题目是LeetCode第182场周赛的第三题,链接:设计地铁系统。具体描述见原题链接。
这道题其实偏向于数据结构的设计,这里直接用map来保存行程信息,主要是{乘客id–>入站名与入站时间},{出站名–>{入站名–>总时长与总人次}}。这么做的好处在于可以将各种操作的时间复杂度都控制在 。
JAVA版代码如下:
class UndergroundSystem {
private Map<String, Map<String, int[]>> end2in;
private Map<Integer, StationAndTime> inST;
public UndergroundSystem() {
end2in = new HashMap<>();
inST = new HashMap<>();
}
public void checkIn(int id, String stationName, int t) {
inST.put(id, new StationAndTime(stationName, t));
}
public void checkOut(int id, String stationName, int t) {
StationAndTime in = inST.get(id);
String in_station = in.getStation();
int in_time = in.getTime();
Map<String, int[]> inTimeCount = end2in.getOrDefault(stationName, new HashMap<>());
int[] stationTime = inTimeCount.getOrDefault(in_station, new int[] {0, 0});
stationTime[0] += t - in_time;
++stationTime[1];
inTimeCount.put(in_station, stationTime);
end2in.put(stationName, inTimeCount);
}
public double getAverageTime(String startStation, String endStation) {
int[] timeAndCount = (end2in.get(endStation)).get(startStation);
return timeAndCount[0] * 1.0 / timeAndCount[1];
}
}
class StationAndTime {
private String station;
private int time;
public StationAndTime(String name, int time) {
this.station = name;
this.time = time;
}
public String getStation() {
return this.station;
}
public int getTime() {
return this.time;
}
}
/**
* Your UndergroundSystem object will be instantiated and called as such:
* UndergroundSystem obj = new UndergroundSystem();
* obj.checkIn(id,stationName,t);
* obj.checkOut(id,stationName,t);
* double param_3 = obj.getAverageTime(startStation,endStation);
*/
提交结果如下:
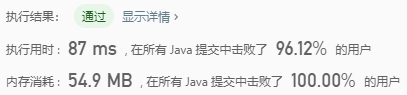
Python版代码如下:
class UndergroundSystem:
def __init__(self):
# {出站名 : {入站名 : [总时间, 总人次]}}
self.end2in = {}
# {id : [入站名, 入站时间]}
self.inST = {}
def checkIn(self, id: int, stationName: str, t: int) -> None:
self.inST[id] = [stationName, t]
def checkOut(self, id: int, stationName: str, t: int) -> None:
in_station = (self.inST[id])[0]
in_time = (self.inST[id])[1]
# 更新一条路线的总时长与总人次
if stationName in self.end2in:
timeCount = (self.end2in[stationName])[in_station] if in_station in self.end2in[stationName] else [0, 0]
timeCount[0] += t - in_time
timeCount[1] += 1
(self.end2in[stationName])[in_station] = timeCount
else:
self.end2in[stationName] = {in_station : [t - in_time, 1]}
def getAverageTime(self, startStation: str, endStation: str) -> float:
timeCount = (self.end2in[endStation])[startStation]
return timeCount[0] / timeCount[1]
# Your UndergroundSystem object will be instantiated and called as such:
# obj = UndergroundSystem()
# obj.checkIn(id,stationName,t)
# obj.checkOut(id,stationName,t)
# param_3 = obj.getAverageTime(startStation,endStation)
提交结果如下:
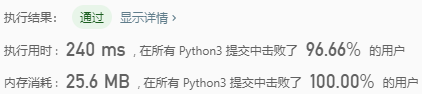