Recently, my application needs to use cross-platform sharing links. It just happens that the AppLinking service of Huawei AppGallery Connect meets my usage scenarios.
Regarding the integration steps, there is a lot of information on the official website. I will summarize the steps
i. Step 1: Create an application and activate the AppLinking service
ii. Step 2: Create a link prefix
iii. Step 3: Integrate AppLinking SDK in the Android project;
iv. Step 4: Create AppLinking
v. Step 5: Receive AppLinking link and test.
1. Create an application and activate AppLinking service
In the AGC console, create an application, or use an existing application, find My Project -> Growth -> AppLinking on the interface, and click Activate Now.
After opening, remember to go to My Project -> Project Settings -> General, download the agconnect-services.json file to the app path of your Android project.
2. Create a link prefix
Under AppLinking that has just been activated, click the link prefix tab, click Add Link Prefix, and create a unique prefix on the live network as needed.
The system will automatically check for you to ensure that your domain name is unique in the entire network.
3. Integrate AppLinking SDK in the Android project
Configure the SDK address, open the Android project, and configure the following in the project-level build.gradle file
buildscript {
repositories {
//….
maven {url 'https://developer.huawei.com/repo/'}
}
dependencies {
//….
classpath 'com.huawei.agconnect:agcp:1.4.1.300'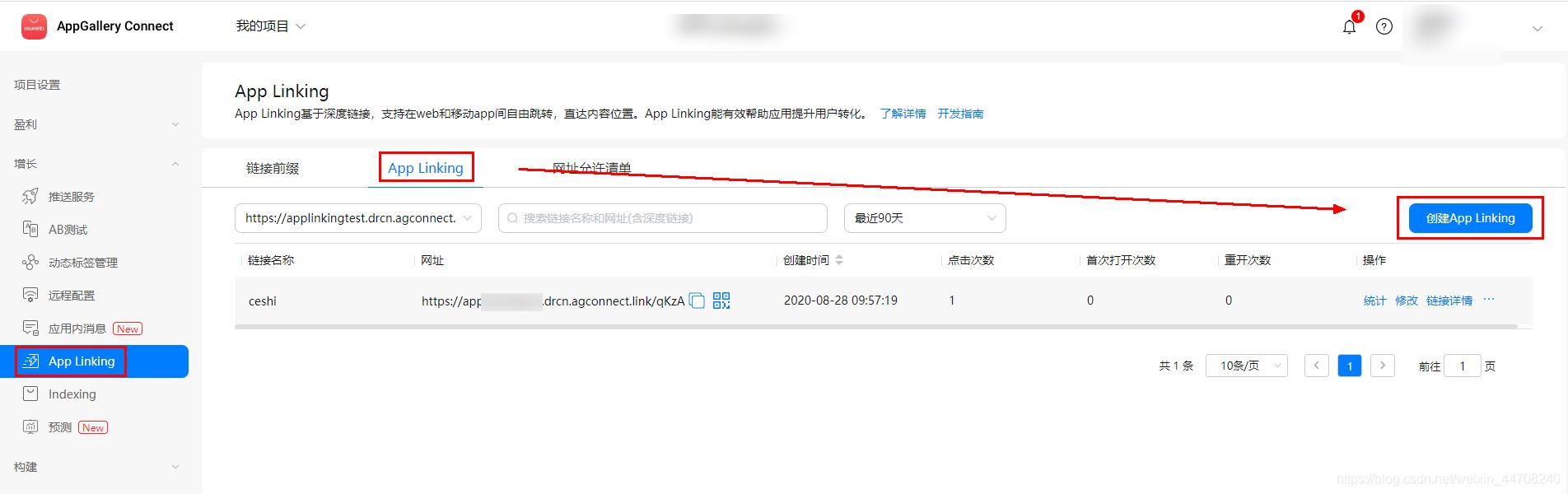
}
}
allprojects {
repositories {
//….
maven {url 'https://developer.huawei.com/repo/'}
}
}
Open the application-level build.gradle file, configure AppLinking and Huawei Analytics SDK, and configure the content marked in red below
…
apply plugin: 'com.huawei.agconnect'
...
dependencies {
...
implementation "com.huawei.agconnect:agconnect-applinking:1.4
implementation 'com.huawei.hms:hianalytics:5.0.4.301'.1.300"
}
4. Create AppLinking
There are two ways to create AppLinking: one is to create it directly on the AGC interface, and the other is to create it in the Android project with the API interface of the code.
