排序算法
如果不太了解各种排序算法可以参考菜鸟教程(希望对你有帮助)
题目一
题目描述
定义包含学号、姓名和成绩的学生信息结构类型,完成以下功能:
(1) 键盘输入n个学生信息数据(n从键盘输入);
(2)使用“冒泡法”对学生成绩由高到低排序;
(3)输出排序后学生信息。
输入输出样例如下,数据间以空格分隔。
输入样例:
3
100001 wang-li 91
100002 chen-da-wei 95
100003 guo-tao 93
输出样例:
100002 chen-da-wei 95
100003 guo-tao 93
100001 wang-li 91
题解
#include <stdio.h>
#include <stdlib.h>
#include <memory.h>
#define MAX 50
typedef struct {
char stuId[10];
char name[20];
int score;
}Student;
void input(Student students[MAX], int n)
{
for ( int i = 0; i < n; i++) {
scanf("%s", students[i].stuId);
scanf("%s", students[i].name);
scanf("%d", &students[i].score);
}
}
void sort(Student students[MAX], int n)
{
Student temp;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (students[j].score < students[j + 1].score) {
memcpy(&temp, &students[j], sizeof(Student));// void *memcpy(void *str1, const void *str2, size_t n) 从存储区 str2 复制 n 个字符到存储区 str1
memcpy(&students[j], &students[j + 1], sizeof(Student));
memcpy(&students[j + 1], &temp, sizeof(Student));
}
}
}
}
题目二
题目描述
从键盘输入奇数个整型数(大于3),对该整数序列按如下规则排序:最大值排在中间,最小值排在最左,中值排在最右,其它值清为0。
输以空格隔开
定义排序函数原型为:void booble(int *array,int n);
运行样例如下所示:
输入
5
1 2 3 4 5
输出
1 0 5 0 3
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
/*冒泡排序法*/
void booble(int* array, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (array[j]>array[j+1]) {
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
int t = (n - 1) / 2;//t即为中间是的数的序号
/*交换中间的数和最大的数的位置*/
int temp = array[t];
array[t] = array[n-1];
array[n - 1] = temp;
/*除中间数和最大最小数全都赋值为0*/
for (size_t i = 1; i < n-1; i++)
{
if (i!=t)
{
array[i] = 0;
}
}
}
int main() {
int n;
scanf("%d", &n);
int *description = (int*)malloc(n * sizeof(int));
for (size_t i = 0; i < n; i++)
{
scanf("%d", (description+i));
}
booble(description, n);
for (size_t i = 0; i < n-1; i++)
{
printf("%d ", *(description + i));
}
printf("%d", *(description+n-1));
}
题目三
题目描述
编写折半查找函数,函数原型:int Binserach(int *a,int n,int key);
功能:在n个元素的数组a中寻找key,若找到则返回key在数组中的下标,否则返回-1。
已知有排好序的整数数组:
int a[]={1,2,3,4,5,6,7,8,9,10,11,12,13,14,15};
在main函数中,使用折半查找函数,输入一个整数,查找是否在数组中,如在给出下标,否则-1
输入样例:
9
输出样例:
8
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
int Binserach(int* a, int n, int key) {
for (size_t i = 0; i <n; i++)
{
if (a[i] == key) return i;
}
return -1;
}
int main() {
int a[] = { 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15 };
int key;
scanf("%d", &key);
printf("%d",Binserach(a, 15, key));
}
题目四
题目描述
编写冒泡排序函数,函数原型:void BubbleSort(int a[],int n);对n个整数升序排序。
编写选择排序函数,函数原型:void SelectionSort(int a[],int n);对n个整数降序排序。
编写main函数,输入10个整数,利用函数将其按升序和降序分别输出排序结果。(输入输出数据间用一个空格分隔)
输入样例:
5 2 8 9 10 1 3 4 7 6
输出样例:
1 2 3 4 5 6 7 8 9 10
10 9 8 7 6 5 4 3 2 1
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
void bubble_sort(int arr[], int len) {
int i, j, temp;
for (i = 0; i < len - 1; i++)
for (j = 0; j < len - 1 - i; j++)
if (arr[j] > arr[j + 1]) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
void swap(int* a, int* b) //交換兩個變數
{
int temp = *a;
*a = *b;
*b = temp;
}
void selection_sort(int arr[], int len)
{
int i, j;
for (i = 0; i < len - 1; i++)
{
int max = i;
