A, JSR107
Java Caching defines five core interfaces are CachingProvider, CacheManager, Cache, Entry and Expiry.
1, CachingProvider defines create, configure, acquire, manage and control multiple CacheManager.
An application can access multiple CachingProvider at runtime.
2, CacheManager defines create, configure, acquire, manage and control multiple uniquely named Cache,
The Cache is present in the context of CacheManager. A CacheManager only a CachingProvider owned.
3, Cache Map is similar to a data structure and temporarily stored as the value of the index Key. Cache is a only a CacheManager owned.
4, Entry is a key-value pairs stored in the Cache.
5, Expiry stored in each of the Cache entry has a defined period. Once over this time, the entry for the expired state.
Once expired, the entry will not be accessible, update, and delete. Cache validity period can be set by ExpiryPolicy.
Use too much trouble
Two, Spring cache abstraction
Spring version 3.1 defines org.springframework.cache.Cache
And org.springframework.cache.CacheManager interfaces to unify different caching techniques;
And supports JCache (JSR-107) annotations simplify our development;
Cache interface specification is defined as the assembly cache, the cache comprising a set of various operations;
Cache interface at Spring provides an implementation of various xxxCache;
As RedisCache, EhCacheCache, ConcurrentMapCache the like;
Each time method requires the cache function calls the specified target method Spring will check to check whether the specified parameter has been called;
If there is to get the result of method calls directly from the cache, returned to the user if not call the method and caches the results.
Next call is available directly from the cache.
When using Spring cache abstraction that we need to focus on two things;
1, to determine the method needs to be cached as well as their cache policy
2, the cache memory before reading the data from the cache
Third, the basic build environment:
Code practice to explain:
1, new construction:
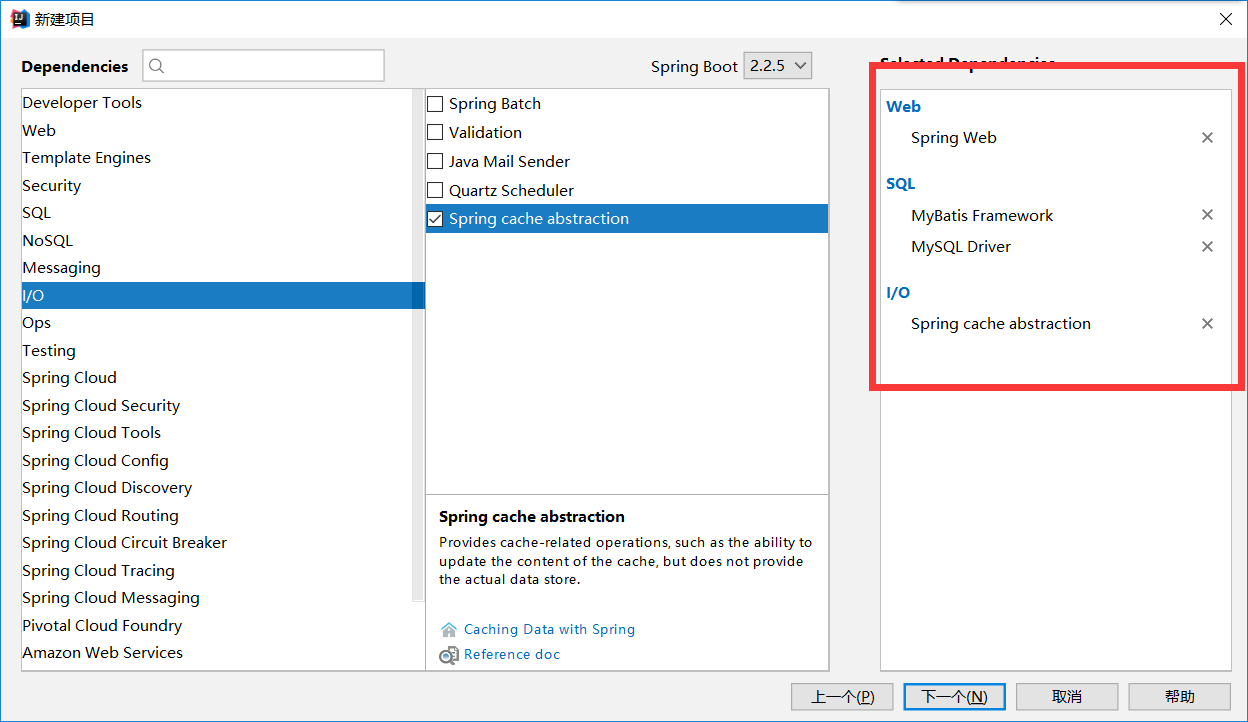
2, create a database table and the corresponding javabean
department table structure
employee table structure
public class Department { private Integer id; private String departmentName; public Department() { }
....
}
public class Employee { private Integer id; private String lastName; private String email; private Integer gender; private Integer dId; public Employee() { } ..... }
3, the operation of the database integration mybatis
Basic configuration data source information in the application.xml
spring.datasource.url=jdbc:mysql://localhost:3306/springboot_cache?serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# Enable camelCase
mybatis.configuration.map-underscore-to-camel-case=true
Add mapper interface corresponds to the entity classes
Being the first to write a test EmployeeMapper
// @ Mapper // configured @MapperScan springbooot start in class, so there will not need to configure the @Mapper
public interface EmployeeMapper {
@Select("select * from employee where id = #{id}")
Public Employee getEmpById (Integer id);
@Update("update employee set last_name=#{lastName},email=#{email},gender=#{gender},d_id=#{dId} where id=#{id}")
public void updateEmp(Employee employee);
@Delete("delete employee where id=#{id}")
public void deleteEmpById(Integer id);
@Insert("insert into employee(last_name,email,gender,d_id) values(#{lastName},#{email},#{gender},#{d_id})")
public void insertEmp(Employee employee);
}
In springbooot start a mapper class configuration under specified scanning @MapperScan which package, mapper interfaces will not need to configure the @Mapper
@MapperScan("com.atguigu.cache.mapper") @SpringBootApplication public class Springboot01CacheApplication { public static void main(String[] args) { SpringApplication.run(Springboot01CacheApplication.class, args); } }
Manually insert a record
Query test operation in the test class
@SpringBootTest class Springboot01CacheApplicationTests { @Autowired EmployeeMapper employeeMapper; @Test void contextLoads() { Employee emp = employeeMapper.getEmpById(1); System.out.println(emp); } }
Console output:
Description successful integration mybatis
4, create a service layer and controller layer test
@RestController public class EmployeeController { @Autowired EmployeeService employeeService; @RequestMapping("/emp/{id}") public Employee getEmployee(@PathVariable("id") Integer id){ Employee emp = employeeService.getEmp(id); return emp; } }
@Service public class EmployeeService { @Autowired EmployeeMapper employeeMapper; Public Employee getEmp (Integer id) { System.out.println ( "query" + id + "number of employees" ); Employee employee = employeeMapper.getEmpById(id); return employee; } }
At this visit in the browser:
Successful visit, the basic environment to build success.