4.1 Create AppLinking in AGC interface:
1. The interface entry is as follows: click Create AppLinking, and then create it step by step according to the steps.
2. With the default deep link configuration, I just found a Huawei official website at will. Pay attention to the configuration of Android's deep link.
3. Android link behavior, configured as: open in Android application.
After creating it, you can copy it and use it
4.2 End-side code creation AppLinking
1. First configure the interface in activity_main.xml: two buttons, one to create and one to share; then create a TextView display box to display AppLinking.
<Button
android:id="@+id/create"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Create App Linking"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.4" />
<TextView
android:id="@+id/showLink"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="your App Linking"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.5" />
<Button
android:id="@+id/shareLink"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Share App Linking"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.8" />
As shown below:
2. Then copy and add the link prefix just created to the constant, and first configure the DeepLink address that needs to be opened.
private static final String DOMAIN_URI_PREFIX = "https://testapplinking1016.drcn.agconnect.link";
private static final String DEEP_LINK = " https://consumer.huawei.com/cn/";
private static final String Android_DEEP_LINK = "myapp://testapplinking/?data=1016";
private String shortLink;
2. In the OnCreate of MainActivity, use the create button to create AppLinking
findViewById(R.id.create).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AppLinking.Builder builder = new AppLinking.Builder()
.setUriPrefix(DOMAIN_URI_PREFIX)
.setDeepLink(Uri.parse(DEEP_LINK))
.setAndroidLinkInfo(new AppLinking.AndroidLinkInfo.Builder()
.setAndroidDeepLink(Android_DEEP_LINK).build());.build());
builder.buildShortAppLinking().addOnSuccessListener(shortAppLinking -> {
shortLink = shortAppLinking.getShortUrl().toString();
TextView showAppLinking = findViewById(R.id.showLink);
showAppLinking.setText(shortLink);
}).addOnFailureListener(e -> {
Log.e("AppLinking", "Failure + "+ e.getMessage());
});
}
});
3. Using the shareLink button to share the AppLinking just created
findViewById(R.id.shareLink).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_TEXT, shortLink);
startActivity(intent);
}
});
5. Receive related AppLinking
There are two steps when receiving. One is to configure the manifest file, and the other is to configure the getAppLinking method at the link entry:
1. Configure the manifest file: Note that this is to configure the Scheme of the DeepLink domain name: For
example, my DeepLink is: DEEP_LINK = "myapp://testapplinking/?data=1016";
private static final String DEEP_LINK = "myapp://testapplinking/?data=1016";
Then the manifest file needs to be configured like this
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:host="testapplinking" android:scheme="myapp" />
</intent-filter>
2. In the main entrance of OnCreate, configure getAppLinking, get and display the link
AGConnectAppLinking.getInstance().getAppLinking(this).addOnSuccessListener(resolvedLinkData -> {
if (resolvedLinkData != null) {
String Result = resolvedLinkData.getDeepLink().toString();
TextView showAppLinking = findViewById(R.id.showLink);
showAppLinking.setText(Result);
}
});
6. Pack test and check the phenomenon.
1. After the application is running, click the Create button to create an AppLinking link and display it on the interface.
2. Click the Share button to share the AppLinking link in the note for temporary storage, and then click the link in the note to open it through the browser. The browser can open the application directly, and the test is complete.
(AppLinking created from the interface is also the same, you can copy it to the sticky note first, and then click to test through the sticky note)
For more details, please see:
https://developer.huawei.com/consumer/cn/doc/development/AppGallery-connect-Guides/agc-applinking-introduction
7. Summary
The integration is simple, the SDK relies on a small size, and can realize cross-platform sharing. Both Android and iOS can support it. There is no need to make different adaptations on different platforms, saving workload.
The operation and promotion can be created on the AGC interface, and the development and sharing function can be created with code on the end side, which is perfect.
Reference documents:
Huawei AGC AppLinking service development document:https://developer.huawei.com/consumer/cn/doc/development/AppGallery-connect-Guides/agc-applinking-introduction
Original link:https://developer.huawei.com/consumer/cn/forum/topic/0204406653695530273?fid=0101271690375130218
Author: Jessyyyyy