通过JDK8源码javadoc,可以知道@FunctionalInterface有以下特点:
- 该注解只能标记在"有且仅有一个抽象方法"的接口上,表示函数式接口。
- JDK8接口中的静态方法和默认方法,都不算是抽象方法。
- 接口默认继承java.lang.Object,所以如果接口显示声明覆盖了Object中的方法,那么也不算抽象方法。
- 该注解不是必须的,如果一个接口符合"函数式编程"定义,那么加不加该注解都没有影响。加上该注解能够更好地让编译器进行检查,如果编写的不是函数式接口,但是加上了@FunctionalInterface 那么编译器会报错。
代码说明:使用Lambda表达式。一般的格式是 ()->{} ,如果{}里面只有一行代码,则{}可以省略。 (->左边的()表示方法体,如果有形参,则在()中添加形参,->右边{}表示具体逻辑。如果方法体返回值是void,则甚至可以在{}中不写任何逻辑(当然也要结合场景)。返回值如果有值,则需要写具体的逻辑,return处理后的值。)理解这里非常重要!!!!
演示一、请求参数、返回参数均有值的接口
package com.calvin.currency.function;
/**
* @Title CustomFuctionInterface
* @Description 自定义函数式接口之演示一、请求参数、返回参数均有值的接口
* @author calvin
* @date: 2020/3/3 1:02 AM
*/
@FunctionalInterface
public interface CustomFuctionInterface {
String printStr(String str1, String str2);
}
代码测试
@Test
public void test1() {
CustomFuctionInterface customFuctionInterface = (str1, str2) -> "hello " + str1 + str2;
String printStr = customFuctionInterface.printStr("A&", "B");
System.out.println("printStr = " + printStr);
}
控制台输出结果:
演示二、请求参数没有值、返回参数有值的接口
package com.calvin.currency.function;
/**
* @Title CustomFuctionInterface
* @Description 自定义函数式接口之演示二、请求参数没有值、返回参数有值的接口
* @author calvin
* @date: 2020/3/3 1:06 AM
*/
@FunctionalInterface
public interface CustomFuctionInterface2 {
String printStr();
}
代码测试
@Test
public void test2() {
CustomFuctionInterface2 customFuctionInterface2 = () -> "hello world";
String printStr = customFuctionInterface2.printStr();
System.out.println("printStr = " + printStr);
}
控制台输出结果:
演示三、实际项目中可借鉴使用(落地)
/**
* @Title CustomFuctionInterface
* @Description 自定义函数式接口之演示三、实际项目中可借鉴使用(落地)
* @author calvin
* @date: 2020/3/3 1:25 AM
*/
@FunctionalInterface
public interface CustomFuctionInterface3 {
void doSomething();
}
假设现在某个类的某个方法形参为CustomFuctionInterface3,如代码所示:
public static void execute(CustomFuctionInterface3 interface3) {
interface3.doSomething();
}
传统的调用方法 :
@Test
public void test3() {
execute(new CustomFuctionInterface3() {
@Override
public void doSomething() {
System.out.println("doSomething...");
}
});
}
控制台输出结果:
通过Lambda表达式改进以上测试代码:
扫描二维码关注公众号,回复:
9647257 查看本文章
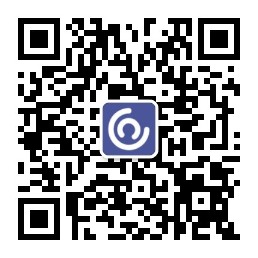
@Test
public void test3() {
execute(() -> System.out.println("doSomething..."));
}
再次查看控制台输出结果:
可以发现结果是一致的,代码看起来更加简洁美观。
总结: 以上几个小案例,通过使用函数式接口@FunctionalInterface+Lambda表达式进行了代码演示,对于我们理解函数式编程的思想以及并发工具包JUC下的函数型、断定型、消费者、供给型等接口的源码阅读都有一定的帮助。