===============================
爬取百度网粤港澳大湾区7个机场的新闻数据并存入MySQL数据库中
===============================
一、获取网页源代码
1.引入所需库
import requests
import re
import pymysql
import time
2.请求头
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/69.0.3497.100 Safari/537.36'}
3.建立挖取某个机场新闻的函数
def baidu(company):
url = 'https://www.baidu.com/s?ie=utf-8&medium=1&rtt=4&bsst=1&rsv_dl=news_b_pn&cl=2&wd=' + company
res = requests.get(url, headers=headers).text
二、提取信息并清洗数据
1.提取源代码中所需的网址、标题、新闻来源和发布日期的正则表达式
p_href = '<h3 class="news-title_1YtI1"><a href="(.*?)"'
href = re.findall(p_href, res, re.S)
p_title = '<h3 class="news-title_1YtI1"><.*?>(.*?)<!--/s-text--></a></h3>'
title = re.findall(p_title, res, re.S)
p_date = '<span class="c-color-gray2 c-font-normal">(.*?)</span>'
date = re.findall(p_date, res, re.S)
p_source = '<span class="c-color-gray c-font-normal c-gap-right">(.*?)</span>'
source = re.findall(p_source, res, re.S)
2.清洗标题及发布日期中的干扰信息
for i in range(len(href)):
title[i] = title[i].strip()
title[i] = re.sub('<.*?>', '', title[i])
date[i] = date[i].split(' ')[0]
date[i] = re.sub('年', '-', date[i])
date[i] = re.sub('月', '-', date[i])
date[i] = re.sub('日', '', date[i])
if ('小时' in date[i]) or ('分钟' in date[i]):
date[i] = time.strftime("%Y-%m-%d")
else:
date[i] = date[i]
三、将数据存入MySQL数据库
需要首先在MySQL中建立一个pachong的文件夹,文件夹下面建立一个test文档。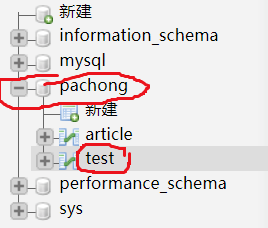
文档里面要建立如下5个项,类型,排序规则都请见下图
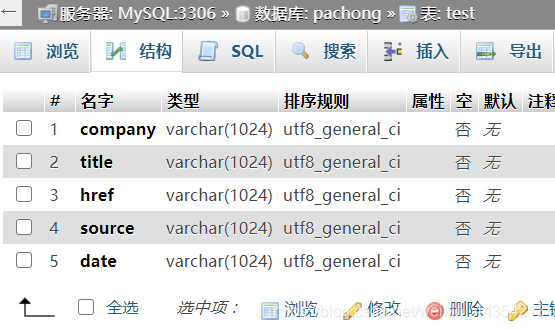
for i in range(len(title)):
db = pymysql.connect(host='localhost', port=3306, user='root', password='', database='pachong', charset='utf8')
cur = db.cursor()
sql = 'INSERT INTO test(company,title,href,source,date) VALUES (%s,%s,%s,%s,%s)'
cur.execute(sql, (company, title[i], href[i], source[i], date[i]))
db.commit()
cur.close()
db.close()
四、批量爬取7个机场数据并存入数据库,可以采用如下代码:
companys = ['广州白云机场', '深圳宝安机场','惠州平潭机场', '佛山沙堤机场', '珠海金湾机场', '香港国际机场', '澳门国际机场']
for company in companys:
baidu(company)
五、最终效果
