First, look for:
Sequential search:
Hash table is an easy way to find a set of stored items thereof, each hash table location, commonly referred to as a slot, can hold an item, and named by integer values starting from 0, for example, named 0 slot, slot 1 ...... named, initially, does not include the hash table entries, each slot is empty, we can achieve the hash table is initialized by each element of a list is none. And a mapping between items belonging to the groove in the hash table is referred to as a hash function. The hash function accepts any items in the collection, and the slot name in the range (between 0 ~ m-1) returns an integer
hash function:
Remainder number: only one size of table entries and dividing it, as the remaining portion to return its hash value will result in a range of the slot name (h (item) = item% 11 (size of the slot)) in
Upon calculating the hash value, we can treat each item into the specified location in the hash table
A given set of items, each item is mapped to a unique groove perfect hash function is referred to as a hash function, a way to always have a perfect hash function is to increase the size of the hash table, so that the items can be accommodated in the range for each possible value
Many simple extension remainder method:
1, packet summation method
2, square reindeer method
3, create a hash function based on the character of items such as: ord ( 'c') == 99
specific principles hash lookup of the blog: https://blog.csdn.net/weixin_41362649/article/details/81865829
Because the slot is calculated by the computer name hash function, then hash table lookup, he complexity is O (1)
Second, the sort:
bubble:
For like the two lists are compared, the larger items in the back of the smaller items, so, compare n-1 times, the last intent of the list is the biggest one, and then the remaining n- a second time to find the biggest item, and then put the final, this is the case, so the cycle n times, the entire list will be incremental list, but you can also see that complexity is O (n 2 ).
select:
Select sort is optimized for bubbling, which directly find the largest item in the list, and then placed in the last position in the list, then the remaining n-1 find the maximum term for a second term, and then into the final, then the remaining n-1 find the maximum term for a second term, and then put the final. It can be seen that the selection sort Bubble sort have the same number of comparison, and therefore O (n- 2 ). However, due to the reduction in the number of exchange, choose the sort usually execute faster.
insert:
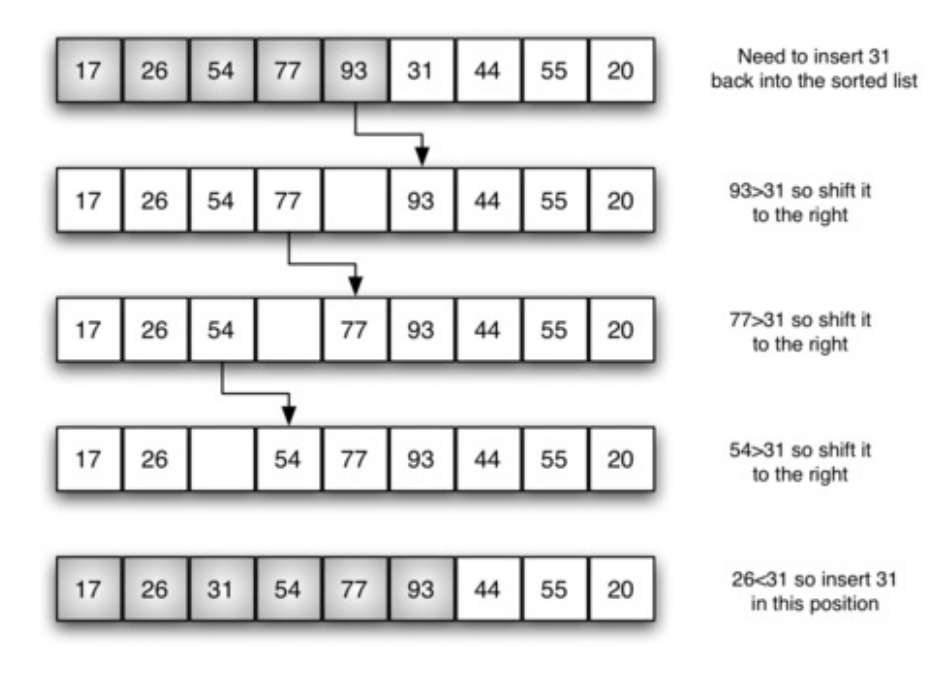
To [1726547793] The insert 31 has an ordered list, then sort by shifting the sub-table, this cycle, the maximum number of comparisons is the sum of insertion sort n-1 integers, the same, is O (n- 2 ). Typically, the shift operation only about one third of the exchange processing, because the allocation is performed only once, insertion sort have very good performance.
Hill:
归并:
归并过程为:比较a[i]和b[j]的大小,若a[i]≤b[j],则将第一个有序表中的元素a[i]复制到r[k]中,并令i和k分别加上1;否则将第二个有序表中的元素b[j]复制到r[k]中,并令j和k分别加上1,如此循环下去,直到其中一个有序表取完,然后再将另一个有序表中剩余的元素复制到r中从下标k到下标t的单元。归并排序的算法我们通常用递归实现,先把待排序区间[s,t]以中点二分,接着把左边子区间排序,再把右边子区间排序,最后把左区间和右区间用一次归并操作合并成有序的区间[s,t]。
发现了一个C语言的关于归并的讲解https://www.cnblogs.com/Java3y/p/8631584.html,虽然语言不同,但是数据结构是一样的
快速:
快速排序通过在列表中随机确定一个参照值,进行跨越式比较,快速排序之所比较快,因为相比冒泡排序,每次交换是跳跃式的。每次排序的时候设置一个基准点,将小于等于基准点的数全部放到基准点的左边,将大于等于基准点的数全部放到基准点的右边。这样在每次交换的时候就不会像冒泡排序一样每次只能在相邻的数之间进行交换,交换的距离就大的多了。因此总的比较和交换次数就少了,速度自然就提高了。当然在最坏的情况下,仍可能是相邻的两个数进行了交换。因此快速排序的最差时间复杂度和冒泡排序是一样的都是O(N2),它的平均时间复杂度为O(NlogN)。
具体的话,在博客中很详细https://blog.csdn.net/adusts/article/details/80882649