GitHub 简介
Annotation-triggered注解触发 method call方法调用 logging for your debug builds调试版本.
As a programmer, you often add log statements片段、语句 to print method calls, their arguments, their return values, and the time it took to execute.
This is not a question. Every one of you does this. Shouldn't it be easier?
Simply add @DebugLog to your methods and you will automatically自动 get all of the things listed above logged for free.
@DebugLog
public String getName(String first, String last) {
SystemClock.sleep(15); // Don't ever really do this!
return first + " " + last;
}
public String getName(String first, String last) {
SystemClock.sleep(15); // Don't ever really do this!
return first + " " + last;
}
V/Example: ⇢ getName(first="Jake", last="Wharton")
V/Example: ⇠ getName [16ms] = "Jake Wharton"
V/Example: ⇢ getName(first="Jake", last="Wharton")
V/Example: ⇠ getName [16ms] = "Jake Wharton"
The logging will only happen in debug builds and the annotation itself is never present存在于 in the compiled class file编译的类文件 for any build type.
This means you can keep the annotation and check it into检入 source control源代码控制. It has zero effect on non-debug builds.
Add it to your project today!
Local Development
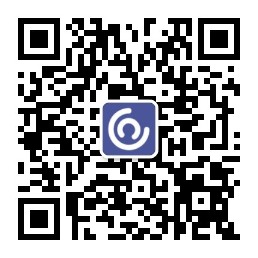
(不知道这几个步骤是搞什么玩意的)Working on this project? Here's some helpful Gradle tasks:
- install - Install plugin, runtime, and annotations into local repo.
- cleanExample - Clean the example project build.
- assembleExample - Build the example project. Must run install first.
- installExample - Build and install the example project debug APK onto a device.
使用步骤
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'com.jakewharton.hugo:hugo-plugin:1.2.1'
}
}
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'com.jakewharton.hugo:hugo-plugin:1.2.1'
}
}
2、在module的 build.gradle 中添加:
apply plugin: 'com.jakewharton.hugo'
apply plugin: 'com.jakewharton.hugo'
3、(此配置应该是废弃了)Disable logging temporarily暂时 by adding the following:
hugo {
enabled false
}
hugo {
enabled false
}
(此配置应该是废弃了)If you want to toggle logging at runtime, use
Hugo.setEnabled(true|false)
Hugo.setEnabled(true|false)
4、在需要的的类、方法、构造方法上加上注解即可
@DebugLog
实现原理简介
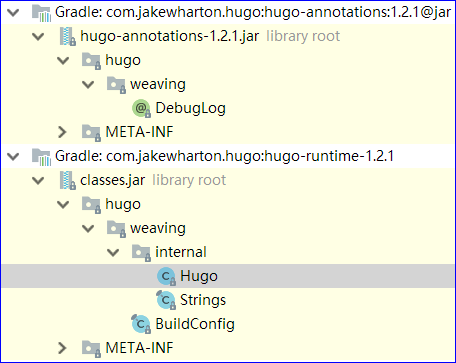
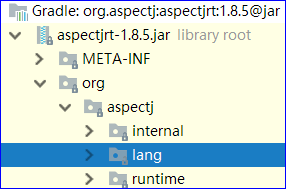
@Target({TYPE, METHOD, CONSTRUCTOR}) //注意类是TYPE的一种)和方法,所以可以用在类上
@Retention(CLASS) //保留策略为CLASS,即编译器将把注解记录在类文件中,因为不是RUNTIME,所以运行时注解丢掉了
//注意,如果我们需要在运行时获取注解信息(比如注解中有字段且需要在运行时获取到此字段的值),则Retention必须设为【RUNTIME】
public @interface DebugLog {
}
TYPE, METHOD, CONSTRUCTOR}) //注意类是TYPE的一种)和方法,所以可以用在类上 ({
CLASS) //保留策略为CLASS,即编译器将把注解记录在类文件中,因为不是RUNTIME,所以运行时注解丢掉了 (
//注意,如果我们需要在运行时获取注解信息(比如注解中有字段且需要在运行时获取到此字段的值),则Retention必须设为【RUNTIME】
public @interface DebugLog {
}
@Around("method() || constructor()")
public Object logAndExecute(ProceedingJoinPoint joinPoint) throws Throwable {
enterMethod(joinPoint); //ProceedingJoinPoint有参数信息,输出参数的值
long startNanos = System.nanoTime();//函数执行前记录时间,像我们手动做的一样
Object result = joinPoint.proceed();//这里代表我们监控的函数
long stopNanos = System.nanoTime();//函数执行结束时,打点记录时间,并计算耗时
long lengthMillis = TimeUnit.NANOSECONDS.toMillis(stopNanos - startNanos);
exitMethod(joinPoint, result, lengthMillis);//输出函数的值,执行耗时
return result;
}
"method() || constructor()") (
public Object logAndExecute(ProceedingJoinPoint joinPoint) throws Throwable {
enterMethod(joinPoint); //ProceedingJoinPoint有参数信息,输出参数的值
long startNanos = System.nanoTime();//函数执行前记录时间,像我们手动做的一样
Object result = joinPoint.proceed();//这里代表我们监控的函数
long stopNanos = System.nanoTime();//函数执行结束时,打点记录时间,并计算耗时
long lengthMillis = TimeUnit.NANOSECONDS.toMillis(stopNanos - startNanos);
exitMethod(joinPoint, result, lengthMillis);//输出函数的值,执行耗时
return result;
}
PS:Hugo运行还离不开自己JakeWharton开发的一个编译器,值加上以上jar包或依赖,在Android框架下并不会执行。
2018-4-23