This article refers to the C++ one-way circular linked list to realize the arrangement. First understand the structural framework, and then use c to implement the content according to the video, which can also have a higher improvement on c.
Article Directory
The one-way circular linked list is very similar to the one-way linked list. For the detailed theory and implementation process of the one-way linked list, please refer to "C++ Data Structure X _04_Single-way linked list framework construction, implementation and testing" . In fact, the only difference between the one-way circular linked list and the one-way linked list is that the pointer field of the tail node points to a different point. For the tail node of the one-way linked list, since there is no subsequent node, the pointer field needs to point to NULL. The one-way circular linked list points the pointer field of the tail node to the head node, and the first bits are connected to form a one-way circular linked list. The specific form is: the specific
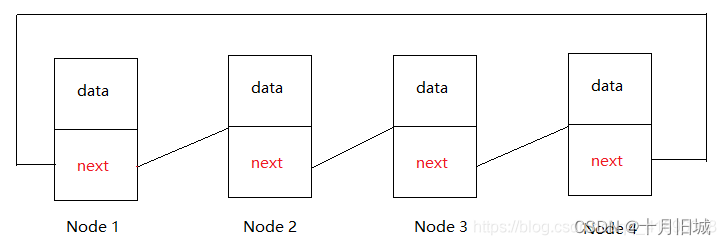
implementation code is:
1. Linked list definition
//定义节点
class circle_node
{
public:
int data;
circle_node* next;
};
//定义循环链表
class circle_list
{
public:
circle_node* head;
int size;
};
//链表初始化
circle_list* list_init()
{
circle_list* L =new circle_list;
L->size=0;
L->head=new circle_node;
L->head->data=NULL;
L->head->next=L->head; //头指针初始指向自己
return L;
}
The only difference between this and the one-way linked list is that the head pointer L->head->next=L->head points to itself instead of NULL.
2. Linked list insertion
//插入链表
void list_insert(circle_list *L,int pos,int data)
{
//创建新指针new_node;
circle_node* new_node=new circle_node;
new_node->data=data;
new_node->next=NULL;
//根据位置查找pos的前一个节点
circle_node* pcurrent=L->head;
for (int i = 0; i < pos; i++)
{
pcurrent=pcurrent->next;
}
//将new_node插入
new_node->next=pcurrent->next;
pcurrent->next=new_node;
L->size++;
}
The insertion method here is exactly the same as that of a singly linked list.
3. Print the linked list (note that the printing process skips the head node head)
//打印链表,按num自由指定打印数据个数
void list_print(circle_list *L,int num)
{
circle_node* pcurrent=L->head->next;
for (int i = 0; i <num; i++)
{
if (pcurrent==L->head)
{
pcurrent=L->head->next;
}
cout<<pcurrent->data<<"\t";
pcurrent=pcurrent->next;
}
cout<<endl;
}
Since the one-way circular linked list is connected end to end, you can specify num according to the number of nodes to be printed when printing the linked list.
4. Experiment
int main()
{
//创建单向循环链表
circle_list* L=list_init();
//插入数据0~9
for (int i = 0; i < 10; i++)
{
list_insert(L,i,i);
}
cout<<"链表中数据为:"<<endl;
list_print(L,10);
cout<<"链表中2倍数据为:"<<endl;
list_print(L,20);
system("pause");
return 0;
}
The result is:
5. Learning video: Circular linked list idea ; Circular linked list framework ; Circular linked list framework implementation ; Circular linked list test