1. 商品修改页面绘制
1.1 编辑页面HTML
<!-- 定义商品修改的对话框 -->
<!-- 定义商品修改的对话框 -->
<el-dialog title="商品修改" :visible.sync="updateDialogVisible" width="60%">
<!-- 准备修改的表单-->
<el-form :model="updateItem" :rules="rules" ref="ruleForm" label-width="100px" class="demo-ruleForm">
<el-form-item label="标题信息" prop="title">
<el-input v-model="updateItem.title"></el-input>
</el-form-item>
<el-form-item label="卖点信息" prop="sellPoint">
<el-input v-model="updateItem.sellPoint"></el-input>
</el-form-item>
<el-form-item label="价格信息" prop="price">
<el-input v-model="updateItem.price"></el-input>
</el-form-item>
<el-form-item label="数量信息" prop="num">
<el-input v-model="updateItem.num"></el-input>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="updateDialogVisible = false">取 消</el-button>
<el-button type="primary" @click="dialogVisible = false">确 定</el-button>
</span>
</el-dialog>
updateDialogVisible: false,
updateItem: {
},
rules: {
title: [
{
required: true, message: '请输入商品标题信息', trigger: 'blur' },
{
min: 3, max: 50, message: '长度在 3 到 50 个字符', trigger: 'blur' }
],
sellPoint: [
{
required: true, message: '请输入商品卖点信息', trigger: 'blur' },
{
min: 3, max: 50, message: '长度在 3 到 50 个字符', trigger: 'blur' }
],
price: [
{
required: true, message: '请输入商品价格信息', trigger: 'blur' },
{
min: 3, max: 50, message: '长度在 3 到 50 个字符', trigger: 'blur' }
],
num: [
{
required: true, message: '请输入商品数量信息', trigger: 'blur' },
{
min: 3, max: 50, message: '长度在 3 到 50 个字符', trigger: 'blur' }
],
}
updateItemBtn(item){
console.log("扩展案例,自己实现 只需要修改 标题/卖点/价格/数量")
this.updateDialogVisible = true
this.updateItem = item
this.updateItem.price = (this.updateItem.price / 100).toFixed(2)
}
2. 实现商品图片上传
2.1 编辑页面
2.1.1 官网说明
<!-- 图片上传的JS
1. action: 代表图片上传的地址url
2. file-list: 图片列表数据的集合[{
name:"xx",url:"xxx"},{
}]
3. 钩子函数: 满足某些条件时触发.
4. on-preview 当点击已上传列表的信息时触发
5. on-remove 当移除列表中的图片时触发
-->
<el-upload
class="upload-demo"
action="https://jsonplaceholder.typicode.com/posts/"
:on-preview="handlePreview"
:on-remove="handleRemove"
:file-list="fileList"
list-type="picture">
<el-button size="small" type="primary">点击上传</el-button>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
2. 页面JS补充知识
handlePreview(){
console.log("触发查看函数!!!!")
},
handleRemove(){
console.log("移除时触发!!!!")
}
2.1.2 图片上传项目说明
<!--
一.文件上传组件说明
1.action: 上传图片地址 http://localhost:8091/xxx/xxx
2.on-preview 点击图片时触发
3.on-remove 移除图片时触发
4.on-success 图片上传成功时触发
5.multiple 可以支持多张图片上传
6.drag 是否允许拖拽
二.请求类型: 一般上传字节信息时,首选post请求
三.上传文件key 说明: 文件上传时的key=file.
后端接收数据时采用file接收.