for (j = i + 1; j < len; j++) //走訪未排序的元素
if (arr[j] > arr[max]) //找到目前最大值
max = j; //紀錄最大值
swap(&arr[max], &arr[i]); //做交換
}
}
int main() {
int a[10];
for (size_t i = 0; i < 10; i++)
{
scanf("%d", &a[i]);
}
bubble_sort(a, 10);
for (size_t i = 0; i < 9; i++)
{
printf("%d ", a[i]);
}
printf("%d\n", a[9]);
selection_sort(a, 10);
for (size_t i = 0; i < 9; i++)
{
printf("%d ", a[i]);
}
printf("%d\n", a[9]);
}
题目五
题目描述
输入一行数字,如果我们把这行数字中的‘5’都看成空格,那么就得到一行用空格分割的若干非负整数(可能有些整数以‘0’开头,这些头部的‘0’应该被忽略掉,除非这个整数就是由若干个‘0’组成的,这时这个整数就是0)。你的任务是:对这些分割得到的整数,依从小到大的顺序排序输出。
输入格式:每组输入数据只有一行数字(数字之间没有空格),这行数字的长度不大于1000。输入数据保证:分割得到的非负整数不会大于100000000;输入数据不可能全由‘5’组成。
输出格式:对于每个测试用例,输出分割得到的整数排序的结果,相邻的两个整数之间用一个空格分开,每组输出占一行。
输入样例:
0051231232050775
输出样例:
0 77 12312320
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<string.h>
int a[120]; //记录分离的数据;
char b[1000];//记录总数据;
void fun1(int* p, int m)//排序并输出
{
int i, j, k;
for (i = 0; i < m; i++)
{
for (j = 0; j < m; j++)
{
if (a[i] < a[j])
{
k = a[i];
a[i] = a[j];
a[j] = k;
}
}
}
for (i = 0; i < m; i++){
printf("%d", a[i]);
if (i != m - 1) printf(" ");
}
}
//split ()//分离函数;
void fun2(char* b)
{
int i, j = 0;
int sum = 0;
for (i = 0; i < strlen(b); i++)
{
if (b[i] != '5')
{
sum = sum * 10 + (b[i] - '0');
}
else
{
if (b[i - 1] != '5' && i != 0)
{
a[j] = sum;
j++;
sum = 0;
}
}
}
if (b[i - 1] == '5') fun1(a, j);
else
{
a[j] = sum;
fun1(a, j + 1);
}
}
int main()
{
gets(b);
fun2(b);
return 0;
}
题目六
题目描述
改进冒泡排序程序,使其当数据已经有序时,直接结束排序过程。函数原型:
void Bubble(int *a,int n);//对n个整数从小到大排序
在主函数中调用Bubble对键盘输入的m个整数进行排序并输出。其中m个元素的整型数组p可以动态申请,
如 int *p=(int *)calloc(m,sizeof(int));
测试样例如下:
输入样例:
10
87 66 92 85 62 77 95 100 89 73
输出样例:
62 66 73 77 85 87 89 92 95 100
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
void bubble_sort(int arr[], int len) {
int i, j, temp,k;//比冒泡排序法多一个k变量用于判断是否已经排好序
for (i = 0; i < len - 1; i++) {
k = 1;
for (j = 0; j < len - 1 - i; j++)
if (arr[j] > arr[j + 1]) {
k = 0;
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
if (k)
{
break;
}
}
}
int main() {
int n;
scanf("%d", &n);
int* description = (int*)malloc(n * sizeof(int));
for (size_t i = 0; i < n; i++)
{
scanf("%d", (description + i));
}
bubble_sort(description, n);
for ( int i = 0; i < n-1; i++)
printf("%d ", *(description + i));
printf("%d",*(description+n-1));
return 0;
}
题目七
题目描述
输入数组a( 长度为n, n<10),对元素按由小到大顺序排列,然后再输入一个数b插入数组a中,插入后,数组a中的元素仍然由小到大顺序排列。
输入(第一行数组大小n,第二行为输入的数组,第三行为插入的整数):
5
5 2 3 4 8
7
输出:
2 3 4 5 7 8
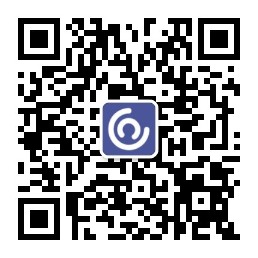
题解
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
void bubble_sort(int arr[], int len) {
int i, j, temp,k;
for (i = 0; i < len - 1; i++) {
k = 1;
for (j = 0; j < len - 1 - i; j++)
if (arr[j] > arr[j + 1]) {
k = 0;
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
if (k)
{
break;
}
}
}
int main() {
int n,m;
scanf("%d", &n);
int* description = (int*)malloc((n+1) * sizeof(int));
for (size_t i = 0; i < n; i++)
{
scanf("%d", (description + i));
}
scanf("%d", &m);
description[n] = m;
bubble_sort(description, n + 1);
for (size_t i = 0; i < n; i++)
{
printf("%d ", *(description + i));
}
printf("%d", *(description + n));
}