版权声明:本文为博主NJU_ChopinXBP原创文章,发表于CSDN,仅供交流学习使用,转载请私信或评论联系,未经博主允许不得转载。感谢您的评论与点赞。 https://blog.csdn.net/qq_20304723/article/details/87923571
2019.2.25 《剑指Offer》从零单刷个人笔记整理(66题全)目录传送门
之前有做过一道镜像二叉树和一道同构二叉树,比这道对称二叉树的判断要难得多,思路还是递归:
#数据结构与算法学习笔记#剑指Offer17:镜像二叉树+升级版同构二叉树+测试用例(Java、C/C++)
这道题的递归判断条件很简单:
1.若为叶子结点,返回true;
2.若只有左子树或右子树,返回false;
3.如果两棵子树都有,看左子结点和右子节点是否相同,并进行递归判断。
题目描述
请实现一个函数,用来判断一颗二叉树是不是对称的。注意,如果一个二叉树同此二叉树的镜像是同样的,定义其为对称的。
Java实现:
/**
*
* @author ChopinXBP
* 请实现一个函数,用来判断一颗二叉树是不是对称的。注意,如果一个二叉树同此二叉树的镜像是同样的,定义其为对称的。
*
*/
public class IsSymmetrical_57 {
public static class TreeNode {
int val = 0;
TreeNode left = null;
TreeNode right = null;
public TreeNode(int val) {
this.val = val;
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
}
public static boolean isSymmetrical(TreeNode pRoot)
{
if(pRoot == null)return true;
return Solution(pRoot.left, pRoot.right);
}
public static boolean Solution(TreeNode pleft, TreeNode pright) {
if(pleft == null && pright == null)return true;
if(pleft == null ^ pright == null)return false;
return pleft.val == pright.val && Solution(pleft.right, pright.left) && Solution(pleft.left, pright.right);
}
}
C++实现示例:
扫描二维码关注公众号,回复:
5817301 查看本文章
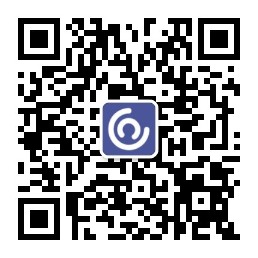
/*
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
TreeNode(int x) :
val(x), left(NULL), right(NULL) {
}
};
*/
class Solution {
public:
bool isSymmetrical(TreeNode* pRoot)
{
return isSymmetrical(pRoot,pRoot);
}
bool isSymmetrical(TreeNode* pRoot1,TreeNode* pRoot2)
{
if(pRoot1==nullptr&&pRoot2==nullptr)
return true;
if(pRoot1==nullptr||pRoot2==nullptr)
return false;
if(pRoot1->val!=pRoot2->val)
return false;
return isSymmetrical(pRoot1->left,pRoot2->right)&& isSymmetrical(pRoot1->right,pRoot2->left);
}
};
#Coding一小时,Copying一秒钟。留个言点个赞呗,谢谢你#