2. Experimental content
- Programming implementation: Please check the following program to find out the errors and correct them. Then debug it on the machine to make it run normally. Enter the values of hours, minutes, and seconds from the keyboard at runtime, and check whether the output is correct.
code: After testing (Error: [Error] 'int Time::hour' is private) #include <iostream> using namespace std; class Time { private:// (private member, can only be used inside the class, void set_time(void);// It is also defined inside the class when it is defined, void show_time(void);//just need to add private and public) public: int hour; int minute; int sec; };Time t; int set_time(void) { cin>>t.hour; cin>>t.minute; cin>>t.sec;} int show_time(void) {
cout<<t.hour<<":"<<t.minute<<":"<<t.sec<<endl;} int main() {
set_time(); show_time(); } Program testing and running results: 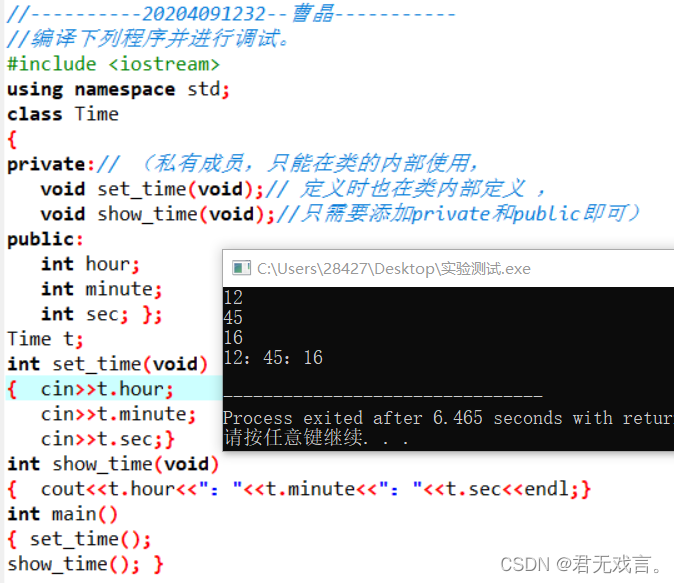
- Run the program:
code: /* (analysis: Student(int n, float s): num(n), score(s){} with void change(int n,float s){num=n;score=s;}功能相同都是初始化num与score。 而前面有了const则函数值不会改变) */ #include <iostream> using namespace std; class Student { public: Student(int n,float s):num(n),score(s){} void change(int n,float s){num=n;score=s;} void display(){cout<<num<<" "<<score<<endl;} private: int num; float score;}; int main() { Student stud(101,78.5); stud.display(); stud.change(101,80.5); stud.display(); return 0;} 程序测试及运行结果: 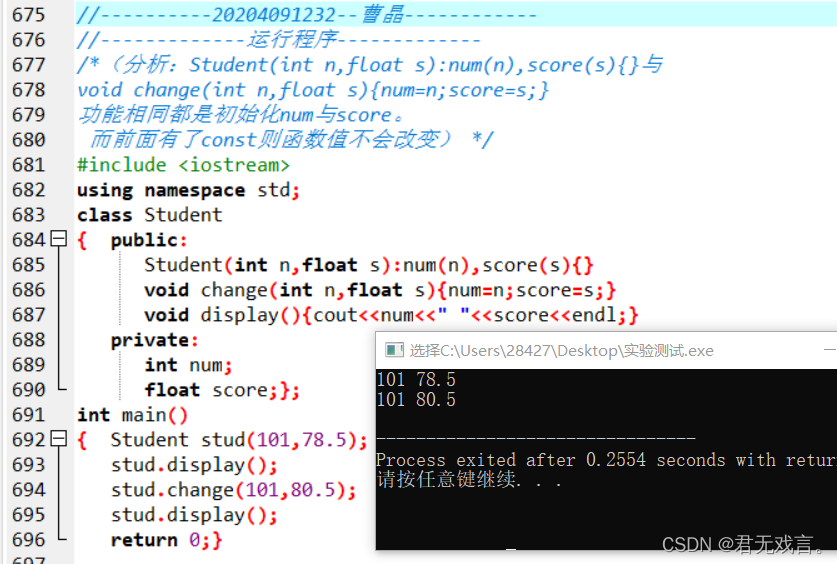
- 编程实现:根据测试程序及测试程序的输出结果,编写类Welcome.
