1:使用泛型接口
public class ILogin<T> {
private String message;
private String status;
private T result;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public T getResult() {
return result;
}
public void setResult(T result) {
this.result = result;
}
}
2:接口
@Entity
public class Login {
/**
* headPic : http://172.17.8.100/images/small/head_pic/2018-12-20/20181220194510.jpg
* nickName : 35_N3B12
* phone : 17701283117
* sessionId : 1546066355444107
* sex : 1
* userId : 107
*/
private String headPic;
private String nickName;
private String phone;
private String sessionId;
private int sex;
@Id
private int userId;
@Generated(hash = 1912988326)
public Login(String headPic, String nickName, String phone, String sessionId, int sex,
int userId) {
this.headPic = headPic;
this.nickName = nickName;
this.phone = phone;
this.sessionId = sessionId;
this.sex = sex;
this.userId = userId;
}
@Generated(hash = 1827378950)
public Login() {
}
public String getHeadPic() {
return this.headPic;
}
public void setHeadPic(String headPic) {
this.headPic = headPic;
}
public String getNickName() {
return this.nickName;
}
public void setNickName(String nickName) {
this.nickName = nickName;
}
public String getPhone() {
return this.phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getSessionId() {
return this.sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public int getSex() {
return this.sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public int getUserId() {
return this.userId;
}
public void setUserId(int userId) {
this.userId = userId;
}
}
3:使用接口拼接
创建一个类
public class StringUrl {
/**
* 登录注册
*/
public static final String LOGIN_URL="http://172.17.8.100/small/user/v1/";
}
4:接口拼接类
public interface ApiService {
/**
* 登录
* @param phone
* @param pwd
* @return
*/
@POST("login")
@FormUrlEncoded
Observable<ILogin<Login>> getlogin(@Field("phone") String phone,@Field("pwd")String pwd);
/**
* 注册
* @param phone
* @param pwd
* @return
*/
@POST("register")
@FormUrlEncoded
Observable<ILogin<Login>> getregister(@Field("phone") String phone,@Field("pwd") String pwd);
}
5:工具类LoginOkHttpUtils
public class LoginOkHttpUtils {
private static LoginOkHttpUtils instance;
private Retrofit retrofit;
public LoginOkHttpUtils(){
init();
}
public static LoginOkHttpUtils getInstance(){
if (instance ==null){
instance = new LoginOkHttpUtils();
}
return instance;
}
private void init() {
HttpLoggingInterceptor loggingInterceptor = new HttpLoggingInterceptor();
loggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BASIC);
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(loggingInterceptor)
.build();
retrofit = new Retrofit.Builder()
.addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.baseUrl(StringUrl.LOGIN_URL)
.client(client)
.build();
}
//把接口的注解翻译为OKhttp请求
public <T> T create(final Class<T> service) {
return retrofit.create(service);
}
}
6:登录布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/login">
<LinearLayout
android:layout_marginLeft="@dimen/dp_40"
android:layout_marginTop="@dimen/dp_160"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:layout_width="@dimen/dp_25"
android:layout_height="@dimen/dp_40"
android:background="@drawable/sj"
></ImageView>
<EditText
android:layout_marginLeft="@dimen/dp_20"
android:layout_marginTop="@dimen/dp_10"
android:id="@+id/login_phone"
android:hint="手机号"
android:textColorHint="#fff"
android:textColor="#fff"
android:textSize="@dimen/sp_18"
android:background="@null"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
<View
android:layout_marginTop="@dimen/dp_10"
android:layout_marginLeft="@dimen/dp_30"
android:layout_marginRight="@dimen/dp_50"
android:background="#fff"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_1"/>
