好的,以下是一个用C语言编写的基本表达式求值问题的代码示例:
``` #include <stdio.h> #include <stdlib.h>
// 定义栈结构体 typedef struct { int *array; // 栈内元素数组指针 int size; // 栈的容量 int top; // 栈顶指针 } Stack;
// 初始化栈 void initStack(Stack s, int size) { s->array = (int) malloc(size * sizeof(int)); s->size = size; s->top = -1; }
// 判断栈是否为空 int isEmpty(Stack *s) { return s->top == -1; }
// 判断栈是否已满 int isFull(Stack *s) { return s->top == s->size - 1; }
// 入栈 void push(Stack *s, int data) { if (isFull(s)) { printf("Error: Stack is full.\n"); return; } s->top++; s->array[s->top] = data; }
// 出栈 int pop(Stack *s) { if (isEmpty(s)) { printf("Error: Stack is empty.\n"); return -1; } int data = s->array[s->top]; s->top--; return data; }
// 获取栈顶元素 int peek(Stack *s) { if (isEmpty(s)) { printf("Error: Stack is empty.\n"); return -1; } return s->array[s->top]; }
// 判断操作符优先级 int precedence(char op) { if (op == '+' || op == '-') { return 1; } else if (op == '*' || op == '/') { return 2; } else { return 0; } }
// 计算表达式 int evaluate(char expression) { Stack operandStack; // 操作数栈 Stack operatorStack; // 操作符栈 int i = 0; while (expression[i] != '\0') { if (expression[i] == ' ') { // 忽略空格 i++; continue; } else if (isdigit(expression[i])) { // 如果是数字,将其入栈 int operand = 0; while (isdigit(expression[i])) { operand = operand * 10 + (expression[i] - '0'); i++; } push(&operandStack, operand); } else { // 如果是操作符,将其入栈 while (!isEmpty(&operatorStack) && precedence(peek(&operatorStack)) >= precedence(expression[i])) { int operand2 = pop(&operandStack); int operand1 = pop(&operandStack); char op = pop(&operatorStack); if (op == '+') { push(&operandStack, operand1 + operand2); } else if (op == '-') { push(&operandStack, operand1 - operand2); } else if (op == '') { push(&operandStack, operand1 * operand2); } else if (op == '/') { push(&operandStack, operand1 / operand2); } } push(&operatorStack, expression[i]); i++; } } while (!isEmpty(&operatorStack)) { int operand2 = pop(&operandStack); int operand1 = pop
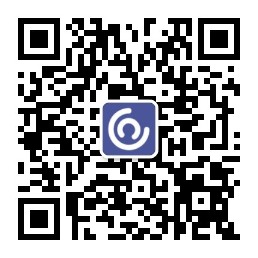