答辩终于顺利结束了,回到公司leader就给了个小需求,说起来挺简单的,不过由于自己各种原因再加自己测试,弄了三天才算完成,哎~
需求功能流程放在文末,在这里主要记录一下其中的功能点:
A>java调用cmd命令;
public static void main(String[] args) {
/*获取cmd命令*/
try {
Process pro = Runtime.getRuntime().exec("cmd /c calc"); //添加要进行的命令,"cmd /c calc"中calc代表要执行打开计算器,如何设置关机请自己查找cmd命令
//cmd /c rd "PATH" /Q /S 删除path下所有东西
//cmd /c move "fromPath" "toPath" 从fromPath移动文件或文件夹到toPath下
BufferedReader br = new BufferedReader(new InputStreamReader(pro.getInputStream())); //虽然cmd命令可以直接输出,但是通过IO流技术可以保证对数据进行一个缓冲。
String msg = null;
while ((msg = br.readLine()) != null) {
System.out.println(msg);
}
} catch (IOException exception) {
}
B>java发送邮件;
参考原文地址:https://blog.csdn.net/qq_32371887/article/details/72821291
JavaMailAPI地址:https://blog.csdn.net/imain/article/details/1453677
使用JavaMail发送邮件
// 1.创建一个程序与邮件服务器会话对象 Session
Properties props = new Properties();
props.setProperty("mail.transport.protocol", "SMTP");
props.setProperty("mail.smtp.host", "smtp.163.com");
props.setProperty("mail.smtp.port", "25");
// 指定验证为true
props.setProperty("mail.smtp.auth", "true");
props.setProperty("mail.smtp.timeout","1000");
// 验证账号及密码,密码需要是第三方授权码
Authenticator auth = new Authenticator() {
public PasswordAuthentication getPasswordAuthentication({
return new PasswordAuthentication("*******@163.com", "*******");
}
};
Session session = Session.getInstance(props, auth);
// 2.创建一个Message,它相当于是邮件内容
Message message = new MimeMessage(session);
// 设置发送者
message.setFrom(new InternetAddress("*******@163.com"));
// 设置发送方式与接收者
message.setRecipient(MimeMessage.RecipientType.TO, new InternetAddress(email));
// 设置主题
message.setSubject("邮件发送测试");
// 设置内容
message.setContent(emailMsg, "text/html;charset=utf-8");
// 3.创建 Transport用于将邮件发送
Transport.send(message);
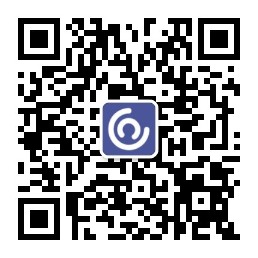
功能大概流程:
1.根据一个时间段 查数据库有哪些hourfolder
select distinct hour_folder FROM FD_DOC_GK_TIFVSPDF where hour_folder between start date and end date
2.遍历hourfolder,根据hourfolder,and PASS_IND='N'的,查询数据库拿到tiff_Path和pdf_Path
select TIF_FILE_PATH,PDF_FILE_PATH FROM FD_DOC_GK_TIFVSPDF WHERE hour_folder=2018060100 AND PASS_IND='N'
3.用dos的move命令将tiff和pdf文件一一移动到一个临时的文件夹里,记录移动次数movecount。
cmd >move TIF_FILE_PATH & PDF_FILE_PATH D:\test\TifToPdfCheck\hour_folder
4.如果tif和pdf文件总个数和movecount相等,则删除原文件夹及文件夹所有东西。
IF TOTAL COUNT = MOVE COUNT
cmd >rd /q /s D:\Case360Migration\Out\DOC_IMG\2018060100
4.1如果删除成功把文件再移动move回来。
MOVE D:\Case360Migration\TifToPdfCheck\hour_folder D:\Case360Migration\Out\DOC_IMG
如果删除失败记录日志
log:Hour folder XXXXX delete failed.
5.如果不相等不做删除,记录总个数和移动次数并发送email
send email >TOTAL COUNT:100 and MOVE COUNT:99 for Hour Folder 2018060100 is different.
6.遍历完所有hourfolder后,
send email;
代码实现:代码写的太不规范,太不美观了,以后得改改了...