<LinearLayout
android:layout_marginLeft="@dimen/dp_40"
android:layout_marginTop="@dimen/dp_20"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:layout_width="@dimen/dp_25"
android:layout_height="@dimen/dp_35"
android:background="@drawable/suo"
></ImageView>
<EditText
android:layout_marginLeft="@dimen/dp_20"
android:layout_marginTop="@dimen/dp_10"
android:id="@+id/login_password"
android:hint="登录密码"
android:inputType="textPassword"
android:textColor="#fff"
android:textColorHint="#fff"
android:layout_weight="1"
android:textSize="@dimen/sp_18"
android:background="@null"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/login_eye_check"
android:layout_marginRight="@dimen/dp_10"
android:layout_marginTop="@dimen/dp_10"
android:layout_width="@dimen/dp_28"
android:background="@drawable/yj"
android:layout_height="@dimen/dp_24" />
</LinearLayout>
<View
android:layout_marginTop="@dimen/dp_10"
android:layout_marginLeft="@dimen/dp_30"
android:layout_marginRight="@dimen/dp_50"
android:background="#fff"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_1"/>
<LinearLayout
android:layout_marginLeft="@dimen/dp_30"
android:layout_marginTop="@dimen/dp_20"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<CheckBox
android:id="@+id/login_jizhu"
android:layout_width="@dimen/dp_12"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="记住密码"
android:background="@null"
android:textSize="@dimen/sp_14"
style="@style/MyCheckBox"
android:textColor="#fff"/>
<TextView
android:id="@+id/login_zhuce"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#fff"
android:textSize="@dimen/sp_14"
android:text="快速注册" />
</LinearLayout>
<Button
android:id="@+id/login_button"
android:layout_width="@dimen/dp_280"
android:layout_marginTop="@dimen/dp_50"
android:layout_height="@dimen/dp_40"
android:text="登录"
android:textSize="@dimen/sp_18"
android:textColor="#ff5f71"
android:background="@drawable/shapelogin"
android:layout_marginLeft="@dimen/dp_30"
android:layout_marginRight="@dimen/dp_30"
/>
</LinearLayout>
7:抽取基类
public abstract class BaseActvity extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(getcontentview());
initView();
initData();
}
/**
* 初始化数据
*/
public abstract void initData();
/**
* 初始化控件
*/
public abstract void initView();
/**
* 初始化布局
* @return
*/
public abstract int getcontentview();
}
8:登录actvity代码
public class LoginActivity extends BaseActvity {
@BindView(R.id.login_phone)
EditText loginPhone;
@BindView(R.id.login_password)
EditText loginPassword;
@BindView(R.id.login_eye_check)
ImageView loginEyeCheck;
@BindView(R.id.login_jizhu)
CheckBox loginJizhu;
@BindView(R.id.login_zhuce)
TextView loginZhuce;
@BindView(R.id.login_button)
Button loginButton;
private String username;
private String password;
private SharedPreferences sharedPreferences;
/**
* 初始化数据
*/
@Override
public void initData() {
loginPassword.setInputType(129);
}
/**
* 初始化控件
*/
@Override
public void initView() {
ButterKnife.bind(this);
sharedPreferences = getSharedPreferences("simin",MODE_PRIVATE);
rememberThePassword();
}
private void rememberThePassword() {
//判断记住密码复选框是否选中
if (sharedPreferences.getBoolean("rememberPassword",false)){
loginJizhu.setChecked(true);
loginPhone.setText(sharedPreferences.getString("phone",""));
loginPassword.setText(sharedPreferences.getString("pwd",""));
}
//点击复选框记住密码
loginJizhu.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked){
sharedPreferences.edit().putBoolean("rememberPassword",true).commit();
}else {
sharedPreferences.edit().putBoolean("rememberPassword",false).commit();
}
}
});
}
/**
* 初始化布局
* @return
*/
@Override
public int getcontentview() {
return R.layout.activity_login;
}
@OnClick({R.id.login_eye_check, R.id.login_zhuce, R.id.login_button})