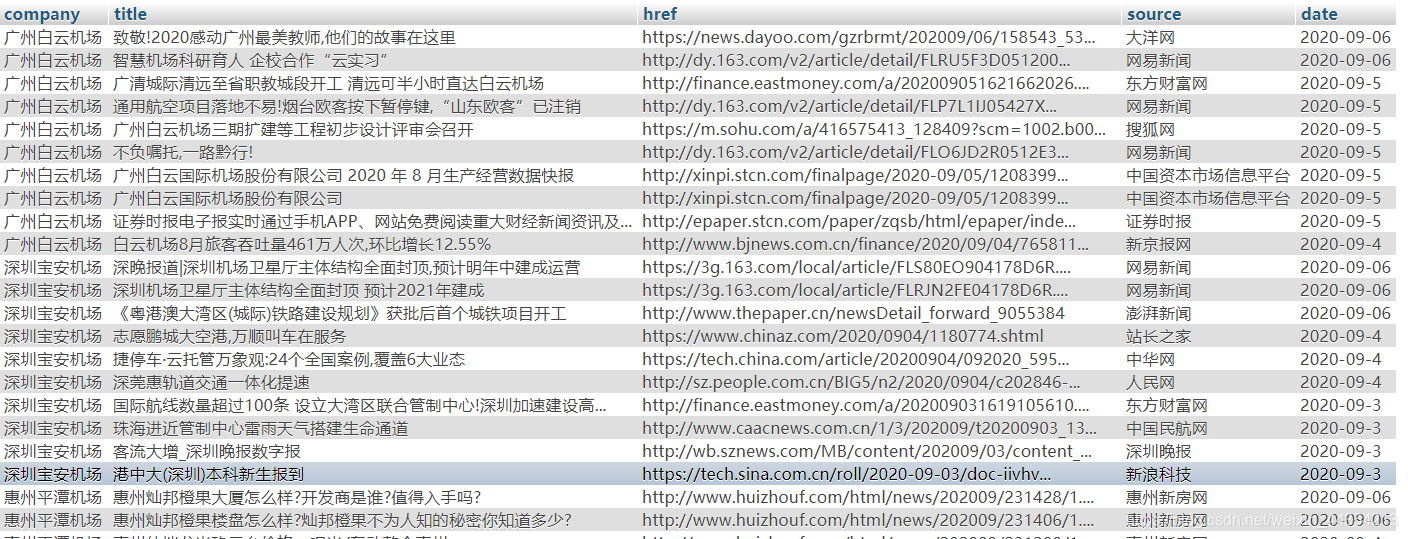
附上所有代码
# =============================================================================
# 爬取百度网粤港澳大湾区7个机场的新闻数据并存入MySQL数据库中
# =============================================================================
# 一、获取网页源代码
# 1.引入所需库
import requests
import re
import pymysql
import time
# 2.请求头
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/69.0.3497.100 Safari/537.36'}
# 3.建立挖取某页新闻的函数
def baidu(company):
url = 'https://www.baidu.com/s?ie=utf-8&medium=1&rtt=4&bsst=1&rsv_dl=news_b_pn&cl=2&wd=' + company
res = requests.get(url, headers=headers).text
# 二、提取信息并清洗数据
# 1.提取源代码中所需的网址、标题、新闻来源和发布日期的正则表达式
p_href = '<h3 class="news-title_1YtI1"><a href="(.*?)"'
href = re.findall(p_href, res, re.S)
p_title = '<h3 class="news-title_1YtI1"><.*?>(.*?)<!--/s-text--></a></h3>'
title = re.findall(p_title, res, re.S)
p_date = '<span class="c-color-gray2 c-font-normal">(.*?)</span>'
date = re.findall(p_date, res, re.S)
p_source = '<span class="c-color-gray c-font-normal c-gap-right">(.*?)</span>'
source = re.findall(p_source, res, re.S)
# 2.清洗标题及发布日期中的干扰信息
for i in range(len(href)):
title[i] = title[i].strip()
title[i] = re.sub('<.*?>', '', title[i])
date[i] = date[i].split(' ')[0]
date[i] = re.sub('年', '-', date[i])
date[i] = re.sub('月', '-', date[i])
date[i] = re.sub('日', '', date[i])
if ('小时' in date[i]) or ('分钟' in date[i]):
date[i] = time.strftime("%Y-%m-%d")
else:
date[i] = date[i]
# 三、将数据存入MySQL数据库
for i in range(len(title)):
db = pymysql.connect(host='localhost', port=3306, user='root', password='', database='pachong', charset='utf8')
cur = db.cursor()
sql = 'INSERT INTO test(company,title,href,source,date) VALUES (%s,%s,%s,%s,%s)'
cur.execute(sql, (company, title[i], href[i], source[i], date[i]))
db.commit()
cur.close()
db.close()
# 四、批量爬取7个机场数据并存入数据库,可以采用如下代码:
companys = ['广州白云机场', '深圳宝安机场','惠州平潭机场', '佛山沙堤机场', '珠海金湾机场', '香港国际机场', '澳门国际机场']
for company in companys:
baidu(company)