布局相关组件:
文章目录
一、各基础组件的作用及运用
SingleChildRenderObjectWidget(单节点布局组件)
// Padding
设置布局内边距
// Opacity
通常用来改变透明度
// ClipRRect
将布局裁剪成方形
Padding(//填充
padding: EdgeInsets.all(10),//padding设置内边距
child: ClipRRect(//剪裁成方形
//圆角
borderRadius:BorderRadius.all(Radius.circular(10)),
child: Opacity(
opacity: 0.6,//60%的透明度
child: Image.network(
'https://pic.netbian.com/uploads/allimg/220409/000014-1649433614c50f.jpg',
width: 100,
height: 100,
),
),
),
)
// ClipOval
将布局裁剪成圆形
// SizedBox
用来约束布局大小
ClipOval(//剪裁成圆形
child: SizedBox(//设置尺寸
width: 100,
height: 100,
child: Image.network('https://pic.netbian.com/uploads/allimg/220408/005316-16493503969696.jpg'),
),
)
// PhysicalModel
用来将布局显示成不同形状的组件
PhysicalModel(//PhysicalModel ,主要的功能就是设置widget四边圆角,可以设置阴影颜色,和z轴高度
color: Colors.transparent,
borderRadius: BorderRadius.circular(6),
clipBehavior: Clip.antiAlias,//抗锯齿
child: PageView(
children: <Widget>[
_item('page1',Colors.deepPurple),
_item('page2',Colors.green),
_item('page3',Colors.red)
],
),
)
//Align Center
设置居中
alignment: Alignment.center,//alignment设置居中方式
//FractionallySizedBox
用来约束布局水平/垂直方向的伸展、扩张
FractionallySizedBox(//页面布局之百分百布局FractionallySizedBox,
widthFactor: 1,//值为(0.1)
child: Container(
decoration:
BoxDecoration(color: Colors.greenAccent),
child: Text('宽度撑满'),
),
)
MultiChildRenderObjectWidget(多节点布局组件)
//Stack
其作用是堆叠子组件,用来存放多节点布局
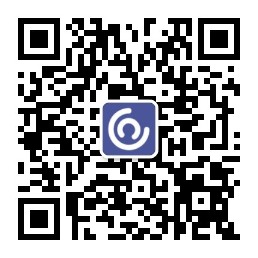
Stack(
// 将两个图片一大一小重叠
children: [
Image.network('https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:100,
height:100,
),
Positioned(
left: 0,
bottom: 0,
child:
Image.network('https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:36,
height:36,
),)
],
)
//Wrap
流式布局组件Wrap
Wrap(
//创建一个Wrap布局,从左向右进行排列,会自动换行
spacing: 8,//水平间距
runSpacing: 6,//垂直间距
children: [
_chip('Flutter'),
_chip('进阶'),
_chip('实战'),
_chip('携程'),
_chip('App'),
],
)
//Flow
流式布局组件Flow
Row和Colum时,如果子widget超出屏幕
范围,则会报溢出错误,这是因为Row默
认只有一行,如果超出屏幕不会折行。我
们把超出屏幕显示范围会自动折行的布局称
为流式布局,flow和wrap都是流式布局组件,
都是通常更多的使用wrap,因为flow使用不
方便
//Flex
Row与Column
Row 组件是不可以滚动的,所以在 Row 组件中一般不会放置过多子组件,如果需要滚动的话应该考虑使用 ListView。
如果需要垂直展示,应该考虑 Column 组件。
这两个组件的属性没有上面区别。
(Expanded 组件的使用很简单,除了一个必传的参数 child 以外,flex 属性,它就相当于 LinearLayout 中的 weight,默认是 1,如果指定为 2 就表示它包裹的子组件占据的空间为总数的两份)
Column //垂直布局
Row //水平方向布局
ParentDataWidget
//Positioned
用于固定view的位置
布局定位控件Positioned,主要用来设置绝对布局,必须配合Stack控件来使用,通过设置left和top属性可以设置控件的绝对位置
Positioned(
left: 0,
bottom: 0,
child:
Image.network('https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:36,
height:36,
),)
//Flexoble Expanded
‘展开’,它可以动态调整child组件沿主轴的尺寸,比如填充剩余空间,比如设置尺寸比例。它常常和Row或Column组合起来使用
Expanded(//自动识别并拉伸container填充可用的空间
child: Container(
decoration: BoxDecoration(
color: Colors.red,
),
child: Text('拉伸填满高度'),
))
二、练习代码
可供参考
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
///如何进行flutter布局开发?
class FlutterLayoutPage extends StatefulWidget {
//const StateFullGroup({Key? key}) : super(key: key);
@override
State<FlutterLayoutPage> createState() => _FlutterLayoutPageState();
}
class _FlutterLayoutPageState extends State<FlutterLayoutPage> {
int _currentIndex = 0;//设置选中状态
@override
Widget build(BuildContext context) {
TextStyle textStyle = TextStyle(fontSize: 20);
return MaterialApp(
title: '如何进行flutter布局开发?',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(title: Text('如何进行flutter布局开发?')