public void onViewClicked(View view) {
switch (view.getId()) {
case R.id.login_eye_check://点击小眼睛显示或隐藏密码
// //判断密码类型
if (loginPassword.getInputType() ==129){
loginPassword.setInputType(127);
}else {
loginPassword.setInputType(129);
}
int index=loginPassword.getText().toString().length();
loginPassword.setSelection(index);
break;
case R.id.login_zhuce://跳转注册页
Intent intent = new Intent(LoginActivity.this,RegisteActivity.class);
startActivity(intent);
break;
case R.id.login_button://点击登录
username = loginPhone.getText().toString().trim();
password = loginPassword.getText().toString().trim();
if (TextUtils.isEmpty(username)&& TextUtils.isEmpty(password)) {
Toast.makeText(this, "账号和密码不能为空", Toast.LENGTH_SHORT).show();
} else {
ApiService apiService = LoginOkHttpUtils.getInstance().create(ApiService.class);
apiService.getlogin(username,password)
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Consumer<ILogin<Login>>() {
@Override
public void accept(ILogin<Login> loginILogin) throws Exception {
if (loginILogin.getStatus().equals("0000")){
Toast.makeText(LoginActivity.this, "登录成功", Toast.LENGTH_SHORT).show();
//登录成功记住密码
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putString("phone",username);
edit.putString("pwd",password);
edit.commit();
//将用户信息存入数据库
DaoSession daoSession = DaoMaster.newDevSession(LoginActivity.this, LoginDao.TABLENAME);
LoginDao loginDao = daoSession.getLoginDao();
loginILogin.getResult().setStatues(1);
loginDao.insertOrReplace(loginILogin.getResult());
Intent intent = new Intent(LoginActivity.this,MainActivity.class);
startActivity(intent);
finish();
}
}
});
}
break;
}
}
}
9:注册布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/login">
<LinearLayout
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginTop="@dimen/dp_130"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:layout_width="@dimen/dp_25"
android:layout_height="@dimen/dp_35"
android:background="@drawable/sj"
></ImageView>
<EditText
android:layout_marginLeft="@dimen/dp_20"
android:layout_marginTop="@dimen/dp_10"
android:id="@+id/register_phone"
android:hint="手机号"
android:textColor="#fff"
android:textColorHint="#fff"
android:background="@null"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
<View
android:layout_marginTop="@dimen/dp_13"
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginRight="@dimen/dp_50"
android:background="#fff"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_2"/>
<LinearLayout
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginTop="@dimen/dp_20"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:layout_width="@dimen/dp_25"
android:layout_height="@dimen/dp_35"
android:background="@drawable/yzm"
></ImageView>
<EditText
android:layout_marginLeft="@dimen/dp_20"
android:layout_marginTop="@dimen/dp_10"
android:id="@+id/register_yanzheng"
android:hint="验证码"
android:textColor="#fff"
android:textColorHint="#fff"
android:background="@null"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/register_huoqu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/sp_10"
android:layout_marginLeft="@dimen/dp_110"
android:layout_marginTop="@dimen/dp_10"
android:text="获取验证码"
android:textColor="#fff"/>
</LinearLayout>
<View
android:layout_marginTop="@dimen/dp_13"
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginRight="@dimen/dp_50"
android:background="#fff"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_2"/>
<LinearLayout
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginTop="@dimen/dp_15"
android:layout_marginRight="@dimen/dp_50"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:layout_width="@dimen/dp_25"
android:layout_height="@dimen/dp_35"
android:background="@drawable/suo"
></ImageView>
