The first question: Define a variable of the Map interface type, reference the HashMap implementation class, add key-value pairs, and complete the following requirements (executed in order from 1 to 5):
1. Determine whether the set contains a certain key value, and pass a certain Get the value of a key and print it out;
2. Delete the key-value pair through a key;
add another map set to the map set
3. Return the number of key-value pairs in the set, and print the number And all elements in the set.
4. Determine whether it is empty, and clear the collection if it is.
public class HashMapDemo {
public static void main(String[] args) {
//创建Map对象
Map map = new HashMap();
//添加键值对
map.put(1, "正神黄天禄");
map.put(2, "正神黄天翔");
map.put(3, "正神黄天化");
map.put(4, "东岳大帝");
map.put(5, "青辉武神");
//判断集合中是否包含某一key值,并通过某一key值得到value值,并打印出来;
if (map.containsKey(4) == true) {
System.out.println(map.get(4));
}
//通过某一key删除键值对;
map.remove(1);
Map map1 = new HashMap();
map1.put(6, "娃哈哈");
map1.put(7, "康师傅");
map1.put(8, "可口可乐");
//把另一个map集合添加到此map集合
map.putAll(map1);
//返回集合里key-value对的个数,并打印出来个数和集合中的全部元素。
Collection values = map.values();
for (Object value:values) {
System.out.println(value);
}
//判断是否为空,是则清除集合。
if (map.isEmpty()) {
map.clear();
}
}
}
The second question: Use the implementation class of the Map interface to complete the simulation of employee salary (name-salary):
1) Add a few pieces of information
2) List all employee names
3 List all employee names and their salary
4) Delete name "Tom" employee information
5) Output Jack's salary and add 1,000 yuan to his salary (achieved by value)
6) Increase the salary of all employees whose salary is less than 1,000 yuan by 20% (achieved by value)
public class HashMapDemo01 {
public static void main(String[] args) {
//列出所有员工姓名及其工资
LinkedHashMap link = new LinkedHashMap();
link.put("Tom", 800);
link.put("Toy", 1500);
link.put("Stank", 2000);
link.put("Park", 700);
link.put("Jack", 1200);
//列出所有的员工姓名
Set set = link.keySet();
System.out.println("所有的员工姓名:");
for (Object o : set) {
System.out.println(o);
}
//列出所有员工姓名及其工资
Set set1 = link.entrySet();
System.out.println("所有员工姓名及其工资:");
for (Object o : set1) {
Map.Entry entry = (Map.Entry) o;
System.out.println(entry.getKey() + "," + entry.getValue());
}
//删除名叫“Tom”的员工信息
link.remove("Tom");
//输出Jack的工资,并将其工资加1000元(通过取值实现)
System.out.println("Jack的工资为:" + link.get("Jack"));
//将所有工资低于1000元的员工的工资上涨20%(通过取值实现)
Set set2 = link.entrySet();
for (Object o : set1) {
Map.Entry entry = (Map.Entry) o;
System.out.println("上涨后的工资为:");
if (((int)entry.getValue())< 1000) {
link.put((String)entry.getKey(), (int)entry.getValue()*1.2);
}
}
System.out.println(link);
}
}
Question 3: Use HashMap to store and print the country name information, create a Main class, in its main method a HashMap object ht, add the information of several countries to it, when adding country information, use the country’s English abbreviation (string type) Is the key, and the Chinese name of the country (string type) is the value
data:
CA-Canada (CANADA)
CF-Central Africa (CENTRAL AFRICA)
CG-Congo (CONGO)
CH-Switzerland (SWITZERLAND)
CL-Chile (CHILE)
CM-Cameroon (CAMEROON)
CN-China (CHINA)
CO-Columbia (COLOMBIA)
CR-Costa Rica (COSTA RICA)
CS-Czech (CZECH REPUBIC)
CU-Cuba (CUBA)
CY-Cyprus (CYPRUS)
DE-Germany (GERMANY)
DK-Denmark (DENMARK)
DO-Dominican Republic (DOMINICAN REPUBLIC)
DZ-Algeria (ALGERIA)
Task requirements
1. Query CA country information, if it exists and print its country Chinese name, it will prompt "no such country" if it does not exist.
