In Android program development, we often use Shape to define various shapes.
First, let’s understand what labels are under Shape and what they mean:
solid: fill android:color specifies the color of the fill
gradient: gradient android:startColor and android:endColor are the starting and ending colors respectively.
android:angle is the gradient angle, which must be an integer multiple of 45. In addition, the default mode of the gradient is android:type="linear", that is, a linear gradient.
The gradient can be specified as a radial gradient, android:type="radial", and the radial gradient needs to specify a radius android:gradientRadius="50".
The position corresponding to the angle value is shown in the figure:
stroke: stroke android:width="2dp" the width of the stroke, android:color the color of the stroke. We can also make the stroke into a dotted line. The setting method is: android:dashWidth="5dp" android:dashGap="3dp" where android:dashWidth represents the width of a horizontal line like '-', android:dashGap represents it distance apart
corners: rounded corners android:radius is the radian of the corner, the larger the value, the rounder the corner. We can also set the four corners to different angles,
If five attributes are set at the same time, the Radius attribute is invalid
android:Radius="20dp" Set the radius of the four corners
android:topLeftRadius="20dp" Set the radius of the upper left corner android:topRightRadius="20dp" Set the radius of the upper right corner android:bottomLeftRadius="20dp" Set the radius of the lower right corner android:bottomRightRadius="20dp" Set the radius of the lower left corner
padding: The interval can be set in the four directions of up, down, left, and right
Here we look at a simple example, ShapDemo, first define two xml files under the drawable folder:
The content of button_bg.xml is as follows:
<?xml version="1.0" encoding="utf-8"?><!-- 填充 -->
<solid android:color="#ff9d77" /> <!-- 定义填充的颜色值 -->
<!-- 描边 -->
<stroke
android:width="2dp"
android:color="#fad3cf" /> <!-- 定义描边的宽度和描边的颜色值 -->
<!-- 圆角 -->
<corners
android:bottomLeftRadius="5dp"
android:bottomRightRadius="5dp"
android:topLeftRadius="5dp"
android:topRightRadius="5dp" /> <!-- 设置四个角的半径 -->
<!-- 间隔 -->
<padding
android:bottom="10dp"
android:left="10dp"
android:right="10dp"
android:top="10dp" /> <!-- 设置各个方向的间隔 -->
The content of button_pressed_bg.xml is as follows:
<?xml version="1.0" encoding="utf-8"?><!-- 渐变 -->
<gradient
android:endColor="#FFFFFF"
android:gradientRadius="50"
android:startColor="#ff8c00"
android:type="radial" />
<!-- 描边 -->
<stroke
android:dashGap="3dp"
android:dashWidth="5dp"
android:width="2dp"
android:color="#dcdcdc" />
<!-- 圆角 -->
<corners android:radius="5dp" />
<!-- 间隔 -->
<padding
android:bottom="10dp"
android:left="10dp"
android:right="10dp"
android:top="10dp" />
Let me explain here that the dash parameter is set in the stroke, making the edge of the graph a dotted line.
Add a selector button.xml file under the drawable folder, the content is as follows:
<?xml version="1.0" encoding="utf-8"?><item android:drawable="@drawable/button_pressed_bg" android:state_pressed="true"></item>
<item android:drawable="@drawable/button_bg"></item>
The meaning of this file has been mentioned before, button_bg is displayed under normal (normal) conditions, and button_pressed_bg is displayed under press (pressed) conditions.
Let's take a look at the content of activity_main.xml in the layout directory:
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/button"
android:text="TestShapeButton" />
Directly specify the background as button.xml under the drawable folder.
The screenshot of the program running is as follows:
Finally, I would like to share with you a set of "Advanced Materials on the Eight Modules of Android" written by Alibaba's senior architects to help you systematically organize messy, scattered, and fragmented knowledge, so that you can systematically and efficiently master all aspects of Android development. Knowledge points.
Due to the large content of the article and the limited space, the information has been organized into PDF documents. If you need the complete document of "Advanced Materials on Eight Modules of Android", you can add WeChat to get it for free!
PS: (There are also small benefits for using the ChatGPT robot at the end of the article!! Don’t miss it)
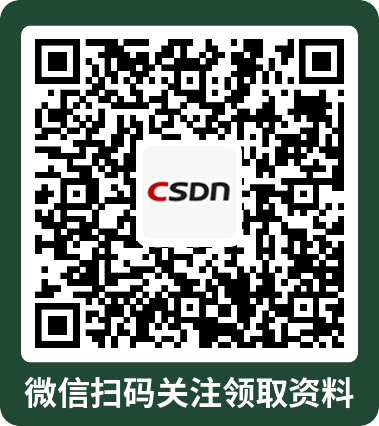
"Android Eight Modules Advanced Notes"
Compared with the fragmented content we usually read, the knowledge points in this note are more systematic, easier to understand and remember, and are strictly arranged according to the knowledge system.
1. Source code analysis collection
2. Collection of open source frameworks
At the same time, a WeChat group chat robot based on chatGPT has been built here to answer difficult technical questions for everyone 24 hours a day .
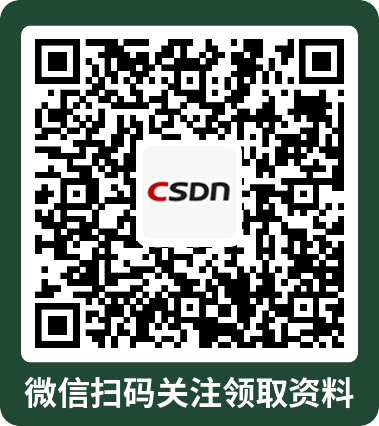