-->
<el-upload class="upload-demo" :action="uploadUrl" :on-preview="handlePreview" :on-remove="handleRemove"
:on-success="handleSuccess" list-type="picture" multiple drag>
<el-button size="small" type="primary">点击上传</el-button>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
uploadUrl: "http://localhost:8091/file/upload",
2.1.3 图片上传接口文档说明
- 请求路径: http://localhost:8091/file/upload
- 请求类型: post
- 请求参数:
参数名称 |
参数说明 |
备注 |
file |
文件上传的参数名称 |
file中携带的是二进制信息 |
参数名称 |
参数说明 |
备注 |
status |
状态信息 |
200表示服务器请求成功 |
msg |
服务器返回的提示信息 |
可以为null |
data |
服务器返回的业务数据 |
返回ImageVO对象 |
参数名称 |
参数类型 |
参数说明 |
备注 |
virtualPath |
String |
图片实际路径 不包含磁盘信息 |
例如: 2021/11/11/a.jpg 不需要写磁盘地址 |
urlPath |
String |
图片url访问地址 |
http://image.jt.com/2021/11/11/a.jpg 需要指定域名地址 |
fileName |
String |
文件上传后的文件名称 |
UUID.type |
2.1.4 编辑ImageVO
@Data
@Accessors(chain = true)
@NoArgsConstructor
@AllArgsConstructor
public class ImageVO {
private String virtualPath;
private String urlPath;
private String fileName;
}
2.1.5 编辑ItemController
@RestController
@CrossOrigin
@RequestMapping("/file")
public class FileController {
@PostMapping("/upload")
public SysResult upload(MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
String dirPath = "E:/project3/images/";
File dirFile = new File(dirPath);
if(!dirFile.exists()){
dirFile.mkdirs();
}
String path = dirPath + fileName;
File allFile = new File(path);
file.transferTo(allFile);
return SysResult.success();
}
}
2.2 正则表达式(复习)
2.2.1 正则表达式说明
正则表达式,又称规则表达式。(英语:Regular Expression,在代码中常简写为regex、regexp或RE),计算机科学的一个概念。正则表达式通常被用来检索、替换那些符合某个模式(规则)的文本。
总结: 正则表达式就是一种特殊格式的字符串.校验文本信息的.
2.2.2 匹配不确定次数
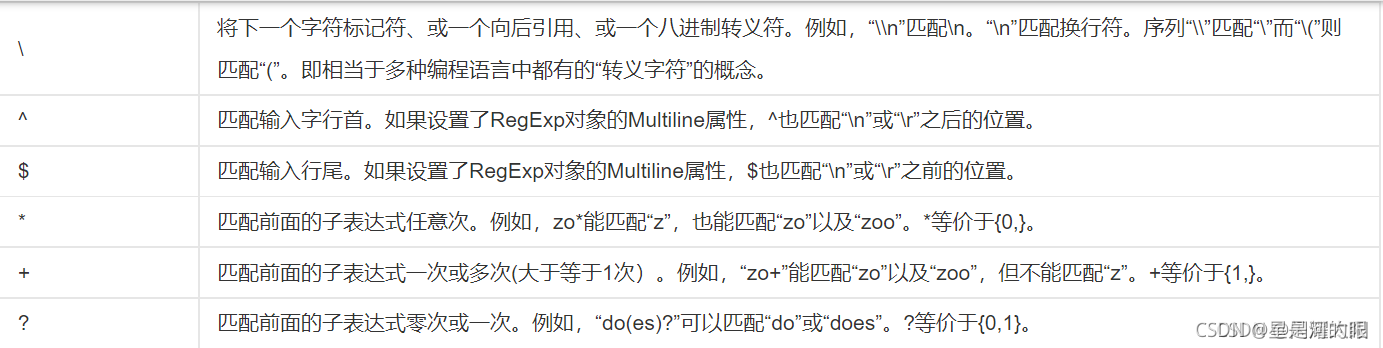
2.2.3 匹配固定次数
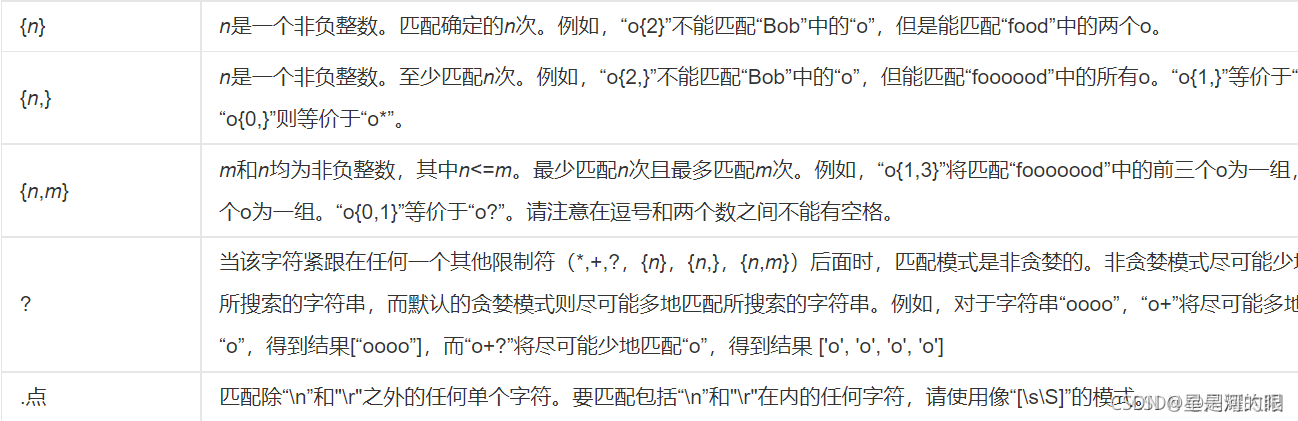
2.2.4 匹配取值区间
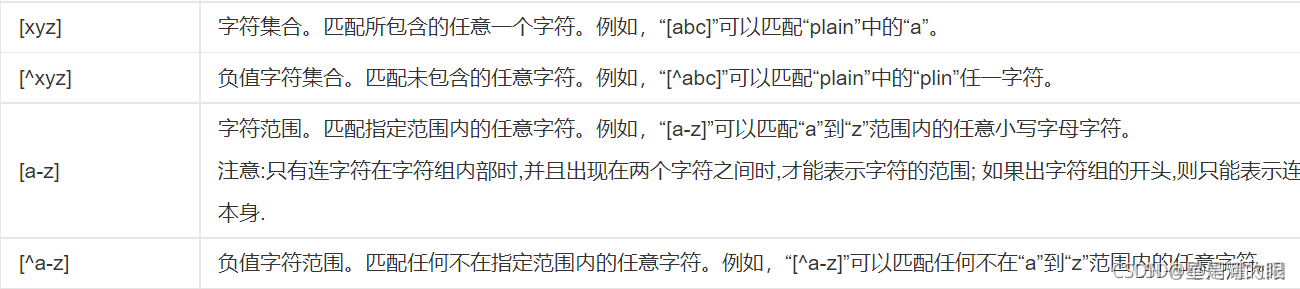
2.2.5 分组匹配
(jpg|png|gif)

2.2.6 正则案例练习
1.要求匹配电话号码 11位 开头都是1
正则表达式: 1[3-9][0-9]{9}
2.要求匹配邮箱 [email protected]
正则表达式:
^[a-zA-Z0-9-_]+@[a-zA-Z0-9-_]+\.[a-zA-Z0-9-_]+$
2.3 文件上传实现
2.3.1 编辑FileController
@RestController
@CrossOrigin
