1100 Mars Numbers (20 分)
People on Mars count their numbers with base 13:
- Zero on Earth is called “tret” on Mars.
- The numbers 1 to 12 on Earth is called “jan, feb, mar, apr, may, jun,
jly, aug, sep, oct, nov, dec” on Mars, respectively. - For the next higher digit, Mars people name the 12 numbers as “tam,
hel, maa, huh, tou, kes, hei, elo, syy, lok, mer, jou”, respectively.
For examples, the number 29 on Earth is called “hel mar” on Mars; and “elo nov” on Mars corresponds to 115 on Earth. In order to help communication between people from these two planets, you are supposed to write a program for mutual translation between Earth and Mars number systems.
Input Specification:
Each input file contains one test case. For each case, the first line contains a positive integer N (<100). Then N lines follow, each contains a number in [0, 169), given either in the form of an Earth number, or that of Mars.
Output Specification:
For each number, print in a line the corresponding number in the other language.
Sample Input:
4
29
5
elo nov
tam
Sample Output:
hel mar
may
115
13
作者:CHEN, Yue
单位:浙江大学
代码长度限制:16 KB
时间限制:400 ms
内存限制:64 MB
题目大意
就是他给你几个数字或这单词,你需要判断一下,如果给你的是十进制数字,你需要转换成十三进制对应的单词;如果给你的是十三进制的单词,需要转换成十进制的数字。
解题思路
- 首先新建数组
ls1
存储每一行给定的数值。 - 遍历一下,判断是否是数字。
- 如果是十进制数字,判断是否小于13,若小于13,则直接输出;否则需要加上一个13的倍数对应的单词。
- 如果是十三进制单词,判断是几个单词,若是一个单词,判断时候是0,如果不是0,打印单词对应的数值;
- 若是两个单词,需要一个变量来存储数值,先把一个单词对应的数值放入到变量中,然后再把第二个单词对应的数字放入到变量中,最后打印变量的值。
Code
n = int(input())
dic1 = ["tret","jan", "feb", "mar", "apr", "may", "jun", "jly", "aug", "sep", "oct", "nov", "dec"] #0-12数字
dic2 = ["tam", "hel", "maa", "huh", "tou", "kes", "hei", "elo", "syy", "lok", "mer", "jou"] #13的倍数(最大为169)
ls1 = []
for i in range(n):
ls1.append(input()) #求出每行给定的数值
for i in range(len(ls1)):
if ls1[i].isdigit() == True: #给定的数字是10进制数字
if int(ls1[i]) < 13:
print(dic1[int(ls1[i])])
elif int(ls1[i]) >= 13 and int(ls1[i]) < 169:
b = int(ls1[i])
b1 = int(b//13)
b2 = b % 13
if b2 == 0:
print('%s'%(dic2[b1-1]))
elif b2 != 0:
print('{} {}'.format(dic2[b1-1],dic1[b2]))
else: #给定的数字是13进制的数字
if len(ls1[i]) == 3:
for h in range(13):
if dic1[h] == ls1[i][:3]:
print(h)
break
for j in range(12):
if dic2[j] == ls1[i][:3]:
print((j+1) * 13)
break
if len(ls1[i]) == 7:
sum = 0
for c in range(12):
if dic2[c] == ls1[i][0:3]:
sum = (c+1) * 13
break
for h in range(13):
if dic1[h] == ls1[i][4:7]:
sum += h
break
print(sum)
if len(ls1[i]) == 4: #判断是否是0的情况
print('0')
运行结果
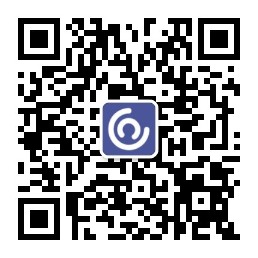