效果展示
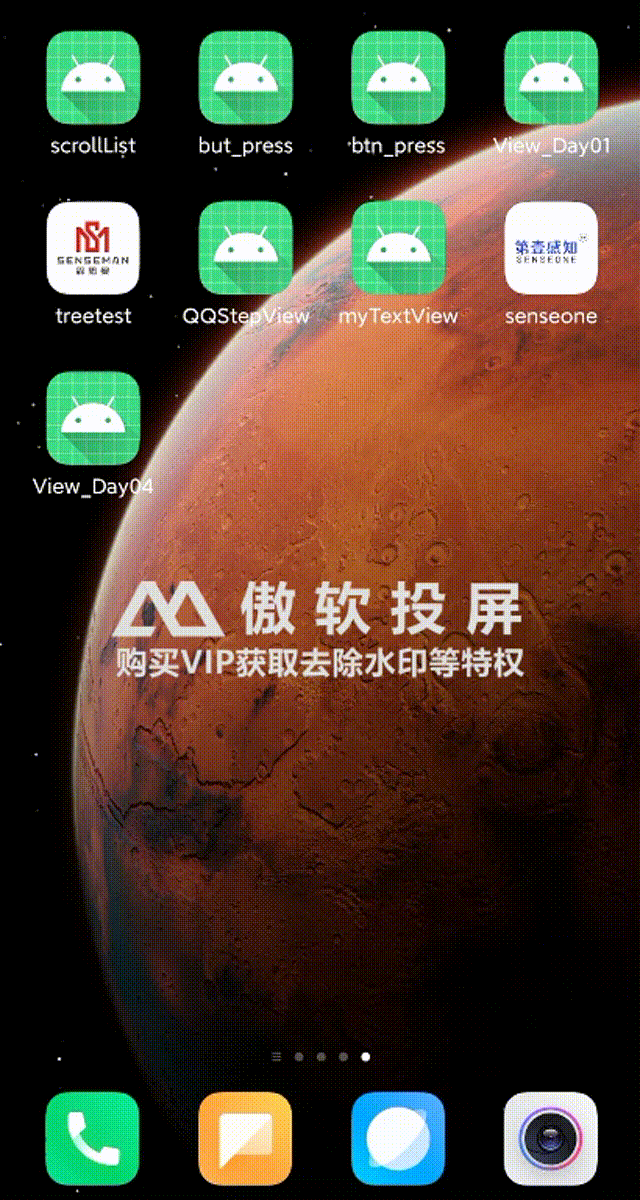
思路分析
1、固定不动蓝色的大圆弧 color borderWidth
2、可以变化的小圆弧(红色) color borderWidth
3、中间的步数文字 color textSize
这里,因为内外圆弧宽度宽度一样,所以,可统一命名为 borderWidth
开始码字
第一步:自定义控件类的创建 继承自 View
第二步:自定义属性文件的创建,及内容的编写
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="QQStepView">
<attr name="outerColor" format="color"/>
<attr name="innerColor" format="color"/>
<attr name="borderWidth" format="dimension"/>
<attr name="stepTextSize" format="dimension"/>
<attr name="stepTextColor" format="color"/>
</declare-styleable>
</resources>
如何创建自定义属性文件,我前面有讲解 ,不懂得可自行复习
第三步:在布局文件中应用
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.example.qqstepview.QQStepView
android:id="@+id/step_view"
android:background="@color/colorAccent"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:outerColor="@color/colorPrimaryDark"
app:innerColor="#f00"
app:borderWidth="20dp"
app:stepTextColor="#f00"
app:stepTextSize="50sp"/>
</RelativeLayout>
第四步:在自定义组件类 中 获取自定义属性 并编写相关方法
package com.example.qqstepview;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Rect;
import android.graphics.RectF;
import android.util.AttributeSet;
import android.view.View;
import androidx.annotation.Nullable;
public class QQStepView extends View {
private int mOuterColor = Color.parseColor("#3700B3");
private int mInnerColor = Color.parseColor("#ff0000");
private int mBorderWidth = 20; //20px
private int mStepTextSize = 14; //14px
private int mStepTextColor;
private Paint mOutPaint,mInnerPaint,mTextPaint;
private Context mContext;
//总共的,当前的步数
private int mStepMax = 100;
private int mCurrentStep = 50;
public QQStepView(Context context) {
this(context,null);
}
public QQStepView(Context context, @Nullable AttributeSet attrs) {
this(context, attrs,0);
}
public QQStepView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
this.mContext = context;
//1、分析效果 2、确定自定义属性 编写attrs.xml 3、在布局中使用 4、在自定义View中获取自定义属性
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.QQStepView);
mOuterColor = typedArray.getColor(R.styleable.QQStepView_outerColor, mOuterColor);
mInnerColor = typedArray.getColor(R.styleable.QQStepView_innerColor, mInnerColor);
mBorderWidth = (int) typedArray.getDimension(R.styleable.QQStepView_borderWidth,mBorderWidth);
mStepTextSize = typedArray.getDimensionPixelSize(R.styleable.QQStepView_stepTextSize,mStepTextSize);
mStepTextColor = typedArray.getColor(R.styleable.QQStepView_stepTextColor,mStepTextColor);
typedArray.recycle();
//初始化数据
initData();
//5、onMeasure()
//6、画外圆弧 内圆弧 文字
//7、其他
}
private void initData() {
mOutPaint = new Paint();
mOutPaint.setAntiAlias(true);
mOutPaint.setStrokeWidth(mBorderWidth);
mOutPaint.setColor(mOuterColor);
mOutPaint.setStyle(Paint.Style.STROKE);
mOutPaint.setStrokeCap(Paint.Cap.ROUND);
mInnerPaint = new Paint();
mInnerPaint.setAntiAlias(true);
mInnerPaint.setStrokeWidth(mBorderWidth);
mInnerPaint.setColor(mInnerColor);
mInnerPaint.setStyle(Paint.Style.STROKE);
mInnerPaint.setStrokeCap(Paint.Cap.ROUND);
