Date与String类型转换
将Date格式化为String String format(Date d)
将String解析为Date Date parse(String s)
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
//1、日期转字符串
Date date = new Date();
String dateStringParse = sdf.format(date);
//2、字符串转日期
String dateString = "2018-03-12 14:44:08";
Date dateParse = sdf.parse(dateString);
} catch (ParseException e) {
e.printStackTrace();
}
注意:创建 SimpleDateFormat 对象时指定转换格式
yyyy代表年,MM代表月,dd代表天(日),HH代表24进制的小时,hh 代表12进制的小时,mm代表分钟,ss代表秒
判断一个字符串是否是一个合法日期
public static boolean isDate(String str) {
boolean flag = true;
// 判个字符串是否是 yyyy/MM/dd 格式的日期
SimpleDateFormat sdf= new SimpleDateFormat("yyyy/MM/dd");
try {
// 设置lenient为false,否则SimpleDateFormat会比较宽松地验证日期。 比如2007/02/29会被接受,并转换成2007/03/01
sdf.setLenient(false);
sdf.parse(str);
} catch (ParseException e) {
convertSuccess=false; //格式不对
}
return flag;
}
获取当前月的上个月
public String getLastMonth() {
SimpleDateFormat format = new SimpleDateFormat("yyyyMM");
Date date = new Date();
Calendar calendar = Calendar.getInstance();
calendar.setTime(date); // 设置为当前时间
calendar.set(Calendar.MONTH, calendar.get(Calendar.MONTH) - 1); // 设置为上一个月
date = calendar.getTime();
String accDate = format.format(date);
return accDate;
}
计算两个日期的时间间隔
public static String getDatePoor(Date endDate, Date nowDate) {
long nd = 1000 * 24 * 60 * 60;
long nh = 1000 * 60 * 60;
long nm = 1000 * 60;
long ns = 1000;
// 获得两个时间的毫秒时间差异
long diff = endDate.getTime() - nowDate.getTime();
// 计算差多少天
long day = diff / nd;
// 计算差多少小时
long hour = diff % nd / nh;
// 计算差多少分钟
long min = diff % nd % nh / nm;
// 计算差多少秒//输出结果
long sec = diff % nd % nh % nm / ns;
// 输出 相差n天n小时n分钟n毫秒
return "相差" + day + "天" + hour + "小时" + min + "分钟" + sec + "秒";
}
补充:在uap平台中,采用UFDouble类型的方法,可以直接得到小数(如:相差1.25天)
uap平台计算时间间隔:
long nh = 1000 * 60 * 60;
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
Date signDate = sdf.parse(signTime);
Date endDate = sdf.parse(endTime);
// 先获得两个时间的毫秒时间差异
long diff = endDate.getTime() - signDate.getTime();
calTime = SafeCompute.div(new UFDouble(diff), new UFDouble(nh));
calTime = calTime.setScale(1, UFDouble.ROUND_HALF_UP); //四舍五入,小数点后1位
} catch (ParseException e) {
// TODO 自动生成的 catch 块
MessageDialog.showErrorDlg(null, "错误", "计算时间有误");
e.printStackTrace();
}
某时间的前/后n天
/**
* @author dch
* 传值-前一天 operDate("yyyyMMdd","20181111", -1)
*/
public static String operDate(String format, String str, int day) {
Date date;
try {
date = new SimpleDateFormat(format).parse(str);
// 当前日期
SimpleDateFormat format = new SimpleDateFormat(format);// 格式化对象
Calendar calendar = Calendar.getInstance();// 日历对象
calendar.setTime(date);// 设置当前日期
calendar.add(Calendar.DAY_OF_MONTH, day);// 加、减
return format.format(calendar.getTime());
} catch (Exception e) {
e.printStackTrace();
}
return "";
}
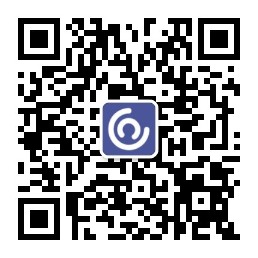