本文系笔者在做课程设计时完成的作品,发布出来主要还是想和大家一起分享交流以下学习成果。如果有什么疏漏的地方,还请各位业界大佬轻喷勿怪
废话不多话,首先上源码
这篇博客只是简单地介绍一下这个项目的内容,具体情况还请查看源代码自行阅读(其实就是我懒得打太多字)
1. 项目结构
View - Model - Dao 三层结构
View 层:使用 Swing 组件完成软件外观设计
Model层:提供实现业务需求的封装方法
Dao 层:提供与数据库操作相关的封装方法
2. 数据库建表语句
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- users 表
-- ----------------------------
DROP TABLE IF EXISTS `users`;
CREATE TABLE `users` (
`username` varchar(20) NOT NULL,
`password` varchar(20) NOT NULL,
PRIMARY KEY (`username`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of users
-- ----------------------------
INSERT INTO `users` VALUES ('root', '111');
INSERT INTO `users` VALUES ('root1', '1234');
INSERT INTO `users` VALUES ('root10', '123456');
INSERT INTO `users` VALUES ('root100', '123456');
INSERT INTO `users` VALUES ('root2', '1234');
INSERT INTO `users` VALUES ('root22', '222123');
INSERT INTO `users` VALUES ('root3', '1234');
INSERT INTO `users` VALUES ('root4', '1234');
INSERT INTO `users` VALUES ('root5', '1234');
INSERT INTO `users` VALUES ('root6', '6666');
INSERT INTO `users` VALUES ('root7', '1234');
INSERT INTO `users` VALUES ('root9', '123456');
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- students 学生表
-- ----------------------------
DROP TABLE IF EXISTS `students`;
CREATE TABLE `students` (
`sno` varchar(255) NOT NULL DEFAULT '0',
`name` varchar(255) DEFAULT NULL,
`sex` varchar(255) DEFAULT NULL,
`birthDate` varchar(255) DEFAULT NULL,
`department` varchar(255) DEFAULT NULL,
`major` varchar(255) DEFAULT NULL,
PRIMARY KEY (`sno`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of students
-- ----------------------------
INSERT INTO `students` VALUES ('0987', '5555', '女', '1994-09-04', '管理学院', '会计');
INSERT INTO `students` VALUES ('2099', 'ooll', '女', '1998-01-01', '信息科学与技术学院', '物联网');
INSERT INTO `students` VALUES ('3131', 'ffawa', '女', '1996-06-07', '管理学院', '工商管理');
INSERT INTO `students` VALUES ('4114', 'grsg', '男', '1990-01-01', '轻工食品学院', '食品安全');
INSERT INTO `students` VALUES ('4119', 'sgs', '男', '1996-06-02', '经贸学院', '贸易');
INSERT INTO `students` VALUES ('4144', 'faa', '男', '1997-04-01', '农学院', '植物保护');
INSERT INTO `students` VALUES ('4242', 'yyy', '男', '1996-01-01', '环境科学与工程学院 ', '环境科学');
INSERT INTO `students` VALUES ('4343', 'tea', '女', '2000-08-06', '信息科学与技术学院', '物联网');
INSERT INTO `students` VALUES ('7654', 'ttt', '男', '2000-05-06', '生命科学学院', '生命科学');
INSERT INTO `students` VALUES ('9090', 'viviv', '男', '1999-02-28', '轻工食品学院', '食品安全');
INSERT INTO `students` VALUES ('9922', 'erw', '男', '1996-01-01', '信息科学与技术学院', '物联网');
3. 实体类
package cn.stuManagementSys.entity;
//User 用户类
public class User {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public User(String username, String password) {
super();
this.username = username;
this.password = password;
}
public User() {
super();
}
}
package cn.stuManagementSys.entity;
//Student 学生类
public class Student {
private String sno;
private String name;
private String sex;
private String birthDate;
private String department;
private String major;
public String getSno() {
return sno;
}
public void setSno(String sno) {
this.sno = sno;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getBirthDate() {
return birthDate;
}
public void setBirthDate(String birthDate) {
this.birthDate = birthDate;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
public String getMajor() {
return major;
}
public void setMajor(String major) {
this.major = major;
}
public Student(String sno, String name, String sex, String birthDate, String department, String major) {
super();
this.sno = sno;
this.name = name;
this.sex = sex;
this.birthDate = birthDate;
this.department = department;
this.major = major;
}
public Student() {
super();
}
}
4. 工具类
package cn.stuManagementSys.utils;
import java.sql.Connection;
import java.sql.DriverManager;
//对 Jdbc 进行封装
public class JdbcUtil {
//此处填入自己本机数据库的信息
private static final String DBDRIVER = "com.mysql.cj.jdbc.Driver";
private static final String DBURL = "jdbc:mysql://localhost:3306/stumanagerdba?serverTimezone=UTC";
private static final String DBUSER = "root";
private static final String DBPASS = "123";
public static Connection getConnection() {
Connection conn = null;
try {
Class.forName(DBDRIVER);
conn = DriverManager.getConnection(DBURL, DBUSER, DBPASS);
return conn;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
5. Dao 层
package cn.stuManagementSys.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import cn.stuManagementSys.entity.User;
import cn.stuManagementSys.utils.JdbcUtil;
public class UserDao {
private Connection con = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
/**
* 根据用户名查找用户
* @return
* @throws SQLException
*/
public User searchUserByUsername(String username) {