mTextPaint = new Paint();
mTextPaint.setAntiAlias(true);
mTextPaint.setTextSize(mStepTextSize);
mTextPaint.setColor(mStepTextColor);
}
//5、onMeasure()
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
//调用者在布局文件中可能 wrap_content 宽度高度不一致
int width = MeasureSpec.getSize(widthMeasureSpec) + getPaddingLeft() + getPaddingRight();
int height = MeasureSpec.getSize(heightMeasureSpec) + getPaddingTop() +getPaddingBottom();
int widthMode = MeasureSpec.getMode(widthMeasureSpec);
int heightMode = MeasureSpec.getMode(heightMeasureSpec);
if (widthMode == MeasureSpec.AT_MOST){
}
if (heightMode == MeasureSpec.AT_MOST){
}
setMeasuredDimension(width>height?height:width,width>height?height:width);
}
//6、画外圆弧 内圆弧 文字
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int center = getWidth()/2;
int radius = getWidth()/2 - mBorderWidth/2;
//6.1 画外圆弧
RectF rectF = new RectF(center - radius ,
center - radius ,
center + radius ,
center + radius ); //第一种写法
//RectF rectF = new RectF(mBorderWidth/2,mBorderWidth/2,getWidth() - mBorderWidth/2,getWidth() - mBorderWidth/2); //第二种写法
canvas.drawArc(rectF,135,270,false,mOutPaint);
//6.2 画内圆弧 怎么画肯定不能写死 百分比 是使用者设置的 从外面传
System.out.println("mCurrentStep:"+mCurrentStep+"mStepMax:"+mStepMax);
if (mStepMax == 0)return;
float sweepAngle = (float)mCurrentStep/mStepMax;
canvas.drawArc(rectF,135,sweepAngle*270,false,mInnerPaint);
//6.3 画文字
String stepText = mCurrentStep + "";
//基线
Paint.FontMetricsInt fontMetricsInt = mTextPaint.getFontMetricsInt();
int dy = (fontMetricsInt.bottom - fontMetricsInt.top)/2 - fontMetricsInt.bottom;
int baseLine = getHeight()/2 + dy;
//文字起始位置
Rect textBounds = new Rect();
mTextPaint.getTextBounds(stepText,0,stepText.length(),textBounds);
int dx = textBounds.width();
float x = getWidth()/2 - dx/2; //dx表示文字宽度
canvas.drawText(stepText,x,baseLine,mTextPaint);
}
//7,其他,写几个方法动起来
public synchronized void setStepMax (int stepMax){
this.mStepMax = stepMax;
}
public synchronized void setCurrentStep (int currentStep){
this.mCurrentStep = currentStep;
//不断绘制
invalidate();
}
}
里面有一些冗余代码,比如初始化画笔可以封装,我就不在此优化了
第五步:在使用了自定义组件的 类中 调用
package com.example.qqstepview;
import androidx.appcompat.app.AppCompatActivity;
import android.animation.ObjectAnimator;
import android.animation.ValueAnimator;
import android.os.Bundle;
import android.view.View;
import android.view.animation.AccelerateDecelerateInterpolator;
import android.view.animation.BounceInterpolator;
import android.view.animation.DecelerateInterpolator;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final QQStepView step_view = findViewById(R.id.step_view);
step_view.setStepMax(4000);
//值动画
ValueAnimator valueAnimator = ObjectAnimator.ofFloat(0, 3000);
valueAnimator.setDuration(1000);
valueAnimator.setInterpolator(new DecelerateInterpolator());
valueAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
float currentStep = (float) animation.getAnimatedValue();
step_view.setCurrentStep((int) currentStep);
}
});
valueAnimator.start();
}
}
源码连接:QQStepView