一、JSON简介
JSON(JavaScript Object Notation) 是一种轻量级的数据交换格式,它使得人们很容易的进行阅读和编写。同时也方便了机器进行解析和生成。适用于进行数据交互的场景,比如网站前台与后台之间的数据交互。
官方文档:https://docs.python.org/3/library/json.html
JSON在线解析工具:http://www.json.cn/#
1.1、json.loads()
把Json格式字符串解码转换成Python对象,从json到python的类型转化对照如下:
JSON | Python |
---|---|
object | dict |
array | list |
string | str |
number (int) | int |
number (real) | float |
true | True |
false | False |
null | None |
import json strList = '[1, 2, 3, 4]' strDict = '{"city": "北京", "name": "大猫"}' print(json.loads(strList)) print(json.loads(strDict))
1.2、json.dumps()
扫描二维码关注公众号,回复:
2211350 查看本文章
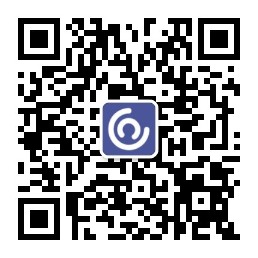
实现python类型转化为json字符串,返回一个str对象把一个Python对象编码转换成Json字符串,从python原始类型向json类型
的转化对照如下:
Python | JSON |
---|---|
dict | object |
list, tuple | array |
str | string |
int, float, int- & float-derived Enums | number |
True | true |
False | false |
None | null |
import json listStr = [1, 2, 3, 4] tupleStr = (1, 2, 3, 4) dictStr = {"city": "北京", "name": "大猫"} print(json.dumps(listStr)) print(json.dumps(tupleStr)) # 注意:json.dumps() 序列化时默认使用的ascii编码 # 添加参数 ensure_ascii=False 禁用ascii编码,按utf-8编码 print(json.dumps(dictStr)) print(json.dumps(dictStr, ensure_ascii=False)) ''' 输出结果: [1, 2, 3, 4] [1, 2, 3, 4] {"city": "\u5317\u4eac", "name": "\u5927\u732b"} {"city": "北京", "name": "大猫"} '''
1.3、json.load()
读取文件中json形式的字符串元素 转化成python类型
import json strList = json.load(open("listStr.json")) print(strList) strDict = json.load(open("dictStr.json")) print(strDict)
1.4、json.dump()
将Python内置类型序列化为json对象后写入文件
import json listStr = [{"city": "北京"}, {"name": "大刘"}] json.dump(listStr, open("listStr.json","w"), ensure_ascii=False) dictStr = {"city": "北京", "name": "大刘"} json.dump(dictStr, open("dictStr.json","w"), ensure_ascii=False)
二、JsonPath简介
JsonPath 是一种信息抽取类库,是从JSON文档中抽取指定信息的工具
官方文档:http://goessner.net/articles/JsonPath
2.1、JsonPath与Xpath语法对比
Json结构清晰,可读性高,复杂度低,非常容易匹配,下表中对应了XPath的用法
XPath | JSONPath | 描述 |
---|---|---|
/ | $ | 根节点 |
. | @ | 现行节点 |
/ | .or[] | 取子节点 |
.. | n/a | 取父节点,Jsonpath未支持 |
// | .. | 就是不管位置,选择所有符合条件的条件 |
* | * | 匹配所有元素节点 |
@ | n/a | 根据属性访问,Json不支持,因为Json是个Key-value递归结构,不需要。 |
[] | [] | 迭代器标示(可以在里边做简单的迭代操作,如数组下标,根据内容选值等) |
[,] | 支持迭代器中做多选。 | |
[] | ?() | 支持过滤操作. |
n/a | () | 支持表达式计算 |
() | n/a | 分组,JsonPath不支持 |
2.2、实例
from urllib import request import jsonpath import json url = 'http://www.lagou.com/lbs/getAllCitySearchLabels.json' req =request.Request(url) response = request.urlopen(req) html = response.read() # 把json格式字符串转换成python对象 jsonobj = json.loads(html) # 从根节点开始,匹配name节点 citylist = jsonpath.jsonpath(jsonobj,'$..name') print(citylist) print(type(citylist)) fp = open('city.json','w') content = json.dumps(citylist, ensure_ascii=False) print(content) fp.write(content.encode("utf-8").decode("utf-8")) fp.close()
三、字符串编码转换
# 1. 因为Python3默认字符串是unicode格式 unicodeStr = "你好地球" print(unicodeStr) # 2. 再将 Unicode 编码格式字符串 转换成 GBK 编码 gbkData = unicodeStr.encode("GBK") print(gbkData) # 1. 再将 GBK 编码格式字符串 转化成 Unicode unicodeStr = gbkData.decode("gbk") print(unicodeStr) # 2. 再将 Unicode 编码格式字符串转换成 UTF-8 utf8Str = unicodeStr.encode("UTF-8") print(utf8Str)