题目描述如下:
Given two binary trees, write a function to check if they are the same or not.
Two binary trees are considered the same if they are structurally identical and the nodes have the same value.
Example 1:
Input: 1 1
/ \ / \
2 3 2 3
[1,2,3], [1,2,3]
Output: true
Example 2:
Input: 1 1
/ \
2 2
[1,2], [1,null,2]
Output: false
Example 3:
Input: 1 1
/ \ / \
2 1 1 2
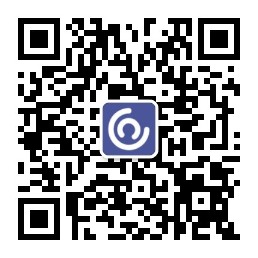
[1,2,1], [1,1,2]
Output: false
个人思路:
该题目肯定是需要用到二叉树的遍历相关知识。当然,最简单的就是层序遍历了。本题目只需要对层序遍历算法稍加修改即可。在实现层序遍历的时候,我们一般借助队列来完成。
首先是根节点入队,然后开始循环遍历,取出队头元素,判断两个树的该节点的值val是否相等,如果不等直接返回false。如果相等的话则把该结点出队。
然后判断两棵树的该结点是否还有左子树,如果都有的话则左子树节点入队;如果是一个有一个没有,则两颗二叉树在结构上已经相异,此时返回false;如果都没有左子树,则转去判断右子树。
判断右子树的情况与左子树相似,就不再赘述。
判断完成后即可进入下一轮循环,直到返回了false或者队列为空时循环结束并返回true。
代码:
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
bool isSameTree(TreeNode* p, TreeNode* q) {
if(!p && !q) return true; //处理特殊情况,两棵树皆空
if(!p || !q) return false; //处理特殊情况,一个树空一个非空
queue<TreeNode*> p1;
queue<TreeNode*> q1;
p1.push(p);
q1.push(q);
while((!q1.empty()) && (!p1.empty())){ //层序遍历循环
TreeNode* tempP = p1.front(); //取队头
TreeNode* tempQ = q1.front(); //取队头
if(tempP->val != tempQ->val) { //判断值相等
return false;
}
else{ //当前判断的结点出队
p1.pop();
q1.pop();
}
if(tempP->left && tempQ->left){//判断有无左子树,两个都有的话入队
p1.push(tempP->left);
q1.push(tempQ->left);
}
else if(tempP->left != tempQ->left)return false; //两个都没有的话则依旧是结构相等,否则结构不相等返回false
if(tempP->right && tempQ->right){//判断有无右子树,两个都有的话入队
p1.push(tempP->right);
q1.push(tempQ->right);
}
else if(tempP->right != tempQ->right)return false;//两个都没有的话则依旧是结构相等,否则结构不相等返回false
}
return true;
}