@RequestMapping("/file")
public class FileController {
@Autowired
private FileService fileService;
@PostMapping("/upload")
public SysResult upload(MultipartFile file) throws IOException {
ImageVO imageVO = fileService.upload(file);
if(imageVO == null){
return SysResult.fail();
}
return SysResult.success(imageVO);
}
}
2.3.2 编辑FileService
package com.jt.service;
import com.jt.vo.ImageVO;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.net.FileNameMap;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Service
public class FileServiceImpl implements FileService{
private String localDirPath = "E:/project3/images";
@Override
public ImageVO upload(MultipartFile file) {
String fileName = file.getOriginalFilename();
fileName = fileName.toLowerCase();
if(!fileName.matches("^.+\\.(jpg|png|gif)$")){
return null;
}
try {
BufferedImage bufferedImage = ImageIO.read(file.getInputStream());
int height = bufferedImage.getHeight();
int width = bufferedImage.getWidth();
if(height == 0 || width == 0){
return null;
}
String dateDir = new SimpleDateFormat("/yyyy/MM/dd/")
.format(new Date());
String dateDirPath = localDirPath + dateDir;
File dirFile = new File(dateDirPath);
if(!dirFile.exists()){
dirFile.mkdirs();
}
String uuid = UUID.randomUUID().toString()
.replace("-","");
String fileType = fileName.substring(fileName.lastIndexOf("."));
String newFileName = uuid + fileType;
String path = dateDirPath + newFileName;
file.transferTo(new File(path));
} catch (IOException e) {
e.printStackTrace();
return null;
}
return null;
}
}
2.4 文件删除操作
2.4.1 文件删除JS
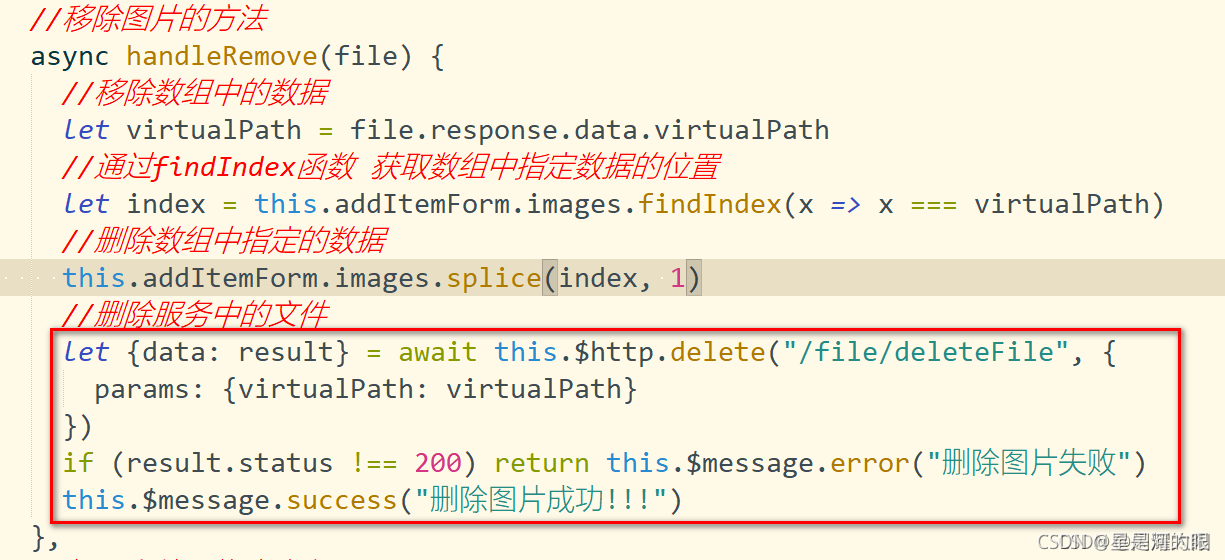
2.4.2 文件删除业务接口
- 请求路径: http://localhost:8091/file/deleteFile
- 请求类型: delete
- 请求参数:
参数名称 |
参数说明 |
备注 |
virtualPath |
文件上传的虚拟的路径 |
删除时需要磁盘路径一起删除 |
参数名称 |
参数说明 |
备注 |
status |
状态信息 |
200表示服务器请求成功 201表示服务器异常 |
msg |
服务器返回的提示信息 |
可以为null |
data |
服务器返回的业务数据 |
可以为null |
2.4.3 编辑FileController
@DeleteMapping("/deleteFile")
public SysResult deleteFile(String virtualPath){
fileService.deleteFile(virtualPath);