程序代码: #include<iostream> #include<stdio.h> #include<string.h> using namespace std; class Weclome //定义类 {
public:Weclome(); Weclome(Weclome &we); void talk(); void get(char s[]); void display(); private: char str[15];}; void Weclome::talk(){} void Weclome::get(char*const){} void Weclome::display() //定义多个调用函数 {
cout<<"Hou are you?"<<endl; cout<<"Fine,thank you."<<endl; cout<<"OK"<<endl; //定义输出内容 } Weclome::Weclome(class Weclome &) {
cout<<"Thank you."<<endl; } Weclome::Weclome() {
cout<<"Weclome"<<endl; } int main() //主函数 {
Weclome we; Weclome you(we); you.display(); you.get("Thank you."); you.talk(); you.display(); return 0; } 程序测试及运行结果: 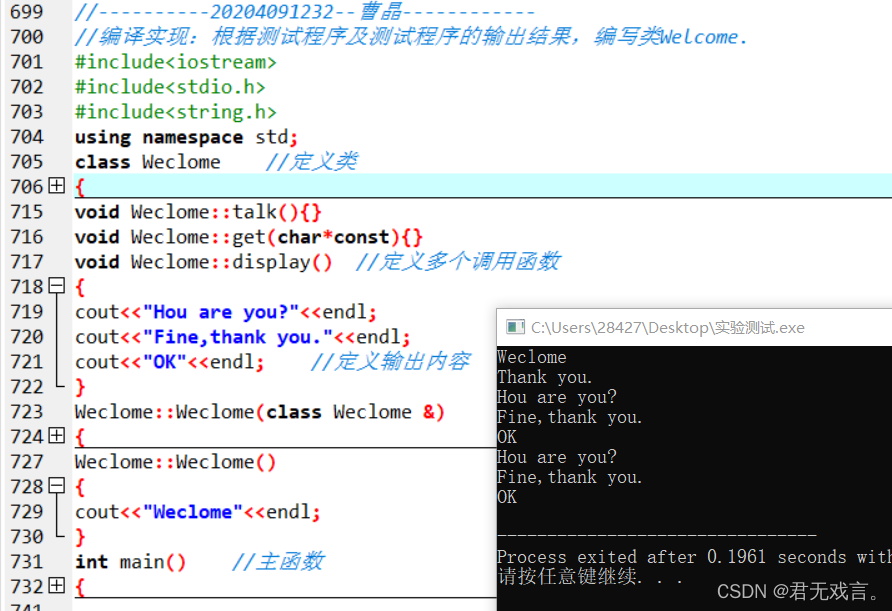
- 编程实现:编写一个复数类型complex,其中含有若干个成员函数,使用该类可以完成复数的加法,复数的输入输出。
程序代码: #include <iostream> using namespace std; class Complex {public: Complex(){real=0; imag=0;} //默认构造函数 Complex(double r){real=r; imag=0;} //转换构造函数 Complex(double r, double i){real=r; imag=i;} //构造函数 friend Complex operator+(Complex c1, Complex c2); //重载运算符+函数为友元函数 void display(); //定义display函数 private: double real; double imag;}; //重载运算符+函数 Complex operator+(Complex c1, Complex c2) { return Complex(c1.real+c2.real, c1.imag+c2.imag);} //display函数 void Complex::display() { cout<<real; if (imag>=0) cout<<"+"; cout<<imag<<"i"<<endl;} int main(){
Complex c1(1, -2), c2(3, 4), c3; //定义Complex类对象c1,c2,c3 int i;c3=c1+c2; c3.display(); cout<<"Please enter number: "; cin>>i; c3=i+c1; c3.display(); c3=c1+i; c3.display(); return 0; } 程序测试及运行结果: 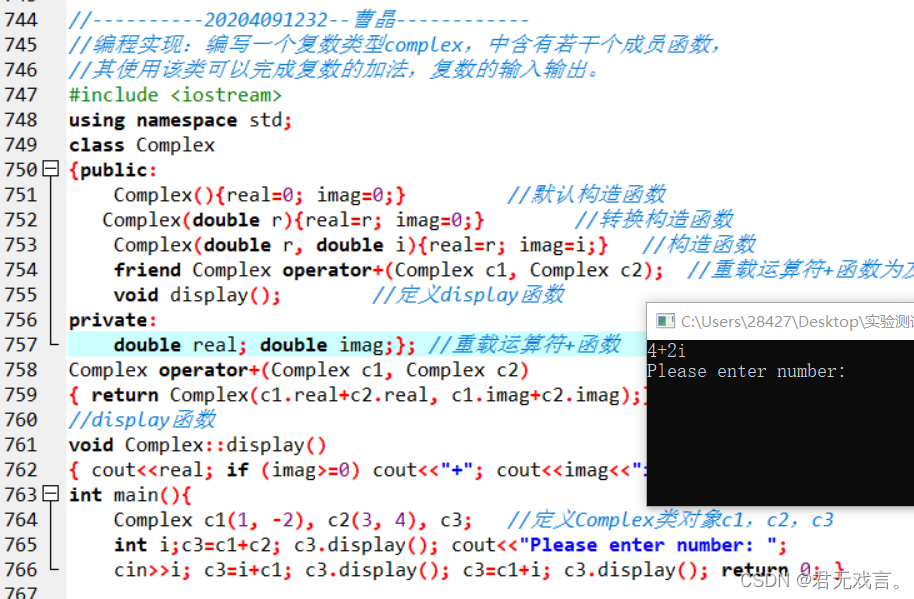 |