String sql = "SELECT * FROM users WHERE username=?";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, username);
rs = pstmt.executeQuery();
if (rs.next()) {
return new User(rs.getString(1), rs.getString(2));
}
} catch (Exception e) {
throw new RuntimeException();
} finally {
try {
rs.close();
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException();
}
}
return null;
}
/**
* 新增用户
* @param user
*/
public void createUser(User user) {
String sql = "INSERT INTO users VALUES(?,?)";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException();
} finally {
try {
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException();
}
}
}
/**
* 删除指定用户
* @param username
*/
public void deleteUserByUsername(String username) {
String sql = "DELETE FROM users WHERE username=?";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, username);
pstmt.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException();
} finally {
try {
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException();
}
}
}
/**
* 修改密码
* @param newPw
* @param username
*/
public void updatePwByUsername(String newPw, String username) {
String sql = "UPDATE users SET password=? WHERE username=?";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, newPw);
pstmt.setString(2, username);
pstmt.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException();
} finally {
try {
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException();
}
}
}
}
package cn.stuManagementSys.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import cn.stuManagementSys.entity.Student;
import cn.stuManagementSys.utils.JdbcUtil;
public class StudentDao {
private Connection con = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
/**
* 根据条件返回符合条件的学生信息
*
* @param student
* @return
*/
public List<Student> queryStuListByFilter(Student student) {
List<Student> stuList = new ArrayList<Student>();
String sql = "SELECT * FROM students WHERE 1<2";
try {
con = JdbcUtil.getConnection();
if (student.getSno() != null && !student.getSno().equals(""))
sql += " AND sno=?";
if (student.getName() != null && !student.getName().equals(""))
sql += " and name=?";
if (student.getSex() != null && !student.getSex().equals(""))
sql += " and sex=?";
if (student.getBirthDate() != null && !student.getBirthDate().equals(""))
sql += " and birthDate=?";
if (student.getDepartment() != null && !student.getDepartment().equals(""))
sql += " and department=?";
if (student.getMajor() != null && !student.getMajor().equals(""))
sql += " and major=?";
pstmt = con.prepareStatement(sql);
int index = 0;
if (student.getSno() != null && !student.getSno().equals(""))
pstmt.setString(++index, student.getSno());
if (student.getName() != null && !student.getName().equals(""))
pstmt.setString(++index, student.getName());
if (student.getSex() != null && !student.getSex().equals(""))
pstmt.setString(++index, student.getSex());
if (student.getBirthDate() != null && !student.getBirthDate().equals(""))
pstmt.setString(++index, student.getBirthDate());
if (student.getDepartment() != null && !student.getDepartment().equals(""))
pstmt.setString(++index, student.getDepartment());
if (student.getMajor() != null && !student.getMajor().equals(""))
pstmt.setString(++index, student.getMajor());
rs = pstmt.executeQuery();
while (rs.next()) {
Student stu = new Student();
stu.setSno(rs.getString(1));
stu.setName(rs.getString(2));
stu.setSex(rs.getString(3));
stu.setBirthDate(rs.getString(4));
stu.setDepartment(rs.getString(5));
stu.setMajor(rs.getString(6));
stuList.add(stu);
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
con.close();
rs.close();
pstmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
return stuList;
}
/**
* 查询全部学生信息
*
* @return
*/
public List<Student> queryAllStudents() {
List<Student> stuList = new ArrayList<Student>();
String sql = "SELECT * FROM students";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
rs = pstmt.executeQuery();
while (rs.next()) {
stuList.add(new Student(rs.getString(1), rs.getString(2), rs.getString(3), rs.getString(4),
rs.getString(5), rs.getString(6)));
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
rs.close();
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
return stuList;
}
/**
* 根据学号删除学生信息
*
* @param sno
*/
public void deleteStudentBySno(String sno) {
String sql = "DELETE FROM students WHERE sno=?";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, sno);
pstmt.executeUpdate();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
con.close();
pstmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
/**
* 根据学号查询学生信息
*
* @param sno
* @return
*/
public Student queryStudentBySno(String sno) {
String sql = "SELECT * FROM students WHERE sno=?";
Student stu = null;
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, sno);
rs = pstmt.executeQuery();
while (rs.next()) {