return SysResult.success();
}
2.4.4 编辑FileService
@Override
public void deleteFile(String virtualPath) {
String filePath = localDirPath + virtualPath;
File file = new File(filePath);
if(file.exists()){
file.delete();
}
}
2.5 图片路径封装
2.5.1 路径分析
- 图片网络地址: https://img14.360buyimg.com/n0/jfs/t2/ac4a3f32ea776da3.jpg
协议://域名:80/虚拟地址
- 图片地址封装: http://image.jt.com:80/2021/11/11/uuid.jpg.
2.5.2 页面URL地址封装
package com.jt.service;
import com.jt.vo.ImageVO;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.net.FileNameMap;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Service
public class FileServiceImpl implements FileService{
private String localDirPath = "E:/project3/images";
private String preUrl = "http://image.jt.com";
@Override
public ImageVO upload(MultipartFile file) {
String fileName = file.getOriginalFilename();
fileName = fileName.toLowerCase();
if(!fileName.matches("^.+\\.(jpg|png|gif)$")){
return null;
}
try {
BufferedImage bufferedImage = ImageIO.read(file.getInputStream());
int height = bufferedImage.getHeight();
int width = bufferedImage.getWidth();
if(height == 0 || width == 0){
return null;
}
String dateDir = new SimpleDateFormat("/yyyy/MM/dd/")
.format(new Date());
String dateDirPath = localDirPath + dateDir;
File dirFile = new File(dateDirPath);
if(!dirFile.exists()){
dirFile.mkdirs();
}
String uuid = UUID.randomUUID().toString()
.replace("-","");
String fileType = fileName.substring(fileName.lastIndexOf("."));
String newFileName = uuid + fileType;
String path = dateDirPath + newFileName;
file.transferTo(new File(path));
String virtualPath = dateDir + newFileName;
String url = preUrl + virtualPath;
System.out.println(url);
return new ImageVO(virtualPath,url,newFileName);
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
@Override
public void deleteFile(String virtualPath) {
String filePath = localDirPath + virtualPath;
File file = new File(filePath);
if(file.exists()){
file.delete();
}
}
}
2.6 动态为属性赋值
2.6.1 业务需求
说明: 如果将属性写死到java类中,后期维护时 导致维护不方便.
