我看了很多网上的demo,先生成ZIP压缩文件,然后再下载。
我这里是生成ZIP文件流 进行下载。(核心代码没多少,就是一些业务代码)
@RequestMapping(value = "/")
public ResponseEntity<byte[]> downloadInterviewFile() throws Exception {
// 根据面试官主键编码 下载文件
List<InterviewFile> interviewFiles = this.interviewFileService.getAll(interviewfile);
ByteArrayOutputStream byteOutPutStream = null;
if (!interviewFiles.isEmpty()) {
//单个文件名称
String interviewFileName = "";
//文件后缀
String fileNameSuffix = "";
//创建一个集合用于 压缩打包的参数
List<Map<String, String>> parms = new ArrayList<>();
//创建一个map集合
Map<String, String> fileMaps =null;
//得到存储文件盘符 例 D:
String root = properties.getValue("upload.root");
//创建一个字节输出流
byteOutPutStream = new ByteArrayOutputStream();
for (InterviewFile file : interviewFiles) {
fileMaps = new HashMap<>();
interviewFileName = file.getFileName();
fileNameSuffix = file.getFileSuffix();
//将单个存储路径放入
fileMaps.put("filePath", root.concat(file.getFilePath()));
//将单个文件名放入
fileMaps.put("fileName", interviewFileName.concat("." + fileNameSuffix));
//放入集合
parms.add(fileMaps);
}
//压缩文件
FileUtils.batchFileToZIP(parms, byteOutPutStream);
}
HttpHeaders headers = new HttpHeaders();
String fileName = null;
try {
fileName = new String("附件.zip".getBytes("UTF-8"), "ISO-8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);// 文件名称
ResponseEntity<byte[]> responseEntity = new ResponseEntity<byte[]>(byteOutPutStream.toByteArray(), headers, HttpStatus.CREATED);
return responseEntity;
}
工具类
@RequestMapping(value = "/")
public ResponseEntity<byte[]> downloadInterviewFile() throws Exception {
// 根据面试官主键编码 下载文件
List<InterviewFile> interviewFiles = this.interviewFileService.getAll(interviewfile);
ByteArrayOutputStream byteOutPutStream = null;
if (!interviewFiles.isEmpty()) {
//单个文件名称
String interviewFileName = "";
//文件后缀
String fileNameSuffix = "";
//创建一个集合用于 压缩打包的参数
List<Map<String, String>> parms = new ArrayList<>();
//创建一个map集合
Map<String, String> fileMaps =null;
//得到存储文件盘符 例 D:
String root = properties.getValue("upload.root");
//创建一个字节输出流
byteOutPutStream = new ByteArrayOutputStream();
for (InterviewFile file : interviewFiles) {
fileMaps = new HashMap<>();
interviewFileName = file.getFileName();
fileNameSuffix = file.getFileSuffix();
//将单个存储路径放入
fileMaps.put("filePath", root.concat(file.getFilePath()));
//将单个文件名放入
fileMaps.put("fileName", interviewFileName.concat("." + fileNameSuffix));
//放入集合
parms.add(fileMaps);
}
//压缩文件
FileUtils.batchFileToZIP(parms, byteOutPutStream);
}
HttpHeaders headers = new HttpHeaders();
String fileName = null;
try {
fileName = new String("附件.zip".getBytes("UTF-8"), "ISO-8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);// 文件名称
ResponseEntity<byte[]> responseEntity = new ResponseEntity<byte[]>(byteOutPutStream.toByteArray(), headers, HttpStatus.CREATED);
return responseEntity;
}
调用看不懂?那来个简单粗暴的
@RequestMapping(value = "/")
