trie:字符串算法中的重要“数据结构”
什么是trie
trie就是利用字符串的公共前缀所建成的树。
众所周知树是有很多很好的性质的,于是trie可以结合其他知识点做一些有趣的事情。
trie的例题
【判断前缀】poj3630Phone List
Description
Given a list of phone numbers, determine if it is consistent in the sense that no number is the prefix of another. Let's say the phone catalogue listed these numbers:
- Emergency 911
- Alice 97 625 999
- Bob 91 12 54 26
In this case, it's not possible to call Bob, because the central would direct your call to the emergency line as soon as you had dialled the first three digits of Bob's phone number. So this list would not be consistent.
Input
The first line of input gives a single integer, 1 ≤ t ≤ 40, the number of test cases. Each test case starts with n, the number of phone numbers, on a separate line, 1 ≤ n ≤ 10000. Then follows n lines with one unique phone number on each line. A phone number is a sequence of at most ten digits.
Output
For each test case, output "YES" if the list is consistent, or "NO" otherwise.
Sample Input
2
3
911
97625999
91125426
5
113
12340
123440
12345
98346
Sample Output
NO
YES
题目分析
这个是trie最基础的应用——判断前缀。
1 #include<cstdio> 2 #include<cctype> 3 #include<cstring> 4 const int maxn = 100035; 5 const int maxe = 13; 6 7 int tt,n,tot; 8 int f[maxn][maxe]; 9 char ch[maxe]; 10 bool vis[maxn],fl; 11 12 int read() 13 { 14 char ch = getchar(); 15 int num = 0; 16 bool fl = 0; 17 for (; !isdigit(ch); ch = getchar()) 18 if (ch=='-') fl = 1; 19 for (; isdigit(ch); ch = getchar()) 20 num = (num<<1)+(num<<3)+ch-48; 21 if (fl) num = -num; 22 return num; 23 } 24 void insert(char *s) 25 { 26 int n = strlen(s), rt = 1; 27 for (int i=0; i<n; i++) 28 { 29 int w = s[i]-'0'; 30 if (!f[rt][w]) f[rt][w] = ++tot; 31 else if (i==n-1) fl = 1; 32 rt = f[rt][w]; 33 if (vis[rt]) fl = 1; 34 } 35 vis[rt] = 1; 36 } 37 int main() 38 { 39 tt = read(); 40 while (tt--) 41 { 42 memset(vis, 0, sizeof vis); 43 memset(f, 0, sizeof f); 44 fl = 0, tot = 1, n = read(); 45 for (int i=1; i<=n; i++) 46 { 47 scanf("%s",ch); 48 insert(ch); 49 } 50 printf("%s\n",!fl?"YES":"NO"); 51 } 52 return 0; 53 }
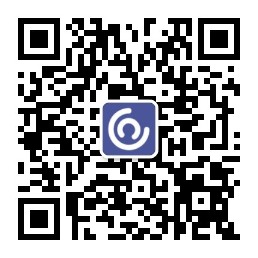
【xor最值】bzoj4260: Codechef REBXOR
Description
Input
Output
Sample Input
1 2 3 1 2
Sample Output
HINT
题目分析
这题就是要稍加建模的题了。
若用dp的思想:$l[i]$表示$1≤l≤r≤i$的最大异或和,$r[i]$表示$i≤l≤r≤n$的最大异或和,那么有$ans=max\{l[i]+r[i+1]\}$。
问题就在于求$l[i],r[i]$,这里讨论$l[i]$的求法,$r[i]$同理。若$r!=i$,那么$l[i]=l[i-1]$;否则就是固定了右端点,再找一个左端点使得$a[x]~a[i]$异或和最大。
粗看xor是没有前缀和加法性质的。但是这不就等于在一个集合里找一个数求其与给定数最大的异或和吗?这就转化成为trie的另一个经典应用了。
1 #include<bits/stdc++.h> 2 const int maxn = 400035; 3 const int maxe = 3; 4 5 int n,tot,cnt,ans; 6 int f[maxn<<5][maxe],a[maxn]; 7 int l[maxn],r[maxn]; 8 9 int read() 10 { 11 char ch = getchar(); 12 int num = 0; 13 bool fl = 0; 14 for (; !isdigit(ch); ch = getchar()) 15 if (ch=='-') fl = 1; 16 for (; isdigit(ch); ch = getchar()) 17 num = (num<<1)+(num<<3)+ch-48; 18 if (fl) num = -num; 19 return num; 20 } 21 void insert(int x) 22 { 23 int u = 1; 24 for (int i=1<<30; i; i>>=1) 25 { 26 int c = (x&i)?1:0; 27 if (!f[u][c]) f[u][c] = ++tot; 28 u = f[u][c]; 29 } 30 } 31 int find(int x) 32 { 33 int u = 1, ret = 0; 34 for (int i=1<<30; i; i>>=1) 35 { 36 int c = (x&i)?0:1; 37 if (f[u][c]) 38 ret += i, u = f[u][c]; 39 else u = f[u][1-c]; //这里u打成c调了半小时…… 40 } 41 return ret; 42 } 43 int main() 44 { 45 n = read(); 46 for (int i=1; i<=n; i++) a[i] = read(); 47 memset(f, 0, sizeof f); 48 tot = 1, cnt = 0, insert(0); 49 for (int i=1; i<=n; i++) 50 { 51 cnt ^= a[i]; 52 insert(cnt); 53 l[i] = std::max(l[i-1], find(cnt)); 54 } 55 memset(f, 0, sizeof f); 56 tot = 1, cnt = 0, insert(0); 57 for (int i=n; i>=1; i--) 58 { 59 cnt ^= a[i]; 60 insert(cnt); 61 r[i] = std::max(r[i+1], find(cnt)); 62 } 63 for (int i=1; i<n; i++) 64 ans = std::max(ans, l[i]+r[i+1]); 65 printf("%d\n",ans); 66 return 0; 67 }
题目分析