#ifndef IPANDA_H
#define IPANDA_H
#include <string>
#include <iostream>
template <typename t>int operator+(t&,t&);
template <typename type>
class panda
{
private:
std::string _name;
std::string name_;
std::string _name_;
int a;
type k;
public:
panda();
panda(const std::string &name1, const std::string &name2,const std::string &name3,int,type );
~panda();
const char* str(std::string _name) { return _name.c_str(); }
friend int operator-(panda<type>& d,panda<type> &e);
friend int operator+<>(panda<type>&, panda<type>&);
operator int();
panda<type>& operator=(panda<type>& );
template<typename T> friend std::ostream& operator <<(std::ostream&,panda<T>&);
template<typename TT>friend void show(TT&);
};
template <typename type>
panda<type>::panda()
{
_name = "a";
name_ = "b";
_name_ = "c";
a = 2;
}
template <typename type>
panda<type>::panda(const std::string& name1, const std::string& name2, const std::string& name3, int b, type K)
{
_name_ = name2;
_name = name1;
name_ = name3;
a = b;
k = K;
}
template<typename type>
panda<type>::~panda()
{
std::cout << "the destructor is called" << std::endl;
std::cout << std::endl;
}
int operator-(panda<double>& d, panda<double>& e)
{
return d.a - e.a;
}
template<typename t>
int operator+(t& d, t&e)
{
return d.a + e.a;
}
template <typename type>
panda<type>::operator int()
{
return (*this).a;
}
template <typename type>
panda<type>& panda<type>::operator =(panda<type>& z)
{
(*this).a = z.a;
this->_name = z._name;
this->name_ = z.name_;
this->_name_ = z._name_;
this->k = z.k;
return *this;
}
template <typename T> std::ostream& operator<<(std::ostream& os,panda<T>&c)
{
using std::endl;
os << c._name << endl;
os << c.name_ << endl;
os << c._name_ << endl;
os << c.a << endl;
return os;
}
template<typename TT> void show(TT& p)
{
std::cout << p._name<<std::endl;
}
#endif
#include <iostream>
#include "ipanda.h"
int main()
{
using std::cin;
using std::cout;
using std::endl;
panda<double> panda1("A", "B", "C", 5, 5.66);
panda<double> panda2;
panda<double> panda3;
cout << "重载减法运算符(模板类的非模板友元函数):\npanda1-panda2;\n";
cout << (panda1 - panda2) << endl;
cout << "重载加法运算符(模板类的约束模板友元函数):\npanda1+panda2;\n";
cout << (panda1 + panda2) << endl;
panda3=panda2=panda1;
cout << "重载<<运算符(模板类的非约束模板友元函数):\n";
cout << "panda1:\n"<<panda1 << endl;
cout << "panda2\n"<<panda2 << endl;
cout << "panda3\n"<<panda3<<endl;
show(panda1);
cout << int(panda2)<<endl;
cout << panda3.operator int()<<endl;
return 0;
}
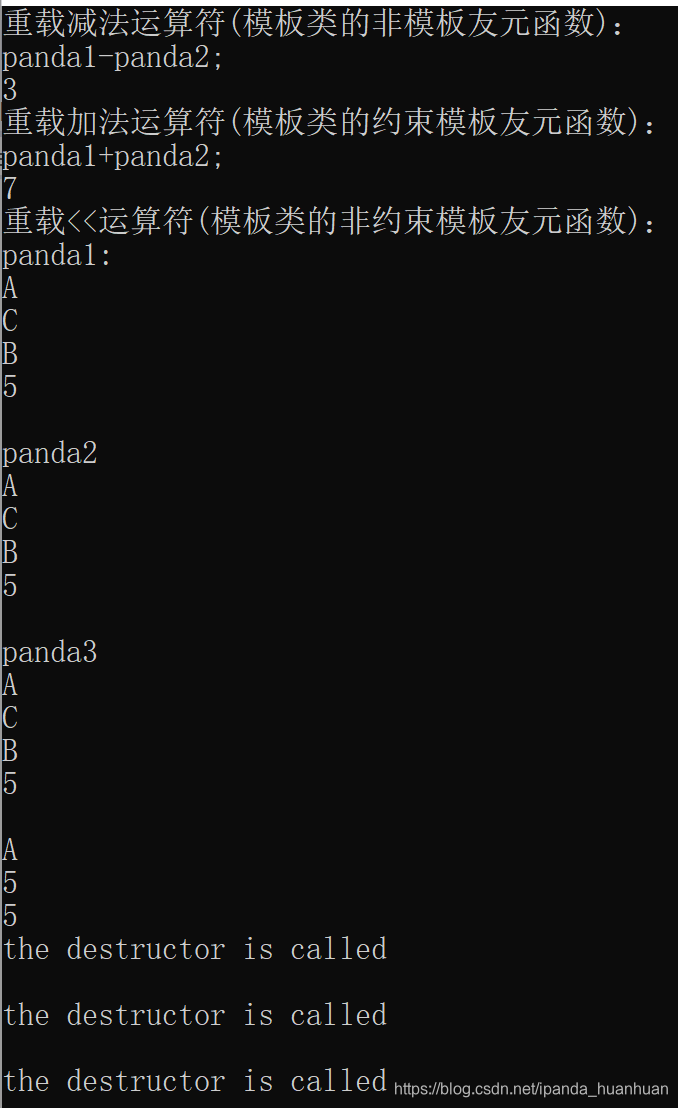