1.压缩工具类
package com.datago.robot.common.utils;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
/**
* @ProjectName powerFind
* @Package com.datago.robot.common.utils
* @Name ZipFileDown
* @Author HB
* @Date 2021/3/18 09:39
* @Version 1.0
*/
public class ZipFileDown {
/**
*
* @param files List<File> 作为参数传进来,就是把多个文件的路径放到一个list里面
* @param response
* @return
* @throws Exception
*/
public static HttpServletResponse downLoadFiles(String zipFilename,List<File> files, HttpServletResponse response) throws Exception {
try {
// 临时文件夹 最好是放在服务器上,方法最后有删除临时文件的步骤
// String zipFilename = "D:/tempFile.zip";
File file = new File(zipFilename);
file.createNewFile();
if (!file.exists()) {
file.createNewFile();
}
response.reset();
// response.getWriter()
// 创建文件输出流
FileOutputStream fous = new FileOutputStream(file);
ZipOutputStream zipOut = new ZipOutputStream(fous);
zipFile(files, zipOut);
zipOut.close();
fous.close();
return downloadZip(file, response);
} catch (Exception e) {
e.printStackTrace();
}
return response;
}
/**
* 把接受的全部文件打成压缩包
*/
public static void zipFile(List files, ZipOutputStream outputStream) {
int size = files.size();
for (int i = 0; i < size; i++) {
File file = (File) files.get(i);
zipFile(file, outputStream);
}
}
/**
* 根据输入的文件与输出流对文件进行打包
*/
public static void zipFile(File inputFile, ZipOutputStream ouputStream) {
try {
if (inputFile.exists()) {
if (inputFile.isFile()) {
FileInputStream IN = new FileInputStream(inputFile);
BufferedInputStream bins = new BufferedInputStream(IN, 1024);
ZipEntry entry = new ZipEntry(inputFile.getName());
ouputStream.putNextEntry(entry);
// 向压缩文件中输出数据
int nNumber;
byte[] buffer = new byte[1024];
while ((nNumber = bins.read(buffer)) != -1) {
ouputStream.write(buffer, 0, nNumber);
}
// 关闭创建的流对象
bins.close();
IN.close();
} else {
try {
File[] files = inputFile.listFiles();
for (int i = 0; i < files.length; i++) {
zipFile(files[i], ouputStream);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static HttpServletResponse downloadZip(File file, HttpServletResponse response) {
if (file.exists() == false) {
System.out.println("待压缩的文件目录:" + file + "不存在.");
} else {
try {
// 以流的形式下载文件。
InputStream fis = new BufferedInputStream(new FileInputStream(file.getPath()));
byte[] buffer = new byte[fis.available()];
fis.read(buffer);
fis.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
return response;
}
}
2.API接口
@GetMapping("/zipFileDown")
@ApiOperation(value = "批量下载表彰文件")
public RestResult<String> zipFileDown(@RequestParam(value = "commendId") String commendId,
HttpServletResponse response) {
try {
File file = new File(commendFile);
if (!file.exists()) {
file.mkdirs();
}
String[] split = commendId.split(",");
List files = new ArrayList();
for (String sp : split
) {
ModelCommendFile cf = commendFileService.findById(sp);
//文件(绝对路径)
String fielUrl = cf.getCommendFileUrl();
if (Utils.isNotEmpty(fielUrl)) {
File filePath = new File(commendFile.split("/")[0] + fielUrl);
files.add(filePath);
}
}
//压缩文件名
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss");
String format = sdf.format(new Date());
String s = format + ".zip";
ZipFileDown.downLoadFiles(commendFile + s, files, response);
String http = httpPath + commendFile.replaceAll(commendFile.split("/")[0], "") + s;
return RestResultUtil.genSuccessResult(http);
} catch (Exception e) {
e.printStackTrace();
return RestResultUtil.failed();
}
}
3.成功页面
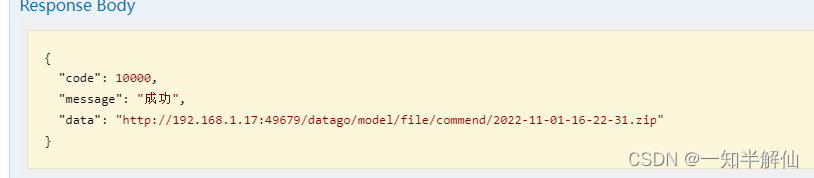