前 言
人总是要有梦想的,也许哪天就实现了呢!
如果有不正确之处,还望指正,毕竟我还是一个菜鸟!
Servlet 登录案例
1、需求分析
用户输入自己的账号和密码,点击登录,账号和密码正确显示登录成功,不正确则显示登录失败。
目录结构:
2、数据库建表
3、前端页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户登录</title>
</head>
<body>
<form action="/login_war_exploded//login.dailycode" method="post">
<label>账号:</label>
<input type="text" name="username" /><br>
<label>密码:</label>
<input type="password" name="password" /><br>
<input type="submit" value="点击登录">
</form>
</body>
</html>
4、用户实体类
public class User {
private int id;
private String username;
private String password;
public User() {
}
public User(int id, String username, String password) {
this.id = id;
this.username = username;
this.password = password;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
'}';
}
}
5、DAO 层
public interface UserDao {
public User checkUser(String username, String password) throws SQLException;
}
public class UserDaoImpl implements UserDao {
private User user = new User();
@Override
public User checkUser(String username, String password) throws SQLException {
Connection conn = JdbcUtils.getConnection();
String sql = "select * from user where username = ? and password = ?";
PreparedStatement pst = conn.prepareStatement(sql);
pst.setString(1, username);
pst.setString(2, password);
ResultSet results = pst.executeQuery();
while (results.next()) {
user.setId(results.getInt("id"));
user.setUsername(results.getString("username"));
user.setPassword(results.getString("password"));
}
JdbcUtils.close(results, pst, conn);
return user;
}
}
6、Servlet
/**
* 登录Servlet
*/
@WebServlet(value = "/login.dailycode")
public class LoginServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
try {
req.setCharacterEncoding("UTF-8");
resp.setContentType("text/html;charset=utf-8");
UserDaoImpl userDao = new UserDaoImpl();
String username = req.getParameter("username");
String password = req.getParameter("password");
User user = userDao.checkUser(username, password);
if (user != null) {
req.setAttribute("username", username);
req.getRequestDispatcher("/main.do").forward(req, resp);
} else {
resp.getWriter().write("<h3 color='red'>您输入的用户或密码错误!</h3>");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doPost(req, resp);
}
}
/**
* 主页面servlet
*/
@WebServlet(value = "/main.do")
public class MainServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setContentType("text/html;charset=utf-8");
String username = (String) req.getAttribute("username");
resp.getWriter().write("<h3 color='red'>欢迎" + username + "</h3>");
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doPost(req, resp);
}
}
7、工具类
/**
* 数据库工具类,采用Druid连接池实现
*/
public class JdbcUtils {
private static DataSource ds;
/**
* 加载配置文件信息,初始化数据源
*/
static {
try {
Properties pro = new Properties();
InputStream in = JdbcUtils.class.getClassLoader()
.getResourceAsStream("druid.properties");
pro.load(in);
ds = DruidDataSourceFactory.createDataSource(pro);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 获取数据库连接
* @return
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
return ds.getConnection();
}
/**
* 获取数据源对象
* @return
*/
public static DataSource getDataSource() {
return ds;
}
/**
* 释放资源,用于增删改操作
* @param stmt
* @param conn
*/
public static void close(Statement stmt, Connection conn) {
if (stmt != null ){
try{
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null ){
try{
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
* 释放资源,用于查询操作
* @param rs
* @param stmt
* @param conn
*/
public static void close(ResultSet rs, Statement stmt, Connection conn) {
if (rs != null ){
try{
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stmt != null ){
try{
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null ){
try{
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
8、Druid 配置信息
扫描二维码关注公众号,回复:
9975103 查看本文章
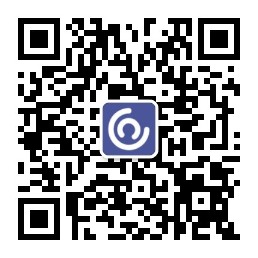
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/test?serverTimezone=Hongkong
username=root
password=root
initialSize=5
maxActive=10
maxWait=3000