7. Reverse Integer
Given a 32-bit signed integer, reverse digits of an integer.
Note: Assume we are dealing with an environment which could only store integers within the 32-bit signed integer range: [−23^1, 2^31 − 1]. For the purpose of this problem, assume that your function returns 0 when the reversed integer overflows.
Input: 123 Output: 321
Input: -123 Output: -321
Input: 120 Output: 21
翻转数字 (注意有int范围限制)
方法1:21ms 99.86%
翻转数字 与 翻转字符串操作不同。
!!翻转数字要利用好 %10
与 /10
!!!
通过(newResult - x % 10) / 10 != result)
来判断newResult是否越过int界限,如果上一步计算得到的newResult超出int,不会报错,但是newResult的值并非计算所得的值。
public int reverse2(int x) {
int result = 0;
while (x != 0)
{
int newResult = result * 10 + x % 10;
if ((newResult - x % 10) / 10 != result) //通过这样来判断newResult是否越过int界限,如果上一步计算得到的newResult超出int,不会报错,但是newResult的值并非计算所得的值
return 0;
result = newResult;
x = x / 10;
}
return result;
}
方法2:22ms 不推荐
不推荐,因为它指定了result为long类型,有一定局限性(比如若result超出long怎么办,凉拌),但思路还是可以借鉴的
public int reverse5(int x) {
long result = 0;
while(x != 0)
{
result = (result * 10) + (x % 10);
if(result > Integer.MAX_VALUE) return 0;
if(result < Integer.MIN_VALUE) return 0;
x = x / 10;
}
return (int)result;
}
方法3:23ms
采用字符串StringBuilder的reverse()
方法来翻转
是否越界用异常NumberFormatException
来捕获
java中左侧补0、删0
public int reverse3(int x) {
StringBuilder result = new StringBuilder();
String resultSt = "";
int val = 0;
if (x > 0) {
resultSt = result.append(x).reverse().toString();
try {
val = (int) Integer.parseInt(resultSt); //转换成int的过程中,就自动把数字前面的零去掉了
} catch (NumberFormatException e) { return 0; }
}
else {
x = -x;
resultSt = result.append(x).reverse().toString();
try {
val = -(int) Integer.parseInt(resultSt);
} catch (NumberFormatException e) { return 0; }
}
return val;
}
扫描二维码关注公众号,回复:
2136713 查看本文章
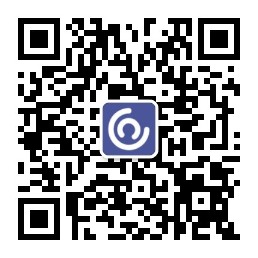