1.引用
&
#include <iostream>
using namespace std;
int main(int argc, const char * argv[]) {
int n = 7;
int & r = n;
r = 4;
cout<<n<<endl; //4
n = 5;
cout<<r<<endl; //5
return 0;
}
必须初始化为一个变量(与这个变量是一回事,“等价”),并且从一而终,不能引用别的变量
c语言 交换两个数 指针 调用swap函数
void swap(int *a,int *b){
int t;
t = *a;
*a = *b;
*b = t;
}
//调用的时候 swap(&x1,&x2);
c++
void swap(int &a,int &b){
int t;
t = a;
a = b;
b = t;
}
//调用的时候不用取地址
常引用
const int & r = n
不能通过常引用r 去修改其引用的内容,可以修改n
注意
eg
int & 可以初始化 const int &
但是const int & 不能用来初始化 int & ,除非进行强制类型转换。
2.const
1)定义常量
const int max = 23;
const char *c = “jhgfds”;
2)定义常量指针——不可以通过常量指针修改其指向内容,常量指针指向可以变化
常量指针赋值给非常量 不可以,除非强制类型转换
(假如赋值给了一个变量,可以通过 *来修改:不安全)
非常量赋值给常量 可以
标准模板库
3.string类
初始化
string s1("hello");
string s2(8,'x'); //八个x
string s3 = "fignting";
注意:
string s3 = ‘x’; 是错的
正确:
string s3;
s3 = ‘x’;
长度
s1.length()
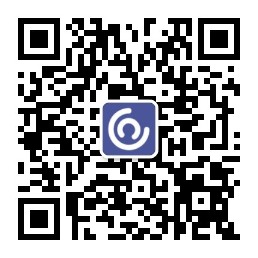
读入
支持流读取运算符: cin >> s1;
支持getline函数 : getline(cin,s1);:
赋值
用=赋值: s2 = s1;
assign 复制: s2.assign(s1);
assign 部分复制: s2.assign(s1,5,10);
单个字符: s2[1] = s1[3];
遍历
cout << s1.at(i) << endl;
[]不做范围检查 at会做范围检查
连接
s2连接到s1后面,改变s1长度 :
s1 += s2;
s1.append(s2);
append 连接部分:
string s1("good"),s2("moring");
s1.append(s2,1,2);
cout << s1 << endl;//goodr
删除插入修改
erase
string s1("012345kiki");
cout << s1.erase(5) << endl; //01234 去掉5以及5以后的字符
replace
string s1("kikikiki");
cout << s1.replace(4,4,"coco") << endl; //kikicoco
insert
string s1("0123456789"),s2("this");
cout << s1.insert(4,s2) << endl;
// 0123this456789
cout << s1.insert(4,"here") << endl;
//0123herethis456789
cout << s1.insert(1,s2,2,3) << endl;
//s1 从1开始插入s2 从2开始的3个字符
// 0is123herethis456789
比较
直接 > < ==
string s1("good"),s2("moring");
bool t = (s1==s2);
cout << t << endl; //0
t = (s1<s2);
cout << t << endl; //1
t = (s1>s2);
cout << t << endl; //0
compare (更强大,比较一部分)
string s1("good"),s2("god");
int t = s1.compare(s2);
cout << t << endl; //11
t = s1.compare(1,2,s2,0,2); //s1 : 1-2 s2 : 0-2
cout << t << endl; // 8
子串
subsets
string s1("goodmoring"),s2;
s2 = s1.substr(0,4); //从0开始4个
cout << s2 << endl; //good
swap
string s1("kiki"),s2("coco");
cout << s1 << s2 << endl; //kikicoco
s1.swap(s2);
cout << s1 << s2 << endl; //cocokiki
查找
find 从前往后找
rfind 从后往前找
string s1("012345kiki");
cout << s1.find("ki") << endl;
//6 第一次出现的下标
cout << s1.find("coco") << endl;
//一个h常数。string静态常量18446744073709551615
cout << s1.rfind("ki") << endl;
//8 第一次出现的下标
find 指定从第几个开始查找
string s1("012345kiki");
cout << s1.find("ki",7) << endl;
//从第七个开始查找(包含第七个)
//8 第一次出现的下标
find_first_of :查找字符
mmmk中任意一个字符第一次出现的位置
string s1("012345kiki");
cout << s1.find_first_of("mmmk") << endl; //6 第一次出现的下标
mmmk中任意一个字符最后一次出现的位置
string s1("012345kiki");
cout << s1.find_last_of("mmmmmk") << endl; //8 最后一次出现的下标
find_first_not_of
find_last_not_of
string s1("012345kiki");
cout << s1.find_first_not_of("mmmmmk") << endl; //0
cout << s1.find_last_not_of("mmmmmk") << endl; //9
转换成c
string s1("0123456789");
printf("%s\n",s1.c_str());
//返回传统的conse char *类型字符串 并且以\0结尾
//用处:c语言的有些库函数需要const char * 但是c++里是string
string s1("0123456789");
const char *p = s1.data();
for(int i=0; i<s1.length(); i++)
printf("%c",*(p+i)); //0123456789
字符串流处理
include
#include
#include
用 istringstream ostringstream
从字符串里面输入数据
mooc: