Explanation: Vue provides this injection method, which greatly facilitates the centralized management of data and makes it easy for developers to deal with the problem of parameter passing in multi-level components. Reference documentation ( https://vuejs.org/guide/components/provide-inject.html )
Background: Typically, props are used when we need to pass data from a parent component to a child component. Imagine such a structure: there are some multi-level nested components, forming a huge component tree, and a deep child component needs part of the data in a distant ancestor component. In this case, if you only use props, you have to pass them down the component chain, which is very cumbersome:
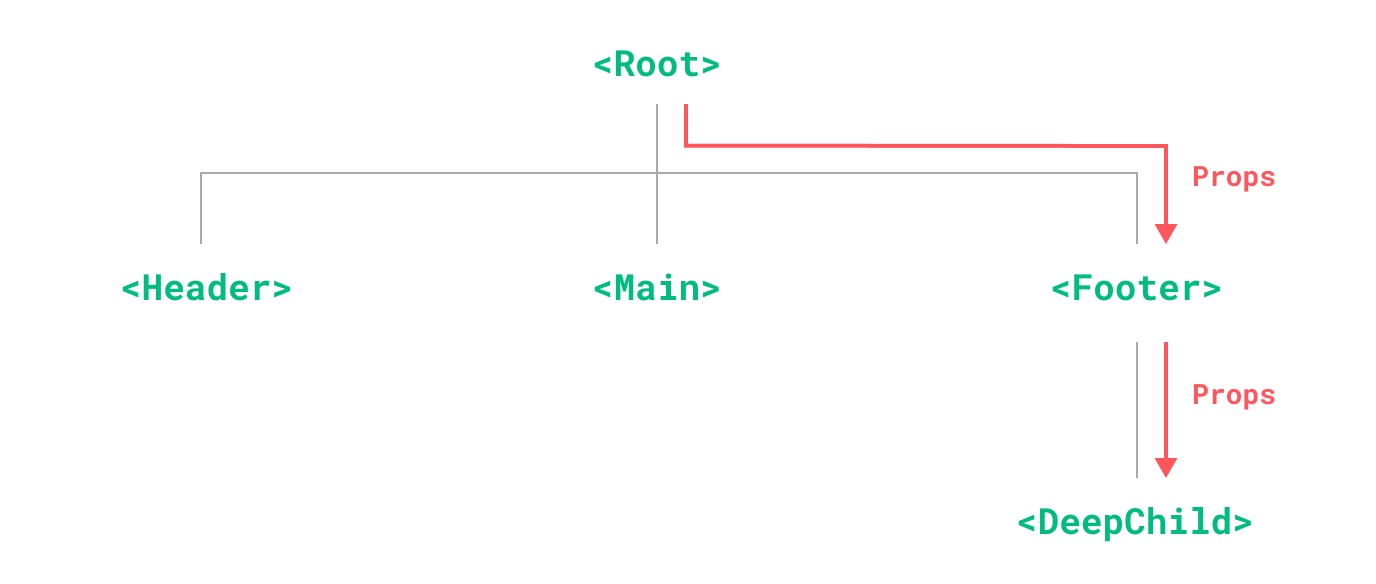
Note that although the <Footer> component here may not care about these props at all, in order for <DeepChild> to access them, they still need to be defined and passed down. If the component chain is very long, it may affect more components on this road. This problem is called "prop step-by-step transparent transmission", and it is obviously a situation we want to avoid as much as possible.
provide and inject can help us solve this problem. A parent component acts as a dependency provider for all its descendants. Any descendant of the component tree, no matter how deep the hierarchy is, can inject the dependencies provided by the parent component for the entire chain.
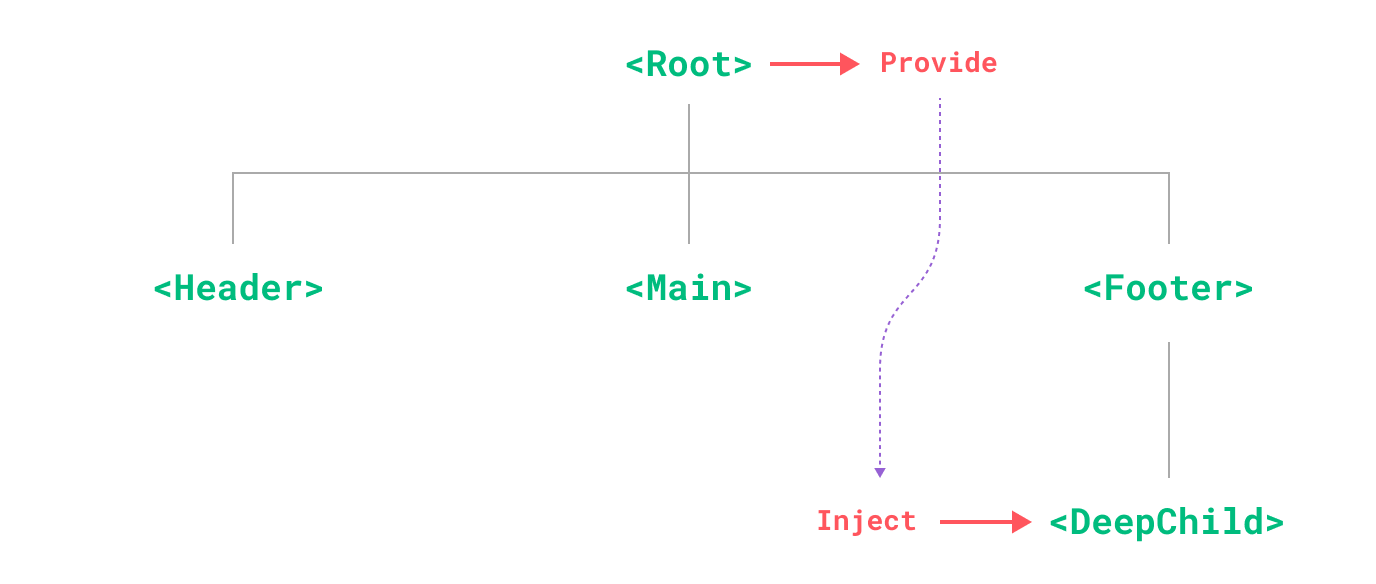
use:
export default {
data() {
return {
message: 'hello!'
}
},
provide() {
return {
message: this.message
}
}
}
//注入
export default {
inject: ['message'],
created() {
console.log(this.message) // injected value
}
}
//或
export default {
inject: ['message'],
data() {
return {
fullMessage: this.message
}
}
}