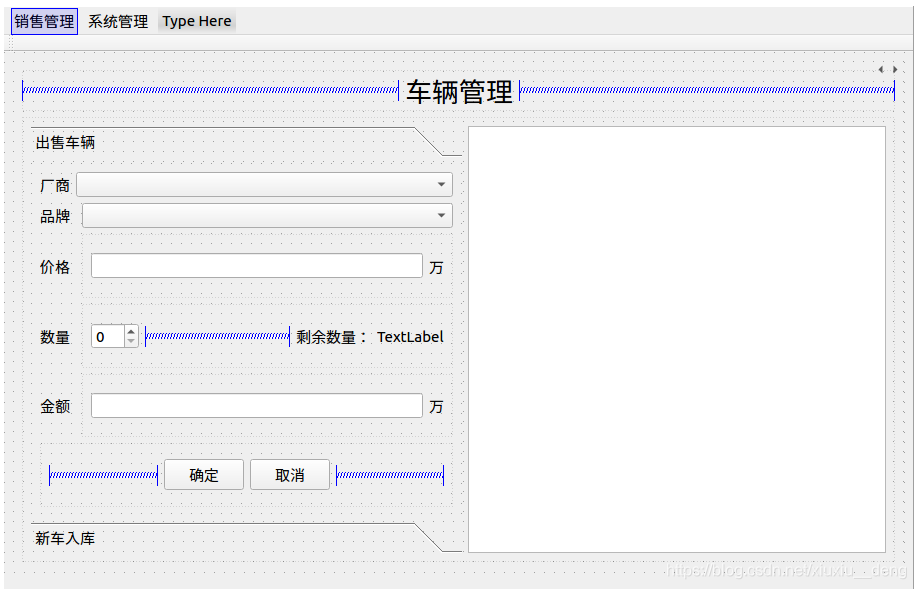
1.链接数据库,与前面链接数据库方法一样
2. 点击“车辆管理”菜单选项的时候,跳转到车辆管理页面
//设置当前默认页面为车辆管理的默认页面
ui->stackedWidget->setCurrentIndex(0);
//点击车辆管理菜单项的时候,跳转到车辆管理的页面
connect(ui->carManager,&QAction::triggered,this,[=](){
ui->stackedWidget->setCurrentIndex(0);
});
//点击销售统计的时候,页面跳转到销售统计
connect(ui->sell_count,&QAction::triggered,this,[=](){
ui->stackedWidget->setCurrentIndex(1);
});
3. 从数据库中查询所有的厂商,并设置到厂商后面的QComboBox中
void MainWindow::initSaleCar(){
//设置厂商
ui->factory->addItem("请选择厂商");
QSqlQuery query;
QString sql = "select name from factory";
query.exec(sql);
while (query.next()) {
ui->factory->addItem(query.value(0).toString());
}
fatoryChange("请选择厂商");
//brand随着厂商的改变而改变
connect(ui->factory,&QComboBox::currentTextChanged,this,&MainWindow::fatoryChange);
//价格随着brand的改变而改变
connect(ui->brand,&QComboBox::currentTextChanged,this,&MainWindow::brandChange);
//数量的变化,价格会随之变化
void (QSpinBox::*sigNumChanged)(int) = &QSpinBox::valueChanged;
connect(ui->num,sigNumChanged,this,&MainWindow::numChange);
}
4.厂商改变的时候,相应的品牌会随之改变
void MainWindow::fatoryChange(const QString str){
ui->brand->clear();
if(str == "请选择厂商"){
ui->price->clear();
ui->num->setValue(0);
ui->last->setText("0");
ui->sum_price->setEnabled(false);
ui->ok->setEnabled(false);
}else {
//查询对应厂商的品牌
QSqlQuery query;
QString sql = QString("select name from brand where fname = '%1'").arg(str);
query.exec(sql);
while (query.next()) {
ui->brand->addItem(query.value(0).toString());
}
}
}
5.品牌改变之后,该厂商所对应品牌的价格和剩余数量会随之改变
void MainWindow::brandChange(const QString str){
QSqlQuery query;
QString sql = QString("select price,last from brand where name = '%1' and fname = '%2'").arg(str).arg(ui->factory->currentText());
query.exec(sql);
while (query.next()) {
ui->price->setText(query.value(0).toString());
ui->last->setText(query.value(1).toString());
ui->num->setValue(0);
ui->sum_price->setEnabled(true);
ui->sum_price->setText("0");
}
}
6. 确定品牌之后,选择要购买的数量,总价格会随着购买数量的改变而改变
void MainWindow::numChange(int i){
//总价格
int price = ui->price->text().toInt();
int s_price = price * i;
// qDebug() << s_price;
ui->sum_price->setText(QString("%1").arg(s_price));
//设置确定按钮可以使用
if(s_price > 0){
ui->ok->setEnabled(true);
}else {
ui->ok->setEnabled(false);
}
}
7. 确定要购买的数量之后,点击确定(需要判断购买数量是否大于剩余数量)
void MainWindow::on_ok_clicked()
{
//点击确定的时候
//更新数据库
int sale_num = ui->num->value();
if(sale_num > ui->last->text().toInt()){
QMessageBox::warning(this,"警告","当前库存不足");
return;
}
//卖完的剩余数量
int last = ui->last->text().toInt() - sale_num;
ui->last->setText(QString("%1").arg(last));
QSqlQuery query;
QString sql = QString("select sell from brand where name = '%1' and fname = '%2'").arg(ui->brand->currentText()).arg(ui->factory->currentText());
query.exec(sql);
while (query.next()) {
int sell = query.value(0).toInt() + sale_num;
sql = QString("update brand set sell = %1,last = %2 where name = '%3' and fname = '%4'").arg(sell).arg(last)
.arg(ui->brand->currentText()).arg(ui->factory->currentText());
bool bl = query.exec(sql);
if(bl){
//显示销售记录
QDateTime current_time = QDateTime::currentDateTime();
QString strCurTime = current_time.toString("yyyy-MM-DD hh:mm:ss");
QString str = QString("%1 卖出了%2--%3,单价:%4,数量:%5,总价:%6").arg(strCurTime).arg(ui->factory->currentText()).arg(ui->brand->currentText())
.arg(ui->price->text()).arg(ui->num->value()).arg(ui->sum_price->text());
ui->listWidget->addItem(str);
QSqlDatabase::database().commit();
}else {
QSqlDatabase::database().rollback();
}
}
}
8. 点击取消按钮初始化车辆管理界面
void MainWindow::on_cancel_clicked()
{
fatoryChange("请选择厂商");
}