stu = new Student();
stu.setSno(rs.getString(1));
stu.setName(rs.getString(2));
stu.setSex(rs.getString(3));
stu.setBirthDate(rs.getString(4));
stu.setDepartment(rs.getString(5));
stu.setMajor(rs.getString(6));
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
con.close();
pstmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
return stu;
}
/**
* 插入学生信息
*
* @param student
*/
public void insertStudent(Student student) {
String sql = "INSERT INTO students VALUES(?,?,?,?,?,?)";
try {
con = JdbcUtil.getConnection();
String sno = student.getSno();
String sname = student.getName();
String ssex = student.getSex();
String sbirthday = student.getBirthDate();
String stie = student.getDepartment();
String smajor = student.getMajor();
pstmt = con.prepareStatement(sql);
pstmt.setString(1, sno);
pstmt.setString(2, sname);
pstmt.setString(3, ssex);
pstmt.setString(4, sbirthday);
pstmt.setString(5, stie);
pstmt.setString(6, smajor);
pstmt.executeUpdate();
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
try {
con.close();
pstmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
/**
* 删除查询出来的全部学生信息
*
* @param stuList
*/
public void deleteStudentsByList(List<Student> stuList) {
String sql = "DELETE FROM students WHERE sno=?";
try {
con = JdbcUtil.getConnection();
pstmt = con.prepareStatement(sql);
for (int i = 0; i < stuList.size(); i++) {
pstmt.setString(1, stuList.get(i).getSno());
pstmt.executeUpdate();
}
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
try {
con.close();
pstmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
/**
* 修改学生信息
*
* @param student
*/
public void updateStudent(Student student, String oldSno) {
try {
con = JdbcUtil.getConnection();
String sql = "UPDATE students SET sno=?, name=?, sex=?, birthDate=?, department=?, major=? where sno=?";
pstmt = con.prepareStatement(sql);
pstmt.setString(1, student.getSno());
pstmt.setString(2, student.getName());
pstmt.setString(3, student.getSex());
pstmt.setString(4, student.getBirthDate());
pstmt.setString(5, student.getDepartment());
pstmt.setString(6, student.getMajor());
pstmt.setString(7, oldSno);
pstmt.executeUpdate();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
try {
pstmt.close();
con.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
}
6. Model 层
package cn.stuManagementSys.service;
import cn.stuManagementSys.dao.UserDao;
import cn.stuManagementSys.entity.User;
import cn.stuManagementSys.exception.UserException;
public class UserService {
private UserDao userDao = new UserDao();
/**
* 登录
* @throws UserException
*/
public void Login(String username, String password) throws UserException {
User user = userDao.searchUserByUsername(username);
if(user == null) {
throw new UserException("用户不存在");
} else if(!(user.getPassword().equals(password))) {
throw new UserException("密码错误");
}
}
/**
* 修改密码
* @param username
* @param oldPw
* @param newPw
* @throws UserException
*/
public void UpdatePw(String username, String oldPw, String newPw) throws UserException {
User user = userDao.searchUserByUsername(username);
if(user == null) {
throw new UserException("用户不存在");
} else if(!(user.getPassword().equals(oldPw))) {
throw new UserException("密码错误");
} else {
userDao.updatePwByUsername(newPw, username);
}
}
/**
* 按用户名查询用户
* @param username
* @return
*/
public User searchUserByUsername(String username) {
return userDao.searchUserByUsername(username);
}
/**
* 新增用户
* @param user
*/
public void createUser(User user) {
userDao.createUser(user);
}
}
package cn.stuManagementSys.service;
import java.util.List;
import cn.stuManagementSys.dao.StudentDao;
import cn.stuManagementSys.entity.Student;
public class StudentService {
private StudentDao studentDao = new StudentDao();
/**
* 根据条件返回符合条件的学生二维数组
* @param student
* @return
*/
public List<Student> queryStuListByFilter(Student student) {
return studentDao.queryStuListByFilter(student);
}
/**
* 查询全部学生的信息
* @param student
* @return
*/
public List<Student> queryAllStudent() {
return studentDao.queryAllStudents();
}
/**
* 根据学号删除学生信息
* @param sno
*/
public void deleteStudentBySno(String sno) {
studentDao.deleteStudentBySno(sno);
}
/**
* 根据学号查询相应学生信息
* @param sno
* @return
*/
public Student queryStudentBySno(String sno) {
return studentDao.queryStudentBySno(sno);
}
/**
* 插入学生信息
* @param student
*/
public void insertStudent(Student student) {
studentDao.insertStudent(student);
}
/**
* 删除查询出来的全部学生信息
* @param stuList
*/
public void deleteStudentsByList(List<Student> stuList) {
studentDao.deleteStudentsByList(stuList);
}
/**
* 修改学生信息
* @param student
*/
public void updateStudent(Student student, String oldSno) {
studentDao.updateStudent(student, oldSno);
}
}
7. View 层(仅展示部分效果图)
扫描二维码关注公众号,回复:
8769023 查看本文章
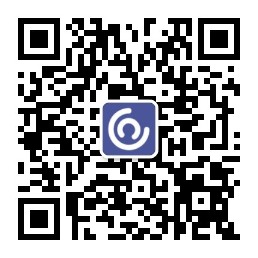
- 登录效果图
- 首页图
- 添加学生信息
- 查询效果图
- 注册账户
- 密码修改
- 修改学生信息