public ResponseEntity<byte[]> downloadInterviewFile() throws Exception {
// ---------------------------压缩文件处理-------------------------------
ByteArrayOutputStream byteOutPutStream = new ByteArrayOutputStream();
// 创建一个集合用于 压缩打包的参数
List<Map<String, String>> parms = new ArrayList<>();
// 创建一个map集合
Map<String, String> fileMaps = new HashMap<>();
fileMaps.put("filePath", "D:/文件路径");
fileMaps.put("fileName", "HRM-Package.txt");
Map<String, String> fileMaps1 = new HashMap<>();
fileMaps1.put("filePath", "D:/文件路径1");
fileMaps1.put("fileName", "java.txt");
// 放入集合
parms.add(fileMaps);
parms.add(fileMaps1);
// 压缩文件
FileUtils.batchFileToZIP(parms, byteOutPutStream);
// ---------------------------压缩文件处理-------------------------------
HttpHeaders headers = new HttpHeaders();
String fileName = null;
try {
fileName = new String("附件.zip".getBytes("UTF-8"), "ISO-8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);// 文件名称
ResponseEntity<byte[]> responseEntity = new ResponseEntity<byte[]>(byteOutPutStream.toByteArray(), headers,
HttpStatus.CREATED);
return responseEntity;
}
结果: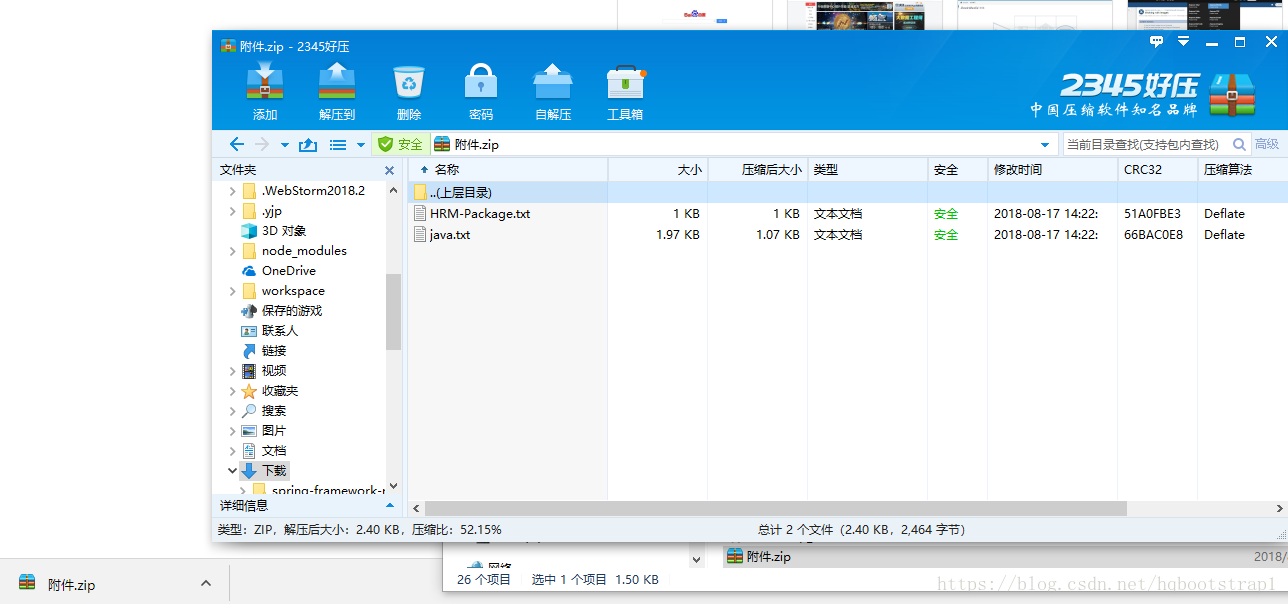
======================我是分割线======================
这个例子给我了我很大的帮助,因为他是利用ResponseEntity进行下载的,这个下载工具类很好,但是网上一般的例子是基于File的而不是基于byte[]数组的,我的文件是存到数据库中的,文件是用byte[]数组存储的,程序猿一定要深刻理解ZipOutputStream在压缩中的处理关键,话不多说,我直接上我改造的代码工具类
注:FileBank类只有两个属性fileName、fileBlob
public class ZipDownloadUtils {
/**
* 文件批量压缩
*
* @param parms
*/
public static void batchFileToZIP(List<FileBank> parms, ByteArrayOutputStream byteOutPutStream) {
ZipOutputStream zipOut = new ZipOutputStream(byteOutPutStream);
try {
for (FileBank value : parms) {
// 使用指定名称创建新的 ZIP 条目 (通俗点就是文件名)
ZipEntry zipEntry = new ZipEntry(value.getFileName());
// 开始写入新的 ZIP 文件条目并将流定位到条目数据的开始处
zipOut.putNextEntry(zipEntry);
//直接写入到压缩输出流中即可
zipOut.write(value.getFileBlob());
zipOut.closeEntry();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
zipOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* ResponseEntity下载文件
*
* @param fileName
* @param byteOutPutStream
*/
public static ResponseEntity<byte[]> downloadZIP(String fileName, ByteArrayOutputStream byteOutPutStream) {
//下载文件
//String fileName = "批量下载【备案材料】.zip";
HttpHeaders headers = new HttpHeaders();
try {
fileName = new String("附件.zip".getBytes("UTF-8"), "ISO-8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", fileName);// 文件名称
ResponseEntity<byte[]> responseEntity = new ResponseEntity<byte[]>(byteOutPutStream.toByteArray(), headers, HttpStatus.CREATED); return responseEntity; } }
Controller中代码很简单:
//压缩文件
ByteArrayOutputStream byteOutPutStream = new ByteArrayOutputStream();
ZipDownloadUtils.batchFileToZIP(dataList, byteOutPutStream); //dataList是一个List<FileBank>
//下载文件
String fileName = "批量下载【备案材料】.zip";
return ZipDownloadUtils.downloadZIP(fileName, byteOutPutStream);