1. Call the browser print plug-in
The PDF printed in this way is relatively high-definition, and the paging will not truncate text and pictures, but the background image will be truncated.
1. Print directly
Directly call the browser's print function to print the entire page
function preview () {
window.print();
}
2.Print the specified area
Print through the start tag and end tag to print partial pages
<!--startprint-->
<div>
打印的内容
</div>
<!--endprint-->
function preview () {
let bdhtml=window.document.body.innerHTML;
let sprnstr="<!--startprint-->"; //开始打印标识字符串有17个字符
let eprnstr="<!--endprint-->"; //结束打印标识字符串
let prnhtml=bdhtml.substr(bdhtml.indexOf(sprnstr)+17); //从开始打印标识之后的内容
prnhtml=prnhtml.substring(0,prnhtml.indexOf(eprnstr)); //截取开始标识和结束标识之间的内容
window.document.body.innerHTML=prnhtml; //把需要打印的指定内容赋给body.innerHTML
window.print(); //调用浏览器的打印功能打印指定区域
window.document.body.innerHTML=bdhtml;//重新给页面内容赋值;
return false;
}
Note : 1. If the area you want to print contains a vue assignment object, then your start and end identifiers need to be placed outside the ID bound to the instantiated VUE. As follows, the Vue instantiation bound is the ID "#content", Then the identifier must be placed outside the "#content" element
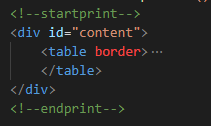
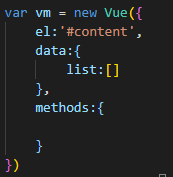
2. The calling method of this printed function cannot be called by vue's @click. Please use js's onclick to call it. The function is allowed to be placed in vue's methods. Call: οnclick="vm.preciew()". Why is this? Woolen cloth? Because at the end of the function, we reassign the specified area. If it is @click, the reassignment cannot bind the click event to the button again. The native click event can be reassigned.
Of course, if you only use js or jquery, then you will not need to consider the above two issues.
3. Remove the header and footer when printing
<style media="print">
@page {
size: auto; /* auto是初始值 */
margin: 0mm; /* 这会影响打印机设置中的边距 */
}
</style>
Special note: When printing using a browser, print from top to bottom, and the text box will be cropped and divided during paging, as shown in the figure:
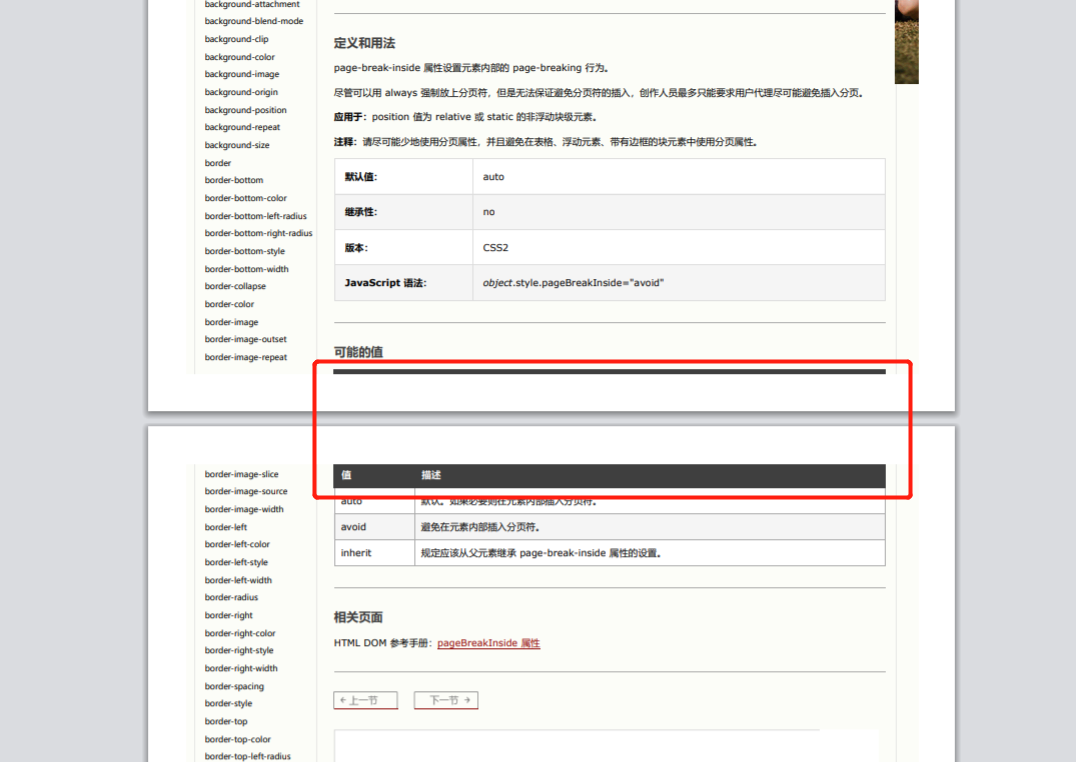
You can use css properties:
page-break-inside: avoid;
Set the paging behavior inside the table element to avoid paging. When printing, this box will be considered by the browser as a whole. If the first page cannot fit, this box will be ranked on the second page.
2. Directly export pdf through jspdf and html2canvas libraries
The PDF exported in this way is relatively blurry. The images in the code must be in base64 format to be displayed, and paging will truncate text and images;
But there is no need to pop up the browser print box
<button onclick="pdfMap()">点击下载</button>
<script src="https://unpkg.com/[email protected]/dist/jspdf.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/0.4.1/html2canvas.js"></script>
function pdfMap() {
html2canvas(document.body, {
onrendered: function (canvas) {
var contentWidth = canvas.width;
var contentHeight = canvas.height;
var pageHeight = contentWidth / 595.28 * 841.89; //一页pdf显示html页面生成的canvas高度;
var leftHeight = contentHeight; //未生成pdf的html页面高度
var position = 0; //pdf页面偏移
//a4纸的尺寸[595.28,841.89],html页面生成的canvas在pdf中图片的宽高
var imgWidth = 555.28;
var imgHeight = 555.28 / contentWidth * contentHeight;
var pageData = canvas.toDataURL('image/jpeg', 1.0);
var pdf = new jsPDF('', 'pt', 'a4');
//有两个高度需要区分,一个是html页面的实际高度,和生成pdf的页面高度(841.89)
//当内容未超过pdf一页显示的范围,无需分页
if (leftHeight < pageHeight) {
pdf.addImage(pageData, 'JPEG', 20, 0, imgWidth, imgHeight);
} else {
while (leftHeight > 0) {
pdf.addImage(pageData, 'JPEG', 20, position, imgWidth, imgHeight)
leftHeight -= pageHeight;
position -= 841.89;
//避免添加空白页
if (leftHeight > 0) {
pdf.addPage();
}
}
}
pdf.save('content.pdf');
}
})
}