POM文件添加
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
添加Redis Key生成策略类
package com.test;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.interceptor.KeyGenerator;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.data.redis.cache.RedisCacheManager;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* 通过注解使用Redis内存服务器
*/
@Configuration
@EnableCaching
public class RedisConfig extends CachingConfigurerSupport{
/**
* 生成Redis的键值,根据类名+方法名+参数值的形式
* @return
*/
@Bean(name = "keyGenerator")
public KeyGenerator keyGenerator() {
return new KeyGenerator(){
@Override
public Object generate(Object target, java.lang.reflect.Method method,
Object... params) {
StringBuffer sb = new StringBuffer();
sb.append(target.getClass().getName());
sb.append(method.getName());
for(Object obj:params){
if(obj != null)
{
sb.append(obj.toString());
}
}
return sb.toString();
}
};
}
/**
* 生成CacheManager对象
* @param redisTemplate
* @return
*/
@Bean
public CacheManager cacheManager(@SuppressWarnings("rawtypes") RedisTemplate redisTemplate) {
RedisCacheManager cacheManager = new RedisCacheManager(redisTemplate);
cacheManager.setDefaultExpiration(10000);
return cacheManager;
}
/**
* 生成Redis内存管理模板,注解@Primary代表此对象是同类型对象的主对象,优先被使用
* @param factory
* @return
*/
@Primary
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory){
RedisTemplate<String, Object> template = new RedisTemplate<String, Object>();
template.setKeySerializer(new StringRedisSerializer());
template.setConnectionFactory(factory);
setSerializer(template);
template.afterPropertiesSet();
return template;
}
/**
* 序列化Redis的键和值对象
* @param template
*/
private void setSerializer(RedisTemplate template){
@SuppressWarnings({ "rawtypes", "unchecked" })
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer =
new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setValueSerializer(jackson2JsonRedisSerializer);
}
}
Spring提供的注解
Spring为我们提供了几个注解来支持Spring Cache。其核心主要是@Cacheable、@CachePut和@CacheEvict。
使用@Cacheable标记的方法,如果未缓存,在执行后Spring Cache将缓存其返回结果;已缓存,则直接返回缓存内容;
使用@CachePut标记的必然会在方法执行后,更新缓存;
而使用@CacheEvict标记的方法会在方法执行前或者执行后移除Spring Cache中的某些元素。
@Cacheable可以标记在一个方法上,也可以标记在一个类上。当标记在一个方法上时表示该方法是支持缓存的,当标记在一个类上时则表示该类所有的方法都是支持缓存的。对于一个支持缓存的方法,Spring会在其被调用后将其返回值缓存起来,以保证下次利用同样的参数来执行该方法时可以直接从缓存中获取结果,而不需要再次执行该方法。
@CachePut
如果缓存需要更新,且不干扰方法的执行,可以使用注解@CachePut。@CachePut标注的方法在执行前不会去检查缓存中是否存在之前执行过的结果,而是每次都会执行该方法,并将执行结果以键值对的形式存入指定的缓存中。
@CacheEvict
spring cache不仅支持将数据缓存,还支持将缓存数据删除。此过程经常用于从缓存中清除过期或未使用的数据。
@CacheEvict要求指定一个或多个缓存,使之都受影响。此外,还提供了一个额外的参数allEntries 。表示是否需要清除缓存中的所有元素。默认为false,表示不需要。当指定了allEntries为true时,Spring Cache将忽略指定的key。有的时候我们需要Cache一下清除所有的元素。
CacheConfig
有时候一个类中可能会有多个缓存操作,而这些缓存操作可能是重复的。这个时候可以使用@CacheConfig
开启缓存注解
@EnableCaching
Controller例程
package com.test.ctrl;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheConfig;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Controller;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.fasterxml.jackson.databind.util.JSONPObject;
import com.github.pagehelper.PageInfo;
import com.test.model.AddressInfo;
import com.test.model.StudentInfo;
import com.test.service.IAddressService;
@Controller
@CacheConfig(cacheNames="student")
public class StudCtrl {
@Autowired
private IAddressService addsrv;
@RequestMapping("/studlist")
public String studlist()
{
return "studlist";
}
@RequestMapping("/getprovice")
@ResponseBody
public List<AddressInfo> getprovice()
{
// List<Map> list = new ArrayList<Map>();
// Map m1 = new HashMap();
// m1.put("code","001");
// m1.put("name", "北京");
// list.add(m1);
// Map m2 = new HashMap();
// m2.put("code","002");
// m2.put("name", "上海");
// list.add(m2);
// return list;
return addsrv.getProvince();
}
@RequestMapping("/getcity")
@ResponseBody
public List<AddressInfo> getcity(String code)
{
return addsrv.getCity(code);
}
@RequestMapping("/getcounty")
@ResponseBody
public List<AddressInfo> getcounty(String code)
{
return addsrv.getCounty(code);
}
@RequestMapping("/savestudent")
@ResponseBody
@CacheEvict
public Boolean savestudent(@RequestBody StudentInfo si) throws Exception
{
// InputStream is = req.getInputStream();
// byte[] content = FileCopyUtils.copyToByteArray(is);
// System.out.println(new String(content));
// JSONObject jsonObj = JSONObject.parseObject(new String(content));
// StudentInfo si = (StudentInfo)JSONObject.toJavaObject(jsonObj,StudentInfo.class);
System.out.println(si);
if(si.getId() != null)
addsrv.updateStudent(si);
else {
addsrv.saveStudent(si);
}
return true;
}
@RequestMapping("/findstudbyid")
@ResponseBody
public StudentInfo findstudbyid(Integer id) throws Exception
{
return addsrv.findStudentById(id);
}
@RequestMapping("/findstud")
@ResponseBody
@Cacheable
public PageInfo findstud(Integer page,Integer rows,@RequestBody Map map) throws Exception
{
String name = null;
String age1 = null;
String age2 = null;
if(map != null)
{
name = (String)map.get("name");
age1 = (String)map.get("age1");
age2 = (String)map.get("age2");
}
return addsrv.findStudent(page, rows, name,age1,age2);
}
@RequestMapping("/deletestud")
@ResponseBody
@CacheEvict
public Boolean deletestud(String ids)
{
if(ids != null)
{
String[] dim = ids.split(",");
for(String s:dim)
{
addsrv.deleteStudent(new Integer(s));
}
}
return true;
}
}
代码下载
https://pan.baidu.com/s/1TrfQi6QOkEoZRCPw398FBQ 提取码g6v2
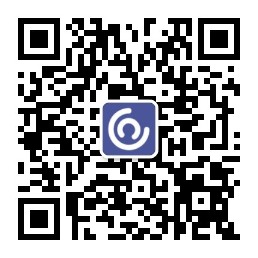