-
不在xml文件中配置bean,而是直接在实体类增加注解,自动实现依赖注入。
-
适用于简单的属性,如果一堆List、set要注入还是用xml配置比较好。
-
xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!--指定要扫描的包,这个包下的注解就会生效--> <context:component-scan base-package="com.rzp.pojo"/> <context:annotation-config/> </beans>
-
bean
package com.rzp.pojo; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; //组件--相当于 <bean id="user" class="com.rzp.pojo.User"/> @Component public class User { //相当于p <property name = "name" value = "rzp"/> @Value("rzp") public String name; }
-
测试
import com.rzp.pojo.User; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MyTest { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationcontext.xml"); User user = context.getBean("user", User.class); System.out.println(user.name); } }
-
这样xml文件不需要配置
扫描二维码关注公众号,回复:
10486319 查看本文章
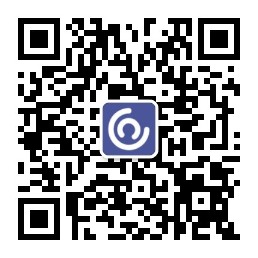
衍生的注解
-
以下四个注解功能一样,都相当于注册到Spring容器中,只是对于MVC层中不同的层我们使用不同的注解
@Component public class User { } @Service public class UserService { } @Controller public class UserController { } @Repository public class UserDao { }
作用域
-
@Scope("prototype")
//组件--相当于 <bean id="user" class="com.rzp.pojo.User"/> @Component @Scope("prototype") public class User { //相当于p <property name = "name" value = "rzp"/> @Value("rzp") public String name; }
属性的自动装配
-
见03
使用Java配置类替代xml文件
-
在上文还需要使用xml文件,Spring4后完全不需要使用xml文件了,官方也更推荐这个方式。
-
实体类
package com.rzp.pojo; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component public class User { @Value("rzp") private String name; @Override public String toString() { return "User{" + "name='" + name + '\'' + '}'; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
package com.rzp.config; import com.rzp.pojo.User; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Import; //增加Configuration,就会被Spring注册到容器中,因为Configuration也是一个Component //Configuration代表一个配置类,原来的xml文件一样 @Configuration @ComponentScan("com.rzp.pojo") //扫描包 @Import(WholeConfig2.class) //和bean类似,导入其他配置文件 public class WholeConfig { //相当于bean标签,方法名字相当于bean中的id,方法返回值就相当于class属性 @Bean public User user(){ return new User(); //返回要注入的对象 } }
-
测试
import com.rzp.config.WholeConfig; import com.rzp.pojo.User; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; public class MyTest { public static void main(String[] args) { //如果完全使用了配置类,就只能通过AnnotationConfig上下文来获取容器,通过配置类Class对象加载 ApplicationContext context = new AnnotationConfigApplicationContext(WholeConfig.class); User user = context.getBean("user", User.class); System.out.println(user.getName()); } }