DocFolderHouseKeep.java
package com.aia.case360.file.operation;
import java.io.File;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Logger;
import com.aia.case360.constant.Constant;
import com.aia.case360.dao.DBManager;
import com.aia.gmd.iw.util.EmailUtil.MailUtil;
public class DocFolderHouseKeep {
private static Logger logger = Logger.getLogger(DocFolderHouseKeep.class.getName());
private static Process exec = null;
/**
*doQuery select distinct hour_folder from FD_DOC_GK_TIFVSPDF where hour_folder between start date and end date
* @throws InterruptedException
*
*/
public static void main(String[] args) throws IOException, InterruptedException {
try {
if(args.length != 2){
logger.error("illegal input parameter");
return;
}
String startDate = args[0];
String endDate = args[1];
boolean isAllSuccess = true;
List<String> hourfolder = new ArrayList<String>();
String queryFolderSql = new String(Constant.SQL_QUERY_HOURFOLDER);
String qfSql = queryFolderSql.replaceAll("@startDate", startDate).replaceAll("@endDate", endDate);
logger.info("queryFolderSql:" + queryFolderSql);
ResultSet resultSet = DBManager.getC360Helper().excute(qfSql, null);
while (resultSet.next()) {
hourfolder.add(resultSet.getString(1));
logger.info(resultSet.getString(1));
}
FileHouseKeepUtil we = new FileHouseKeepUtil();
// get hour_folder list then to do loop
int count = 0;
StringBuilder sb = new StringBuilder();
Runtime runtime = Runtime.getRuntime();
if(hourfolder.size()==0){
sb.append(" hourFolder count=0 between "+startDate +" and "+ endDate +"; ").append("\r\n");
logger.info(" hourFolder count=0 between "+startDate +" and "+ endDate);
}else{
for(String toPath: hourfolder){
if(!FileHouseKeepUtil.isDirExists(Constant.FOLDER_DOC_IMG + File.separator + toPath)){
sb.append("there no hourfolder:" + Constant.FOLDER_DOC_IMG + File.separator + toPath +"; ").append("\r\n");
logger.info("there no hourfolder:" + Constant.FOLDER_DOC_IMG + File.separator + toPath);
isAllSuccess = false;
continue;
}
String sql = new String(Constant.SQL_QUERY_TIFANDPDFPATH);
String hfsql = sql.replaceAll("@hourfolder", toPath);
ResultSet rs = DBManager.getC360Helper().excute(hfsql, null);
List<String> TIFlist = new ArrayList<String>();
List<String> PDFlist = new ArrayList<String>();
while(rs.next()){
String str1 = new String(rs.getString(1));
String str2 = new String(rs.getString(2));
TIFlist.add(str1.replaceAll("Case360Migration", "Case360_SIT"));
PDFlist.add(str2.replaceAll("Case360Migration", "Case360_SIT"));
}
count = TIFlist.size();
logger.info("count: --------------------"+count);
if(count == 0){
exec = runtime.exec("cmd /c rd "+Constant.FOLDER_DOC_IMG + File.separator + toPath+" /Q /S");
logger.info("PASS_NID count = 0,delete :" + Constant.FOLDER_DOC_IMG + File.separator + toPath);
continue;
}
FileHouseKeepUtil.judeDirExists(Constant.FOLDER_TIFTOPDFCHECK+ toPath);
logger.info("82 line : "+Constant.FOLDER_TIFTOPDFCHECK+ toPath);
int moveCount = 0;
for (int i = 0; i < count; i++) {
int index = TIFlist.get(i).lastIndexOf("\\");
System.out.println("index="+index);
int endindex = TIFlist.get(i).lastIndexOf("\\", index-1);
System.out.println("endindex="+endindex);
String str = TIFlist.get(i).substring(endindex,index);
logger.info("86 line : "+str);
//File file= new File(TIFlist.get(i));
//String filename = file.getName();
StringBuilder tifpath = new StringBuilder(TIFlist.get(i));
tifpath.insert(index+1, "\"");
tifpath.append("\"");
StringBuilder pdfpath = new StringBuilder(PDFlist.get(i));
pdfpath.insert(PDFlist.get(i).lastIndexOf("\\")+1, "\"");
pdfpath.append("\"");
if(FileHouseKeepUtil.isFileExists(TIFlist.get(i)) && FileHouseKeepUtil.judeDirExists(Constant.FOLDER_TIFTOPDFCHECK+ toPath+str)){
exec = runtime.exec("cmd /c move "+ tifpath +" "+ Constant.FOLDER_TIFTOPDFCHECK+ toPath+str);
