官方链接:https://github.com/wonday/react-native-pdf
#安装
npm install rn-fetch-blob --save
npm install react-native-pdf --save
#链接依赖
react-native link rn-fetch-blob
react-native link react-native-pdf
import React from 'react';
import {Dimensions, StyleSheet, View} from 'react-native';
import Pdf from 'react-native-pdf';
import SplashScreen from 'react-native-splash-screen'
export default class App extends React.Component {
constructor(props) {
super(props);
this.state = {
totalPage: 1,
currentPage: 1
};
}
async componentDidMount() {
await SplashScreen.hide();
this.timer = setInterval(() => {
let {currentPage, totalPage} = this.state;
currentPage++;
if (currentPage > totalPage) {
currentPage = 1;
}
console.log('页数开始增加', currentPage);
this.setState({currentPage});
}, 3000);
}
componentWillUnmount() {
this.timer && clearInterval(this.timer);
}
render() {
const source = {uri: 'http://samples.leanpub.com/thereactnativebook-sample.pdf', cache: true};
const {currentPage} = this.state;
return (
<View style={styles.container}>
<Pdf
source={source}
horizontal={true}
page={currentPage}
enableRTL={true}
onLoadComplete={totalPage => {
this.setState({totalPage});
}}
onPageChanged={(page, numberOfPages) => {
console.log(`当前页:${page},总页数:${numberOfPages}`);
}}
onError={(error) => {
console.log(error);
}}
style={styles.pdf}/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'flex-start',
alignItems: 'center',
marginTop: 25,
},
pdf: {
flex: 1,
width: Dimensions.get('window').width,
}
});
- Renderings
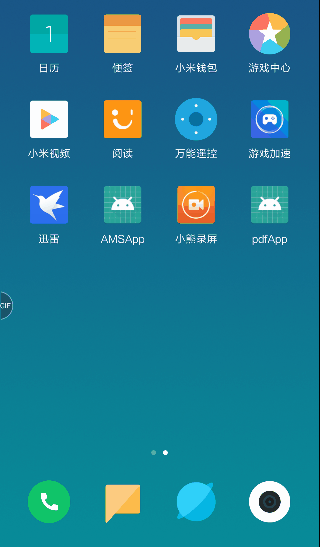