优化: 可以通过@value注解动态赋值.
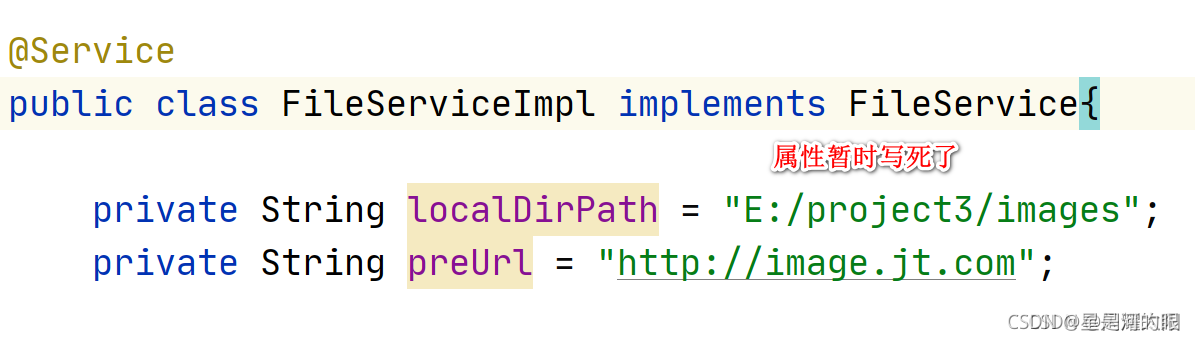
2.6.2 编辑properties配置文件
image.localDirPath=E:/project3/images
image.preUrl=http://image.jt.com
2.6.3 属性动态赋值
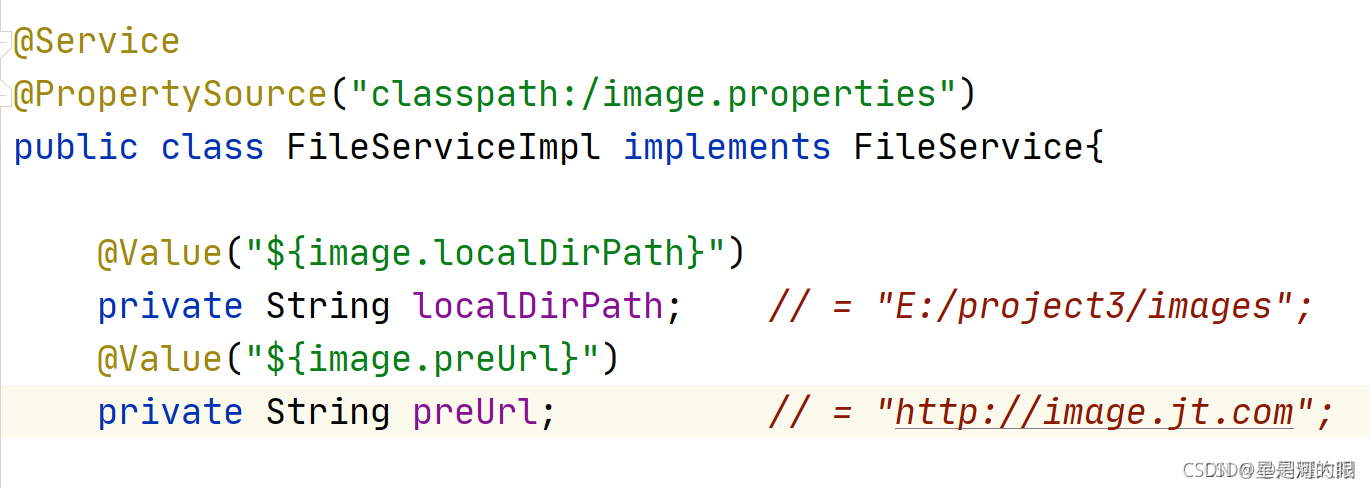