目录
MybatisPlus 是一个功能强大的 MyBatis 增强工具,它提供了丰富的特性来简化操作数据库的代码。它主要用于简化 JDBC 操作,节省开发时间,并能够自动化完成所有的 CRUD 代码。
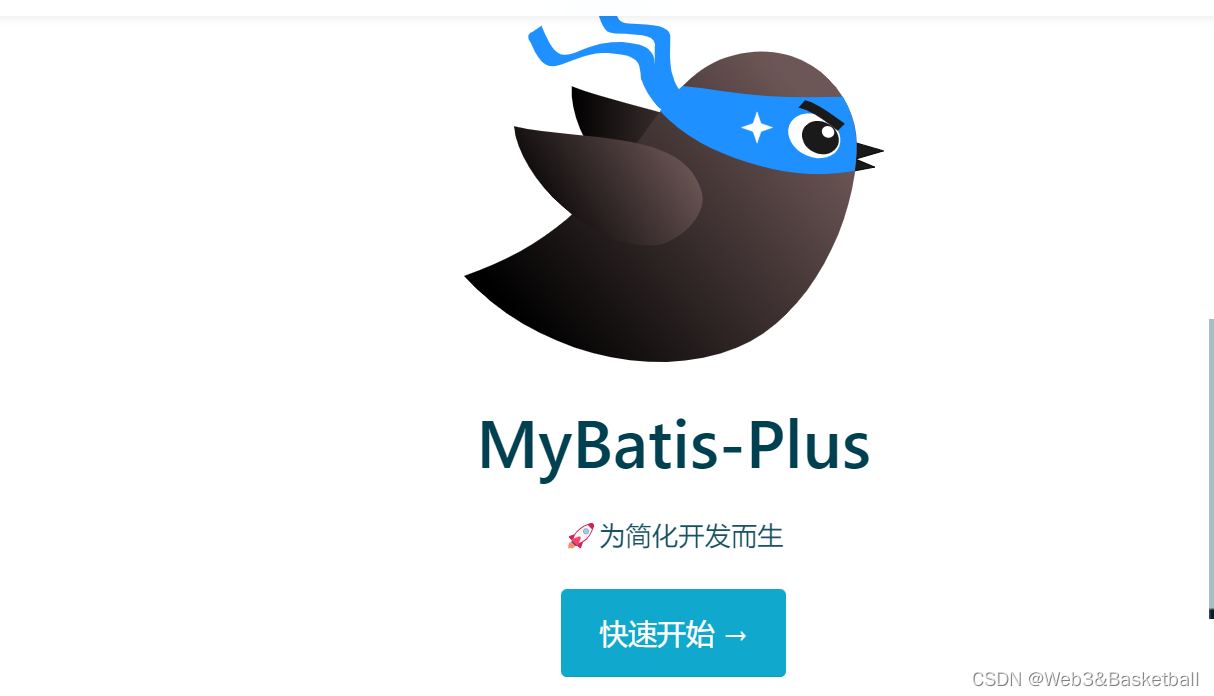
MybatisPlus官网:https://baomidou.com/
1. MybatisPlus 的基本功能
- 提供丰富的 CRUD 方法,包括:insert、selectById、selectBatchIds、update、delete 等。
- 提供乐观锁功能,支持版本号管理。
- 提供查询条件生成器,简化查询条件的编写。
- 提供代码生成器,自动生成实体类、Mapper 接口、Service 类、Controller 类等。
- 支持多种数据库类型,如:MySQL、Oracle、SQL Server 等。
2. 基本用法
(1) 分页插件
MybatisPlus 的分页逻辑底层是通过分页插件来完成的。分页插件的实现原理主要是基于 MyBatis 的动态 SQL 生成,通过 Mybatis 的 count 和 offset 的实现来实现分页功能。
(2) 自动装配
MybatisPlus 提供了自动装配功能,可以自动根据实体类生成对应的 Mapper 接口、Service 类和 Controller 类。自动装配的实现原理是基于 Mybatis 的 XML 配置文件,通过解析 XML 文件生成对应的 Java 代码。
(3) 条件查询
MybatisPlus 提供了丰富的查询方法,如 eq、ne、gt、ge、lt、le、like 等。这些查询方法底层是通过 MyBatis 的动态 SQL 生成来实现的,通过拼接 SQL 语句来实现条件查询。
- eq:等于
用于查询字段值等于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().eq("age", 18));
- ne:不等于
用于查询字段值不等于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().ne("age", 18));
- gt:大于
用于查询字段值大于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().gt("age", 18));
- ge:大于等于
用于查询字段值大于等于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().ge("age", 18));
- lt:小于
用于查询字段值小于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().lt("age", 18));
- le:小于等于
用于查询字段值小于等于指定值的记录。
List<User> users = userMapper.selectList(new QueryWrapper<User>().le("age", 18));
- like:模糊查询
用于根据指定的字符串进行模糊查询。可以指定匹配前缀、后缀或指定多个字符。
// 匹配前缀
List<User> users = userMapper.selectList(new QueryWrapper<User>().like("name", "zhang%"));
// 匹配后缀
List<User> users = userMapper.selectList(new QueryWrapper<User>().like("name", "%zhang"));
// 匹配多个字符
List<User> users = userMapper.selectList(new QueryWrapper<User>().like("name", "z%a%"));
3. MybatisPlus 的配置
在 Spring Boot 项目中,我们可以通过以下步骤来配置 MybatisPlus:
- 在 pom.xml 文件中添加 MybatisPlus 的依赖:
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3.1</version>
</dependency>
- 在 application.properties 文件中配置 MybatisPlus:
mybatis-plus.mapper-locations=classpath:mapper/*.xml
mybatis-plus.type-aliases-package=com.example.demo.entity
mybatis-plus.global-config.id-type=auto
mybatis-plus.global-config.db-config.logic-delete-value=1
mybatis-plus.global-config.db-config.logic-not-delete-value=0
4. MybatisPlus 的实体类、Mapper 接口、Service 类和 Controller 类
- 实体类(Entity):实体类是用于映射数据库表的,通常包含了表的字段以及对应的 getter 和 setter 方法。例如,假设有一个用户表(user),那么对应的实体类可能如下:
package com.example.demo.entity;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
private String email;
// getter 和 setter 方法
}
- Mapper 接口:Mapper 接口用于定义与数据库表相关的操作。例如,对于用户表(user),对应的 Mapper 接口可能如下:
package com.example.demo.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.demo.entity.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
- Service 类:Service 类用于处理业务逻辑。它通常包含了一些与数据库操作相关的方法。例如,对于用户表(user),对应的 Service 类可能如下:
package com.example.demo.service;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.example.demo.entity.User;
import com.example.demo.mapper.UserMapper;
import org.springframework.stereotype.Service;
@Service
public class UserService extends ServiceImpl<UserMapper, User> {
}
- Controller 类:Controller 类用于处理 HTTP 请求。它通常包含了一些与数据库操作相关的方法。例如,对于用户表(user),对应的 Controller 类可能如下:
package com.example.demo.controller;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> getUsers() {
return userService.list();
}
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getById(id);
}
@PostMapping
public void addUser(@RequestBody User user) {
userService.save(user);
}
@PutMapping("/{id}")
public void updateUser(@PathVariable Long id, @RequestBody User user) {
userService.updateById(id, user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userService.removeById(id);
}
}