),
bottomNavigationBar: BottomNavigationBar(//底部导航栏
currentIndex: _currentIndex,
onTap: (index){
setState(() {
_currentIndex = index;
});
},
items:[
BottomNavigationBarItem(
icon: Icon(
Icons.home,
color: Colors.grey,
),
activeIcon: Icon(
Icons.home,
color: Colors.blue,
),
title: Text('首页')
),
BottomNavigationBarItem(
icon: Icon(
Icons.list,
color: Colors.grey,
),
activeIcon: Icon(
Icons.list,
color: Colors.blue,
),
title: Text('列表'))
]),
floatingActionButton: FloatingActionButton(//实现悬浮按钮
onPressed: null,
child: Text('点我'),
),
body: _currentIndex==0//实现页面切换
? RefreshIndicator(//RefreshIndicator下拉刷新一定要配合列表使用,所以这里用列表包裹了container
child:
ListView(
children: <Widget>[
Container(
decoration: BoxDecoration(color: Colors.white),
alignment: Alignment.center,//alignment设置居中方式
child: Column(
children:<Widget> [
Row(//水平方向布局
children: <Widget>[
ClipOval(//剪裁成圆形
child: SizedBox(
width: 100,
height: 100,
child: Image.network('https://pic.netbian.com/uploads/allimg/220408/005316-16493503969696.jpg'),
),
),
Padding(
padding: EdgeInsets.all(10),//padding设置内边距
child: ClipRRect(//剪裁成方形
//圆角
borderRadius:BorderRadius.all(Radius.circular(10)),
child: Opacity(
opacity: 0.6,//60%的透明度
child: Image.network(
'https://pic.netbian.com/uploads/allimg/220409/000014-1649433614c50f.jpg',
width: 100,
height: 100,
),
),
),
)
],
),
Image.network(//图片
'https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:100,
height:100,
),
TextField( ///输入框
//输入文本样式
decoration: InputDecoration(
contentPadding: EdgeInsets.fromLTRB(5, 0, 0, 0),//边距
hintText: '请输入',//默认文本
hintStyle: TextStyle(fontSize: 15)
),
),
Container(///pageview滑动页面的用法
height: 100,
margin: EdgeInsets.all(10),
child:
PhysicalModel(color: Colors.transparent,
borderRadius: BorderRadius.circular(6),
clipBehavior: Clip.antiAlias,//抗锯齿
child: PageView(
children: <Widget>[
_item('page1',Colors.deepPurple),
_item('page2',Colors.green),
_item('page3',Colors.red)
],
),
),
),
Column(
children: <Widget>[
FractionallySizedBox(//页面布局之百分百布局FractionallySizedBox,
widthFactor: 1,//值为(0.1)
child: Container(
decoration:
BoxDecoration(color: Colors.greenAccent),
child: Text('宽度撑满'),
),
)
],
),
],
),
),
Stack(//将两个图片一大一小重叠
children: [
Image.network('https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:100,
height:100,
),
Positioned(
left: 0,
bottom: 0,
child:
Image.network('https://pic.netbian.com/uploads/allimg/210317/001935-1615911575642b.jpg',
width:36,
height:36,
),)
],
),
Wrap(
//创建一个Wrap布局,从左向右进行排列,会自动换行
spacing: 8,//水平间距
runSpacing: 6,//垂直间距
children: [
_chip('Flutter'),
_chip('进阶'),
_chip('实战'),
_chip('携程'),
_chip('App'),
],
)
],
),
onRefresh: _handleRefresh)
:Column(
children: [
Text('列表'),
Expanded(//自动识别并拉伸container填充可用的空间
child: Container(
decoration: BoxDecoration(
color: Colors.red,
),
child: Text('拉伸填满高度'),
))
],
),
),
);
}
Future<Null> _handleRefresh() async{
await Future.delayed(Duration(milliseconds: 200));
return null;
}
_item(String title, Color color) {
return Container(
alignment: Alignment.center,//对齐方式
decoration: BoxDecoration(color: color),
child: Text(
title,
style:TextStyle(fontSize: 22,color: Colors.white)),
);
}
_chip(String label) {
return Chip(label: Text(label),
avatar: CircleAvatar(
backgroundColor: Colors.blue.shade900,
child: Text(
label.substring(0,1),
style: TextStyle(fontSize: 10),
),
),
);
}
}