一直以来做对外的接口文档都比较原始,基本上都是手写的文档传来传去,最近发现了一个新玩具,可以在接口上省去不少麻烦。
swagger是一款方便展示的API文档框架。它可以将接口的类型最全面的展示给对方开发人员,避免了手写文档的片面和误差行为。
swagger目前有两种swagger和swagger2两种,1比较麻烦,所以不考虑使用。本文主要记录我用swagger2做对外接口的两种方式,方面后面查阅。
使用传统的springmvc整合swagger2
maven依赖
<!--springfox依赖--> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.8.0</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.6.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.6.3</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.4.0</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.4.0</version> </dependency>
spring-mvc.xml 中添加映射静态的配置
<!-- swagger静态文件路径 --> <mvc:resources location="classpath:/META-INF/resources/" mapping="swagger-ui.html"/> <mvc:resources location="classpath:/META-INF/resources/webjars/" mapping="/webjars/**"/>
swagger2的配置类:
@Configuration @EnableSwagger2 public class SwaggerConfig extends WebMvcConfigurationSupport { @Bean public Docket createRestApi() { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() .apis(RequestHandlerSelectors.basePackage("net.laoyeyey.yyblog")) .paths(PathSelectors.any()) .build(); } private ApiInfo apiInfo() { return new ApiInfoBuilder() .title("yyblog项目 RESTful APIs") .description("yyblog项目api接口文档") .version("1.0") .build(); } }
paths如果在生产情况下可以调整为PathSelectors.none(),即不显示所有接口信息;
接口信息配置
即在SpringMvc的Controller中配置相关的接口信息
@Controller @RequestMapping(value = "aitou") @Api(description = "测试服务-账户信息查询") public class DailyOperationDataController { Logger logger = Logger.getLogger(DailyOperationDataController.class); @Autowired private DailyOperationDataService DailyOperationDataService; /* * @ApiOperation(value = "接口说明", httpMethod ="接口请求方式", response ="接口返回参数类型", notes ="接口发布说明" * @ApiParam(required = "是否必须参数", name ="参数名称", value ="参数具体描述" */ @ApiOperation(value = "账户信息查询接口") @RequestMapping(method ={RequestMethod.POST,RequestMethod.GET}, value="/query/dailydata/{dataDate}") @ResponseBody public DailyOperationDataDto getDailyReportByDataDate(@PathVariable("dataDate") String dataDate) { try { return DailyOperationDataService.getDailyReportByDataDate(dataDate); } catch (Exception e) { logger.error(e.getMessage(), e); } return null; } }
常用的一些注解
@Api:用在类上,说明该类的作用 @ApiOperation:用在方法上,说明方法的作用 @ApiImplicitParams:用在方法上包含一组参数说明 @ApiImplicitParam:用在 @ApiImplicitParams 注解中,指定一个请求参数的各个方面 paramType:参数放在哪个地方 · header --> 请求参数的获取:@RequestHeader · query -->请求参数的获取:@RequestParam · path(用于restful接口)--> 请求参数的获取:@PathVariable · body(不常用) · form(不常用) name:参数名 dataType:参数类型 required:参数是否必须传 value:参数的意思 defaultValue:参数的默认值 @ApiResponses:用于表示一组响应 @ApiResponse:用在@ApiResponses中,一般用于表达一个错误的响应信息 code:数字,例如400 message:信息,例如"请求参数没填好" response:抛出异常的类 @ApiParam:单个参数描述 @ApiModel:描述一个Model的信息,用对象来接收参数(这种一般用在post创建的时候,使用@RequestBody这样的场景,请求参数无法使用@ApiImplicitParam注解进行描述的时候) @ApiModelProperty:描述一个model的属性 @ApiProperty:用对象接收参数时,描述对象的一个字段 @ApiIgnore:使用该注解忽略这个API
使用springboot整合swagger2
maven依赖
<!--springfox依赖 --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.7.0</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.7.0</version> </dependency>
添加静态资源配置
@Configuration public class WebMvcConfig extends WebMvcConfigurerAdapter {/** * 配置静态资源路径以及上传文件的路径 * * @param registry */ @Override public void addResourceHandlers(ResourceHandlerRegistry registry) { registry.addResourceHandler("/static/**").addResourceLocations("classpath:/static/"); registry.addResourceHandler("/upload/**").addResourceLocations(environment.getProperty("spring.resources.static-locations")); /*swagger-ui*/ registry.addResourceHandler("swagger-ui.html").addResourceLocations("classpath:/META-INF/resources/"); registry.addResourceHandler("/webjars/**").addResourceLocations("classpath:/META-INF/resources/webjars/"); } }