logger.info("move "+TIFlist.get(i) +" "+ Constant.FOLDER_TIFTOPDFCHECK+ toPath+str);
exec.waitFor();
moveCount ++;
exec = runtime.exec("cmd /c move "+ pdfpath +" "+ Constant.FOLDER_TIFTOPDFCHECK+ toPath+str);
logger.info("move "+PDFlist.get(i) +" "+ Constant.FOLDER_TIFTOPDFCHECK+ toPath+str);
exec.waitFor();
moveCount ++;
logger.info("copyFile: " +TIFlist.get(i)+"AND"+PDFlist.get(i) +"to"+ Constant.FOLDER_TIFTOPDFCHECK+ toPath +str+" success !");
}else{
continue;
}
}
logger.info("moveCount: --------------------"+moveCount);
int tifcount = 0;
int pdfcount = 0;
String tifsql = new String(Constant.SQL_QUERY_TIFCOUNT);
String tifsql1 = tifsql.replaceAll("@hourfolder", toPath);
ResultSet tifrs = DBManager.getC360Helper().excute(tifsql1, null);
while(tifrs.next()){
logger.info("tifrs.getInt(1): --------------------"+tifrs.getInt(1));
tifcount = tifrs.getInt(1);
}
String pdfsql = new String(Constant.SQL_QUERY_PDFCOUNT);
String pdfsql1 = pdfsql.replaceAll("@hourfolder", toPath);
ResultSet pdfrs = DBManager.getC360Helper().excute(pdfsql1, null);
while(pdfrs.next()){
logger.info("pdfrs.getInt(1): --------------------"+pdfrs.getInt(1));
pdfcount = pdfrs.getInt(1);
}
int realcount = tifcount+pdfcount;
logger.info("realcount = " + realcount);
if(realcount == moveCount){
//delete all the file
if(FileHouseKeepUtil.isDirExists(Constant.FOLDER_DOC_IMG + File.separator + toPath)){
exec = runtime.exec("cmd /c rd "+ Constant.FOLDER_DOC_IMG + File.separator + toPath +" /Q /S");
exec.waitFor();
logger.info("rd "+ Constant.FOLDER_DOC_IMG + File.separator + toPath +" /Q /S");
}
//move back to the DOC_IMG folder
exec = runtime.exec("cmd /c move "+Constant.FOLDER_TIFTOPDFCHECK+ toPath +" "+ Constant.FOLDER_DOC_IMG + File.separator + toPath);
exec.waitFor();
logger.info("move "+Constant.FOLDER_TIFTOPDFCHECK+ toPath +" "+ Constant.FOLDER_DOC_IMG + File.separator + toPath);
// sb.append(" TOTAL COUNT:"+realcount +" and MOVE COUNT: "+moveCount +" for Hour Folder "+ toPath +"; ").append("\r\n");
logger.info(" TOTAL COUNT:"+realcount +" and MOVE COUNT: "+moveCount +" for Hour Folder "+ toPath +"; ");
} else {
sb.append("count <> moveCount, TOTAL COUNT:"+realcount +" and MOVE COUNT: "+moveCount +" for Hour Folder "+ toPath +"; ").append("\r\n");
logger.info("count <> moveCount and send warning email ");
if(isAllSuccess){
isAllSuccess = false;
}
}
}
}
// send email
if(isAllSuccess){
sb.append("HouseKeeping completed successfully! \r\n");
MailUtil.sendEmail("Housekeeping ", sb.toString(), null, 0);
} else {
MailUtil.sendEmail("Housekeeping ", sb.toString(), null, 1);
}
} catch (SQLException e) {
e.printStackTrace();
logger.error("Housekeeping error", e);
MailUtil.sendEmail("Housekeeping", e.getMessage(), null, 2);
}
}
}
FileHouseKeepUtil.java
package com.aia.case360.file.operation;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.log4j.Logger;
import com.aia.gmd.iw.util.FileUtil;
public class FileHouseKeepUtil {
private static Logger logger = Logger.getLogger(FileHouseKeepUtil.class.getName());
public boolean copyfile(String fromPath,String toPath){
try {
File file = new File(fromPath);
String absolutePath = file.getAbsolutePath();
int index = absolutePath.lastIndexOf("\\");
String filename = absolutePath.substring(index);
FileUtil.copyFile(file.getParentFile().getAbsolutePath(), filename, toPath, null, false);
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
public static void judeFileExists(File file) {
if (file.exists()) {
System.out.println("file exists");
} else {
System.out.println("file not exists, create it ...");
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static boolean judeDirExists(String path) {
File file = new File(path);
if (file.exists()) {
if (file.isDirectory()) {
System.out.println("dir exists");