<EditText
android:layout_marginLeft="@dimen/dp_20"
android:layout_marginTop="@dimen/dp_10"
android:id="@+id/register_password"
android:hint="登录密码"
android:layout_weight="1"
android:textColor="#fff"
android:background="@null"
android:textColorHint="#fff"
android:inputType="textPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/register_eye_check"
android:layout_marginRight="@dimen/dp_10"
android:layout_marginTop="@dimen/dp_10"
android:src="@drawable/yj"
android:layout_width="@dimen/dp_40"
android:layout_height="@dimen/dp_30" />
</LinearLayout>
<View
android:layout_marginTop="@dimen/dp_13"
android:layout_marginLeft="@dimen/dp_50"
android:layout_marginRight="@dimen/dp_50"
android:background="#fff"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_2"/>
<TextView
android:id="@+id/register_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/dp_10"
android:layout_marginRight="@dimen/dp_50"
android:text="已有账户?立即登录"
android:textColor="#fff"
android:textSize="@dimen/sp_14"
android:gravity="end"/>
<Button
android:id="@+id/register_zhuce"
android:layout_width="@dimen/dp_280"
android:layout_marginTop="@dimen/dp_30"
android:layout_height="wrap_content"
android:text="注册"
android:textSize="@dimen/sp_18"
android:textColor="#ff5f71"
android:background="@drawable/shapelogin"
android:layout_marginLeft="@dimen/dp_30"
android:layout_marginRight="@dimen/dp_30"
android:layout_gravity="center"/>
</LinearLayout>
10:注册代码
扫描二维码关注公众号,回复:
6223851 查看本文章
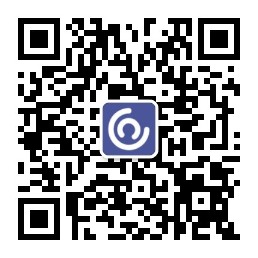
public class RegisteActivity extends BaseActvity {
@BindView(R.id.register_phone)
EditText registerPhone;
@BindView(R.id.register_yanzheng)
EditText registerYanzheng;
@BindView(R.id.register_huoqu)
TextView registerHuoqu;
@BindView(R.id.register_password)
EditText registerPassword;
@BindView(R.id.register_eye_check)
ImageView registerEyeCheck;
@BindView(R.id.register_login)
TextView registerLogin;
@BindView(R.id.register_zhuce)
Button registerZhuce;
private String phone;
private String password;
@Override
public void initData() {
registerPassword.setInputType(129);
}
@Override
public void initView() {
ButterKnife.bind(this);
}
@Override
public int getcontentview() {
return R.layout.activity_registe;
}
@OnClick({R.id.register_eye_check, R.id.register_login, R.id.register_zhuce,R.id.register_huoqu})
public void onViewClicked(View view) {
switch (view.getId()) {
case R.id.register_huoqu:
if (TextUtils.isEmpty(phone)){
Toast.makeText(this, "发送成功,请在手机上查收", Toast.LENGTH_SHORT).show();
}
break;
case R.id.register_eye_check:
//判断密码类型
if (registerPassword.getInputType() ==129){
registerPassword.setInputType(127);
}else {
registerPassword.setInputType(129);
}
break;
case R.id.register_login:
Intent intent = new Intent(RegisteActivity.this,LoginActivity.class);
startActivity(intent);
break;
case R.id.register_zhuce:
phone = registerPhone.getText().toString().trim();
password = registerPassword.getText().toString().trim();
if (TextUtils.isEmpty(phone) && TextUtils.isEmpty(password)){
Toast.makeText(this, "手机号与密码不能为空", Toast.LENGTH_SHORT).show();
}else {
ApiService apiService = LoginOkHttpUtils.getInstance().create(ApiService.class);
apiService.getregister(phone,password)
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(new Consumer<ILogin<Login>>() {
@Override
public void accept(ILogin<Login> loginILogin) throws Exception {
if (loginILogin.getStatus().equals("1001")){
Toast.makeText(RegisteActivity.this, "该手机号已注册,不能重复注册!", Toast.LENGTH_SHORT).show();
}else if (loginILogin.getStatus().equals("0000")){
Toast.makeText(RegisteActivity.this, "注册成功!", Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(RegisteActivity.this, "注册失败!", Toast.LENGTH_SHORT).show();
}
}
});
}
break;
}
}
}