swagger2的配置类
@Configuration @EnableSwagger2 public class SwaggerConfig { @Autowired private BaseConfig baseConfig; @Bean public Docket createRestApi() { if(Constant.TRUE.equals(baseConfig.getSwaggerShow())) { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() .apis(RequestHandlerSelectors.basePackage("com.cpic.sxwx")) .paths(PathSelectors.any()) .build(); }else { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() .paths(PathSelectors.none()) .build(); } } private ApiInfo apiInfo() { return new ApiInfoBuilder() .title("springboot利用swagger构建api文档") .description("1.返回结果如下:<br>{\"errorCode\":0,\"returnObject\":{\"name\":\"xxx\",\"mobile\":\"18812345678\",\"email\":\"[email protected]\"},\"message\":null}<br>" + "errorCode:0表示没有错误一切正常,1001服务器错误, 1002业务逻辑错误,1003自定义错误<br>" + "returnObject:返回需要用到的对象数据都会在这里面。上面这个例子是一个用户对象<br>" + "message:如果发生错误如业务逻辑错误,这里面就是错误的信息,格式为00000=验证码错误<br>"+ "2.http状态码描述:<br>" + "200:正常<br>" + "500:服务器错误<br>" + "400:参数验证不通过<br>" + "404:无法找到相关服务连接<br>" + "401:jwt不合法<br>") .termsOfServiceUrl("") .version("1.0") .build(); } }
接口的配置
/** * 前台文章Controller * @author 小卖铺的老爷爷 * @date 2018年5月5日 * @website www.laoyeye.net */ @Api(description="文章查询") @Controller @RequestMapping("/article") public class ArticleController { @Autowired private ArticleService articleService; @Autowired private SettingService settingService; @Autowired private CateService cateService; @Autowired private TagReferService tagReferService; @Autowired private UserService userService; @Autowired private ArticleMapper articleMapper; @Autowired private CommentService commentService; @ApiOperation(value="文章查询接口") @ApiImplicitParam(name = "id", value = "文章ID", required = true, dataType = "Long") @GetMapping("/{id}") public String index(Model model, @PathVariable("id") Long id) { try { articleService.updateViewsById(id); } catch (Exception ignore) { } List<Setting> settings = settingService.listAll(); Map<String,Object> map = new HashMap<String,Object>(); for (Setting setting : settings) { map.put(setting.getCode(), setting.getValue()); } Article article = articleService.getArticleById(id); model.addAttribute("settings", map); model.addAttribute("cateList", cateService.listAllCate()); model.addAttribute("article", article); model.addAttribute("tags", tagReferService.listNameByArticleId(article.getId())); model.addAttribute("author", userService.getNicknameById(article.getAuthorId())); //回头改 model.addAttribute("articles", articleMapper.listArticleByTitle(null)); model.addAttribute("similars", articleMapper.listArticleByTitle(null)); CommentQuery query = new CommentQuery(); query.setLimit(10); query.setPage(1); query.setArticleId(id); model.addAttribute("comments", commentService.listCommentByArticleId(query)); return "frontend/article"; } @ApiOperation(value="文章评论查询接口") @PostMapping("/comments") @ResponseBody public DataGridResult comments(CommentQuery query) { //设置默认10 query.setLimit(10); return commentService.listCommentByArticleId(query); } @ApiOperation(value="文章点赞接口") @ApiImplicitParam(name = "articleId", value = "文章ID", required = true, dataType = "Long") @PostMapping("/approve") @ResponseBody public YYBlogResult approve(@RequestParam Long articleId) { return articleService.updateApproveCntById(articleId); } }
如果你的项目在根目录:http://localhost:8080/swagger-ui.html
如果不是根目录那就是:http://localhost:8080/你的项目名/swagger-ui.html
扫描二维码关注公众号,回复:
8155871 查看本文章
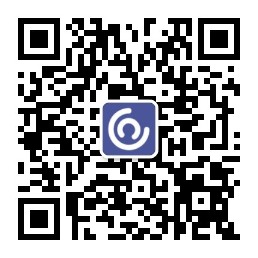