Write a function to find the longest common prefix string amongst an array of strings.
If there is no common prefix, return an empty string ""
.
Example 1:
Input: strs = ["flower","flow","flight"]
Output: "fl"
Example 2:
Input: strs = ["dog","racecar","car"]
Output: ""
Explanation:
There is no common prefix among the input strings.
Constraints:
1 <= strs.length <= 200
0 <= strs[i].length <= 200
strs[i]
consists of only lowercase English letters.
Thought:
- 如果字符串数组为空,则返回空字符串。
- 对字符串数组进行排序,这样数组中的第一个字符串就是字典序最小的字符串,最后一个字符串就是字典序最大的字符串。
- 取出数组中的第一个字符串
head
和最后一个字符串tail
,它们的公共前缀就是整个数组的公共前缀。 - 从头开始比较
head
和tail
的字符,直到遇到第一个不相同的字符为止,这个字符之前的部分就是它们的公共前缀。 - 返回公共前缀。
AC:
/*
* @lc app=leetcode.cn id=14 lang=cpp
*
* [14] 最长公共前缀
*/
// @lc code=start
class Solution {
public:
string longestCommonPrefix(vector<string>& strs) {
if(strs.empty())
return string();
sort(strs.begin(), strs.end());
string head = strs.front(), tail = strs.back();
int i, num = min(head.size(), tail.size());
for(i = 0; i < num && head[i] == tail[i]; i++);
return string(head, 0, i);
}
};
// @lc code=end
另外,官方题解的纵向扫描也很容易想出。
class Solution {
public:
string longestCommonPrefix(vector<string>& strs) {
if (!strs.size()) {
return "";
}
int length = strs[0].size();
int count = strs.size();
for (int i = 0; i < length; ++i) {
char c = strs[0][i];
for (int j = 1; j < count; ++j) {
if (i == strs[j].size() || strs[j][i] != c) {
return strs[0].substr(0, i);
}
}
}
return strs[0];
}
};
Supplements:
std::string
是C++
标准库的一个类,它表示一个字符串。
str.front()
:返回字符串的第一个字符。str.back()
:返回字符串的最后一个字符。str.begin()
:返回指向第一个字符的迭代器。str.end()
:返回指向最后一个字符的迭代器的下一个位置。
它们的用法如下:
扫描二维码关注公众号,回复:
15436099 查看本文章
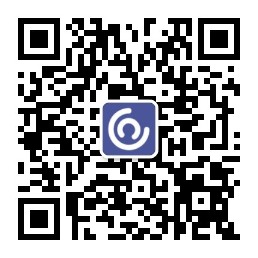
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
// 访问第一个字符
char first = str.front();
std::cout << first << std::endl; // 输出 'H'
// 访问最后一个字符
char last = str.back();
std::cout << last << std::endl; // 输出 '!'
// 使用迭代器遍历字符串
for (auto it = str.begin(); it != str.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl; // 输出 'H e l l o , w o r l d ! '
return 0;
}
需要注意的是,如果字符串为空,则调用str.front()
和str.back()
会导致未定义的行为。另外,str.end()
返回的是迭代器的一个过程值,指向最后一个字符的下一个位置,因此不能解引用。