2. Display the total number of countries in the set;
3. Output the abbreviations of all countries
4. Output the values of all countries
public class Main {
public static void main(String[] args) {
//创建HashMap对象ht
HashMap ht = new HashMap();
//map集合是键值对(key-value)的方法来储存数据
ht.put("CA","加拿大(CANADA)");
ht.put("CF","中非(CENTRAL AFRICA)");
ht.put("CG","刚果(CONGO)");
ht.put("CH","瑞士(SWITZERLAND)");
ht.put("CL","智利(CHILE)");
ht.put("CM","喀麦隆(CAMEROON)");
ht.put("CN","中国(CHINA)");
ht.put("CO","哥伦比亚(COLOMBIA)");
ht.put("CR","哥斯达黎加(COSTA RICA)");
ht.put("CS","捷克(CZECH REPUBIC)");
ht.put("CU","古巴(CUBA)");
ht.put("CY","塞浦路斯(CYPRUS)");
ht.put("DE","德 国(GERMANY)");
ht.put("DK","丹麦(DENMARK)");
ht.put("DO","多米尼加共和国(DOMINICAN REPUBLIC)");
ht.put("DZ","阿尔及利亚(ALGERIA)");
if (ht.get("CA") != null) {
System.out.println("国家中文名称:"+ht.get("CA"));
}else{
System.out.println("无此国家");
}
System.out.println("一共有"+ht.size()+"个国家");
//Set keySet():返回所有key构成的Set集合
Set set = ht.keySet();
for (Object o:set) {
System.out.println(o);
}
//Set keySet():返回所有key构成的Set集合
Collection values = ht.values();
for (Object value: values) {
System.out.println(value);
}
}
}
```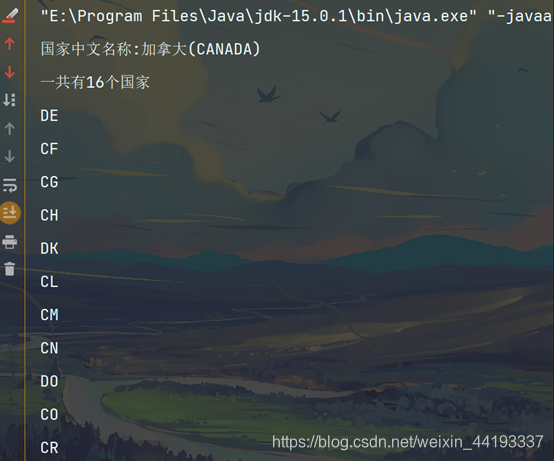
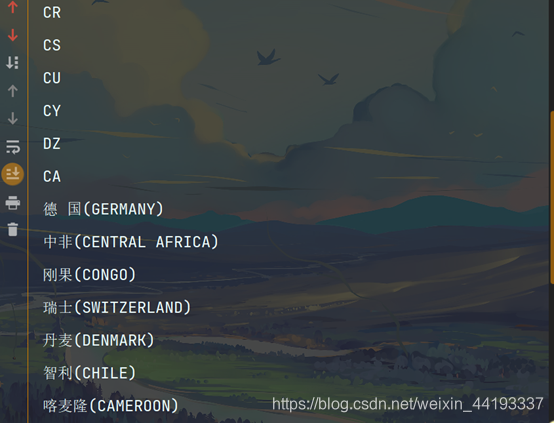
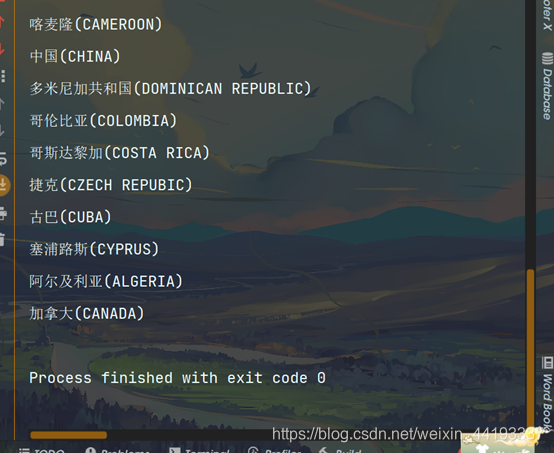