df1<-data.frame(id=c(1,2,3,4), value=c(10,20,30,40))
df2<-data.frame(col1=c(1,2,3,4), col2=c(4,3,2,1))
df2[] <- lapply(df2, function(x) { inds <- match(x, df1$id) ifelse(is.na(inds),x, df1$value[inds]) })
df2
================================================
df <- data.frame(fruits = c("apple", "orange", "pineapple", "banana", "grape"), stringsAsFactors = FALSE)
df_rep <- data.frame(eng = c("apple", "orange", "grape"), esp = c("manzana", "naranja", "uva"), stringsAsFactors = FALSE)
df2 <- merge(df, df_rep, by.x = 'fruits', by.y = 'eng', all.x = TRUE) df2$fruits <- ifelse(is.na(df2$esp), df2$fruits, df2$esp)
================================================
a <- data.frame(id=letters[1:4], age=c(18,NA,9,NA), sex=c("M","F","F","M"))
a
b <- data.frame(id=c("a","b","d"), age=c(18,32,20))
b
library(data.table) setDT(a)[b, agei := i.age, on='id'][is.na(age), age := agei][,agei:= NULL][] a
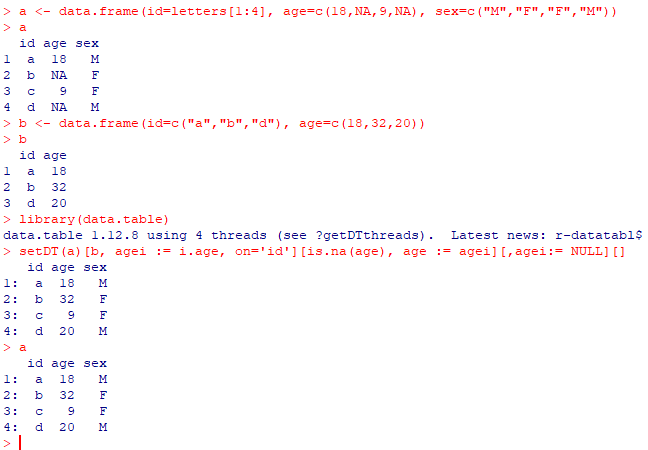
================================================
library(dplyr)
dt1 <- read.table(text = "
SAMPLE_ID CLASS_ID CLASS VALUE
1 0 0 5
2 0 0 5
3 0 0 3
4 0 0 6
5 0 0 6
6 0 0 3
", header = TRUE, stringsAsFactors = FALSE)
dt2 <- read.table(text = "
SAMPLE_ID REF_VAL CLASS_ID CLASS
1 33 2 cloud
2 45 3 water
3 NA 3 water
4 NA 4 forest
", header = TRUE, stringsAsFactors = FALSE)
dt3 <- read.table(text = "
SAMPLE_ID CLASS_ID CLASS STRATA
5 3 NA 20
6 3 water 19
", header = TRUE, stringsAsFactors = FALSE)
dt <- dt1[,c("SAMPLE_ID", "VALUE")]
dt <- left_join(dt, dplyr::bind_rows(dt2, dt3))
dt <- select(dt, SAMPLE_ID, CLASS_ID, CLASS, VALUE)
================================================
REF
https://stackoverflow.com/questions/51614901/r-conditional-replacement-using-two-data-frames
https://stackoverflow.com/questions/33954292/merge-two-data-frame-and-replace-the-na-value-in-r
https://stackoverflow.com/questions/29809512/replace-values-between-two-data-frames-in-r