return true;
} else {
System.out.println("the same name file exists, can not create dir");
}
} else {
System.out.println("dir not exists, create it ...");
file.mkdirs();
return true;
}
return false;
}
public static boolean isFileExists(String path) {
File file = new File(path);
if (file.exists()) {
return true;
} else {
logger.info("file not exists");
return false;
}
}
public static boolean isDirExists(String path) {
File file = new File(path);
if (file.exists() && file.isDirectory()) {
System.out.println("dir exists");
return true;
}
return false;
}
public static void copyFile(File sourceFile,File targetFile){
if(!sourceFile.canRead()){
System.out.println("Source files" + sourceFile.getAbsolutePath() + " are not readable");
return;
}else{
System.out.println("Start copying files " + sourceFile.getAbsolutePath() + "to " + targetFile.getAbsolutePath());
FileInputStream fis = null;
BufferedInputStream bis = null;
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try{
fis = new FileInputStream(sourceFile);
bis = new BufferedInputStream(fis);
fos = new FileOutputStream(targetFile);
bos = new BufferedOutputStream(fos);
int len = 0;
while((len = bis.read()) != -1){
bos.write(len);
}
bos.flush();
}catch(FileNotFoundException e){
e.printStackTrace();
}catch(IOException e){
e.printStackTrace();
}finally{
try{
if(fis != null){
fis.close();
}
if(bis != null){
bis.close();
}
if(fos != null){
fos.close();
}
if(bos != null){
bos.close();
}
System.out.println("file " + sourceFile.getAbsolutePath() + "copy to " + targetFile.getAbsolutePath() + "success ");
}catch(IOException e){
e.printStackTrace();
}
}
}
}
/**
* copy files in from folder to to folder
* @param sourcePathString from folder
* @param targetPathString to folder
* @return
*/
public boolean copyDirectory(String sourcePathString,String targetPathString){
if(!new File(sourcePathString).canRead()){
System.out.println("Source folder " + sourcePathString + "is unreadable and cannot be copied");
return false;
} else {
File file = new File(targetPathString);
if(!file.exists()){
file.mkdirs();
}
System.out.println("begin copy folder" + sourcePathString + "to " + targetPathString);
File[] files = new File(sourcePathString).listFiles();
for(int i = 0; i < files.length; i++){
if(files[i].isFile()){
copyFile(files[i], new File(targetPathString + File.separator + files[i].getName()));
}else if(files[i].isDirectory()){
copyDirectory(files[i].getAbsolutePath(), targetPathString + File.separator + files[i].getName());
}
}
System.out.println("copy folder" + sourcePathString + "to " + targetPathString + " end");
return true;
}
}
public static boolean delFolder(String folderPath) {
try {
delAllFile(folderPath); // Delete all the contents
String filePath = folderPath;
filePath = filePath.toString();
java.io.File myFilePath = new java.io.File(filePath);
myFilePath.delete(); // delete empty folder
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
// Delete all files under the specified folder
// param path, Complete and absolute path of folders
public static boolean delAllFile(String path) {
boolean flag = false;
File file = new File(path);
if (!file.exists()) {
return flag;
}
if (!file.isDirectory()) {
return flag;
}
String[] tempList = file.list();
File temp = null;
for (int i = 0; i < tempList.length; i++) {
if (path.endsWith(File.separator)) {
temp = new File(path + tempList[i]);
} else {
temp = new File(path + File.separator + tempList[i]);
}
if (temp.isFile()) {
temp.delete();
}
if (temp.isDirectory()) {
delAllFile(path + "/" + tempList[i]);// Delete the files in the folder first
delFolder(path + "/" + tempList[i]);// Deleting empty folders
flag = true;
System.out.println("delete folder:"+path + "/" + tempList[i]+"delete file:"+path + "/" + tempList[i]);
}
}
return flag;
}
}